Write a c++ program that includes two functions for sorting integers. your program must acts as following: First asks user how many integers you wants to be sorted, then reads integers and then by calling the first function sort them in ascending order and by calling the second function sort them in descending order. Sample output: How many integers you wants to sort? 5 Please enter 5 integers: 5 8 -1 0 2 the ascending order of your list is: -1 0 2 5 8 The Descending order of your list is: 8 5 2 0 -1 Note: to do the above question do not use the array.

// As per the requirement , i am not used the array to implements sorting stuff :-
source code :-
#include <iostream>
#include<string>
using namespace std;
void ascendingSort(string &str){
for(int j =0 ; j < str.length() ; j++){
for(int k = 0; k < str.length() - 1 ; k++){
if(str[k] > str[k+1]){
int temp = str[k];
str[k] = str[k+1];
str[k+1] = temp;
}
}
}
}
void descendingSort(string &str){
for(int j =0 ; j < str.length() ; j++){
for(int k = 0; k < str.length() - 1 ; k++){
if(str[k] < str[k+1]){
int temp = str[k];
str[k] = str[k+1];
str[k+1] = temp;
}
}
}
}
void printValue(string str){
for(int i = 0 ; i < str.length() ; i++){
cout<<str[i]<<" ";
}
cout<<"\n";
}
int main()
{
int num ;
string s;
string str = "";
cout<<"How many integers we want to sort : "<<endl;
cin>>num;
int i = 1;
cin.ignore();
while(i <= num){
cout<<"Enter the "<<i<<"th ingeger's : "<<endl;
getline(cin, s);
str += s;
i += 1;
}
// for sorting in ascending order :
cout<<"\nprinting ascending order of your List : "<<endl;
ascendingSort(str);
printValue(str);
// for sorting in descending order :
cout<<"\nprinting descending order of your List : "<<endl;
descendingSort(str);
printValue(str);
return 0;
}
Step by step
Solved in 2 steps with 4 images

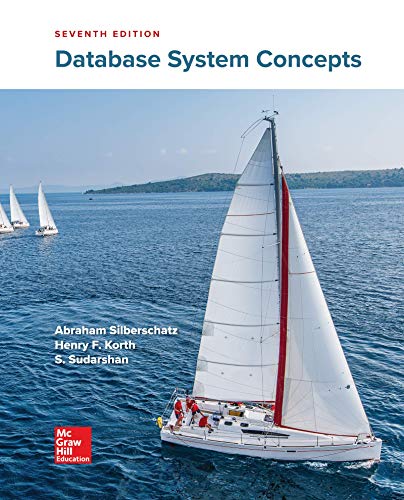
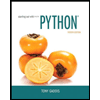
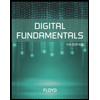
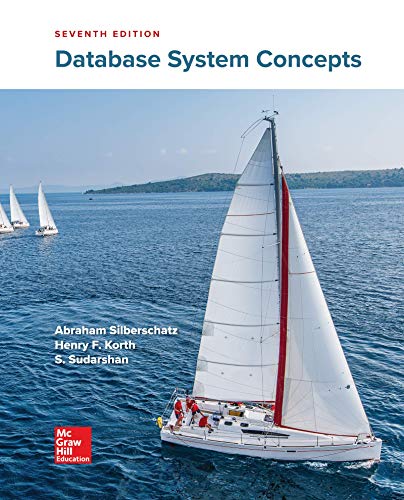
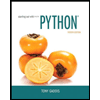
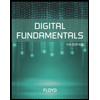
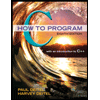
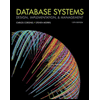
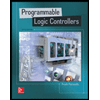