Whenever folks on Earth get into trouble they look towards the Superheroes Federation to save them. Design and implement a Python class called Hero that includes the following class variable(s), instance variable(s) and method(s) for the class. Class variable(s): • TotalNumHeroes is the total number of heroes listed with the Federation • TotalNumChallengesMet is the total number of challenges that all the federation members have successfully overcome Attribute(s)/instance variable(s): • herolD is the unique ID number for the superhero (default value "N/A") • ifeName is the real-life name of the superhero (e.g. Bruce Wayne) (default value "N/A") • alterEgo is the secondary self or secret identity of the superhero (eg. Batman) (default value "N/A") • firstAppeared is the year this superhero was introduced first (e.g. 1939) (default value 0) • knownFor is the special feature for which the hero is known (e.g. can lift unusually heavy objects) (default value "N/A") • toolOfStrength is a tool or weapon that is used by the superhero (eg. hammer) (default value "N/A") • numofChallengesMet is a number that indicates how many challenges have been faced and overcome by the superhero (default value 0) The class definition should also include the following method(s): • a constructor/initializer method, including default values • accessor methods to access and return the instance variables heroiD, alterEgo, and numofChallengesMet a mutator method to update (i.e., add an amount to) the instance variable numofChallengesMet the special method_str_() to print the required information, including the herolD, lifeName, alterEgo, firstAppeared, knownFor, toolOfStrength and numofChallengesMet with appropriate descriptive labels as shown in the sample output below
Whenever folks on Earth get into trouble they look towards the Superheroes Federation to save them. Design and implement a Python class called Hero that includes the following class variable(s), instance variable(s) and method(s) for the class. Class variable(s): • TotalNumHeroes is the total number of heroes listed with the Federation • TotalNumChallengesMet is the total number of challenges that all the federation members have successfully overcome Attribute(s)/instance variable(s): • herolD is the unique ID number for the superhero (default value "N/A") • ifeName is the real-life name of the superhero (e.g. Bruce Wayne) (default value "N/A") • alterEgo is the secondary self or secret identity of the superhero (eg. Batman) (default value "N/A") • firstAppeared is the year this superhero was introduced first (e.g. 1939) (default value 0) • knownFor is the special feature for which the hero is known (e.g. can lift unusually heavy objects) (default value "N/A") • toolOfStrength is a tool or weapon that is used by the superhero (eg. hammer) (default value "N/A") • numofChallengesMet is a number that indicates how many challenges have been faced and overcome by the superhero (default value 0) The class definition should also include the following method(s): • a constructor/initializer method, including default values • accessor methods to access and return the instance variables heroiD, alterEgo, and numofChallengesMet a mutator method to update (i.e., add an amount to) the instance variable numofChallengesMet the special method_str_() to print the required information, including the herolD, lifeName, alterEgo, firstAppeared, knownFor, toolOfStrength and numofChallengesMet with appropriate descriptive labels as shown in the sample output below
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Superhero ID: 6424
Real Life Name: Peter Parker
Number of Challenges Met: 25
Alter Ego: Spider-Man
First Appeared: 1962
Known For: spider related abilities
Tool of Strength: N/A
Superhero ID: 2234
Real Life Name: I'Challa
Alter Ego: Black Panther
First Appeared: 1966
Number of Challenges Met: 50
Day Job: freelance photographer
Mutated By: radioactive spider bite
Known For: enhanced abilities
Tool of Strength: Wakandan technology
Number of Challenges Met: 65
Superhero ID: 7777
Real Life Name: Rex Mason
Alter Ego: Metamorpho
First Appeared: 1965
Known For: can shapeshift into any element
Tool of Strength: N/A
Number of Challenges Met: 30
Day Job: mercenary
Mutated By: radioactivity of an ancient meteor
Superhero ID: 3334
Real Life Name: Diana of Themyscira
Alter Ego: Wonder Woman
First Appeared: 1941
Known For: superhuman powers
Tool of Strength: las30 of truth
Number of Challenges Met: 30
Superhero with most experience meeting challenges: Black Panther
Total number of heroes in the Federation: 8
Total number of challenges completed: 341
Superhero ID: 5545
Real Life Name: Bruce Banner
Alter Ego: Hulk
First Appeared: 1962
Known For: green rage machine
Tool of Strength: N/A
Number of Challenges Met: 45
Day Job: scientist
Mutated By: gamma rays
Question 3- (this question MAY appear on the assignment quiz)
A local taxi company has decided to expand and not only have taxis that go on land (i.e., cars), but also
taxis that go on water (i.e., boats). You have been asked to design a number of classes to represent the
various taxi fares (trips with passengers) and their various fee structures, by using inheritance in
Python.
Superhero ID: 4434
Real Life Name: Steve Rogers
Alter Ego: Captain America
First Appeared: 1941
Known For: enhanced to the peak of human perfection
Tool of Strength: N/A
Number of Challenges Met: 56
Day Job: artist
Mutated By: super-soldier serum
Please read all parts of this question below before starting the question. Include the completed code for all
parts of this question in a file called taxi_company.py.
NOTE: To implement encapsulation, all of the instance variables/fields should be considered private.

Transcribed Image Text:Question 2 (b)
Extend the design of the class in Question 2 (a) to include a subclass, called Mutatediero, that
creates an object with the following additional characteristic(s)/instance variable(s):
dayJob the job that the mutated hero holds in real life (e.g., reporter) (default value "N/A")
• mutatedBy is the element that affected them so that they can mutate (e.g., gamma rays) (default
value "N/A")
Please read all parts of this question before starting the question. Include the completed code for all parts of
this question in a file called superheroes.py.
NOTE: To implement encapsulation, all of the instance variables/fields should be considered private.
Question 2 (a)
Whenever folks on Earth get into trouble they look towards the Superheroes Federation to save them.
and the following method(s):
Design and implement a Python class called Hero that includes the following class variable(s), instance
variable(s) and method(s) for the class.
_str_) method to print all the required information from the Hero class and also the two
attributes listed above (dayJob and mutatedBy)
Class variable(s):
• TotalNumHeroes is the total number of heroes listed with the Federation
• TotalNumChallengesMet is the total number of challenges that all the federation members have
successfully overcome
Question 2 (c)
Test the defined class by performing the following operations in a main function:
• create a list containing 4 Hero objects and 4 mutated hero objects (some sample data given below)
• print each of the list objects using the special_str_ () method on each object (see output
below)
• find the hero or mutated hero with the highest number of challenges met and print their alter ego
print the total number of heroes/mutated heroes created, using the class variable
• print the total number of challenges successfully met by all the Federation members, using the
Attribute(s)/instance variable(s):
• herolD is the unique ID number for the superhero (default value "N/A")
lifeName is the real-life name of the superhero (e.g. Bruce Wayne) (default value "N/A")
• alterEgo is the secondary self or secret identity of the superhero (eg. Batman) (default value
"N/A")
• firstAppeared is the year this superhero was introduced first (e.g. 1939) (default value 0)
• knownFor is the special feature for which the hero is known (e.g. can lift unusually heavy objects)
(default value "N/A")
• toolOfStrength is a tool or weapon that is used by the superhero (e.g. hammer) (default value
"N/A")
• numofChallengesMet is a number that indicates how many challenges have been faced and
class variable
Hint: This main function can be done using one loop and one if statement.
Sample data/output:
Members of the Superheroes Federation
Superhero ID: 1234
Real Life Name: Bruce Wayne
Alter Ego: Batman
First Appeared: 1939
Known For: fighting skills
Tool of Strength: bat-related vehicle
Number of Challenges Met: 40
overcome by the superhero (default value 0)
The class definition should also include the following method(s):
a constructor/initializer method, including default values
• accessor methods to access and return the instance variables herolD, alterEgo, and
numofChallengesMet
a mutator method to update (i.e., add an amount to) the instance variable numofChallengesMet
• the special method_str_0 to print the required information, including the herolD, lifeName,
alterEgo, firstAppeared, knownFor, toolOfStrength and numofChallengesMet with appropriate
Superhero ID: 1543
Real Life Name: Hal Jordan
Alter Ego: Green Lantern
First Appeared: 1940
Known For: able to craft anything he can envision out of sheer
willpower
Tool of Strength: power ring
Number of Challenges Met: 25
descriptive labels as shown in the sample output below
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
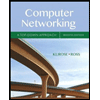
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
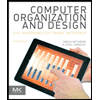
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
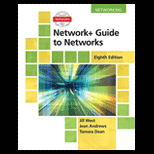
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
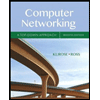
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
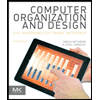
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
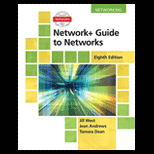
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
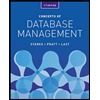
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
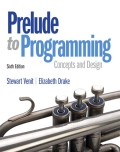
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
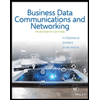
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY