Implement in C Programming 8.16.1: LAB: Time difference #include #include typedef struct Time_struct { int hours; int minutes; int seconds; } Time; // Allocate and return a Time as per parameters Time* CreateTime(int hours, int minutes, int seconds) { Time* newTime = NULL; /* Type your code here. */ } // Read integers hours, minutes, seconds; // create and return a Time using the input values Time* ReadTime() { int hours; int minutes; int seconds; scanf("%d %d %d", &hours, &minutes, &seconds); return CreateTime(hours, minutes, seconds); } Time* TimeDifference(Time* end, Time* start) { Time* diff = NULL; /* TODO: allocate a new Time here */ // Begin with uncorrected arithmetic diff->hours = end->hours - start->hours; diff->minutes = end->minutes - start->minutes; diff->seconds = end->seconds - start->seconds; /* Type your code here. */ } void PrintTime(Time* t) { printf("%02d:%02d:%02d", t->hours, t->minutes, t->seconds); } int main(void) { Time* start = NULL; Time* end = NULL; Time* difference = NULL; start = ReadTime(); end = ReadTime(); difference = TimeDifference(end, start); printf("Start:\t"); PrintTime(start); printf("\nEnd:\t"); PrintTime(end); printf("\nDifference: "); PrintTime(difference); printf("\n"); // Always free dynamically allocated memory when no longer needed free(start); free(end); free(difference); return 0; }
Implement in C Programming
8.16.1: LAB: Time difference
#include <stdio.h>
#include <stdlib.h>
typedef struct Time_struct {
int hours;
int minutes;
int seconds;
} Time;
// Allocate and return a Time as per parameters
Time* CreateTime(int hours, int minutes, int seconds) {
Time* newTime = NULL;
/* Type your code here. */
}
// Read integers hours, minutes, seconds;
// create and return a Time using the input values
Time* ReadTime() {
int hours;
int minutes;
int seconds;
scanf("%d %d %d", &hours, &minutes, &seconds);
return CreateTime(hours, minutes, seconds);
}
Time* TimeDifference(Time* end, Time* start) {
Time* diff = NULL;
/* TODO: allocate a new Time here */
// Begin with uncorrected arithmetic
diff->hours = end->hours - start->hours;
diff->minutes = end->minutes - start->minutes;
diff->seconds = end->seconds - start->seconds;
/* Type your code here. */
}
void PrintTime(Time* t) {
printf("%02d:%02d:%02d", t->hours, t->minutes, t->seconds);
}
int main(void) {
Time* start = NULL;
Time* end = NULL;
Time* difference = NULL;
start = ReadTime();
end = ReadTime();
difference = TimeDifference(end, start);
printf("Start:\t");
PrintTime(start);
printf("\nEnd:\t");
PrintTime(end);
printf("\nDifference: ");
PrintTime(difference);
printf("\n");
// Always free dynamically allocated memory when no longer needed
free(start);
free(end);
free(difference);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

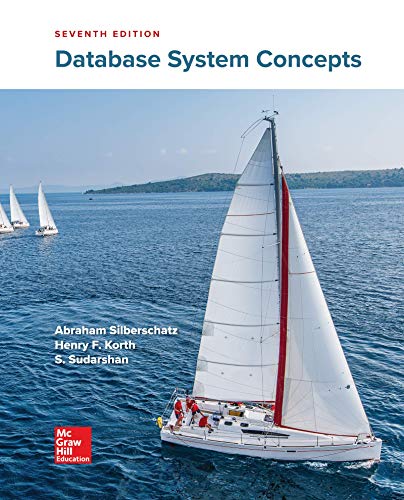
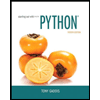
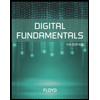
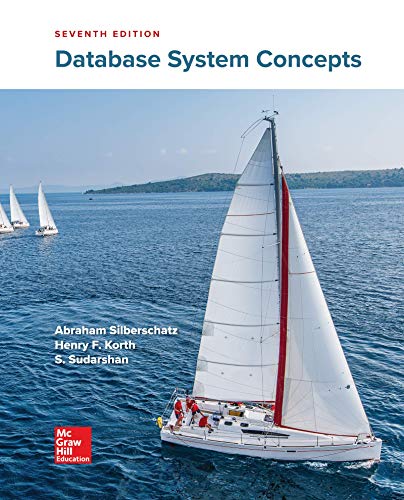
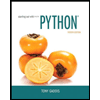
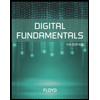
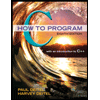
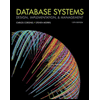
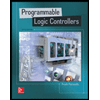