What is the output of the unit testing? #include using namespace std; class TempConvert { public: void SetTemp(int tempVal) { temp = tempVal; } int GetTemp() const { return temp; } TempConvert() { temp = 1; } int InFahrenheit() { return (temp * 1.8) - 32; } private: int temp; }; int main() { TempConvert testData; cout << "Beginning tests." << endl; if (testData.GetTemp() != 0) { cout << " FAILED initialise/get temp" << endl; } testData.SetTemp(10); if (testData.GetTemp() != 10) { cout << " FAILED set/get temp" << endl; } testData.SetTemp(10); if (testData.InFahrenheit() != 50) { cout << " FAILED InFahrenheit for 10 degrees" << endl; } cout << "Tests complete." << endl; return 0; } a. Beginning tests. FAILED initialise/get temp FAILED InFahrenheit for 10 degrees Tests complete. b. Beginning tests. Tests complete. c. Beginning tests. FAILED initialise/get temp FAILED set/get temp FAILED InFahrenheit for 10 degrees Tests complete. d. Beginning tests. FAILED InFahrenheit for 10 degrees Tests complete.
What is the output of the unit testing?
#include <iostream>
using namespace std;
class TempConvert {
public:
void SetTemp(int tempVal) {
temp = tempVal;
}
int GetTemp() const {
return temp;
}
TempConvert() {
temp = 1;
}
int InFahrenheit() {
return (temp * 1.8) - 32;
}
private:
int temp;
};
int main() {
TempConvert testData;
cout << "Beginning tests." << endl;
if (testData.GetTemp() != 0) {
cout << " FAILED initialise/get temp" << endl;
}
testData.SetTemp(10);
if (testData.GetTemp() != 10) {
cout << " FAILED set/get temp" << endl;
}
testData.SetTemp(10);
if (testData.InFahrenheit() != 50) {
cout << " FAILED InFahrenheit for 10 degrees" << endl;
}
cout << "Tests complete." << endl;
return 0;
}
a. |
Beginning tests. |
|
b. |
Beginning tests. |
|
c. |
Beginning tests. |
|
d. |
Beginning tests. |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

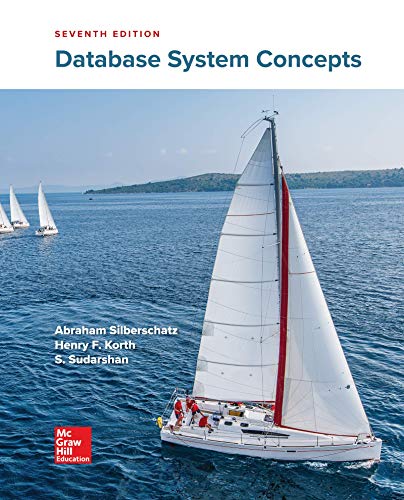
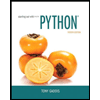
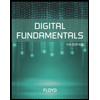
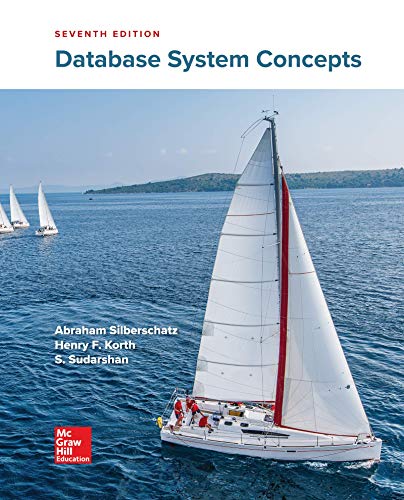
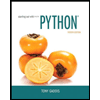
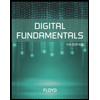
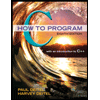
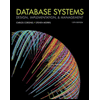
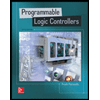