int p =5 , q =6; void foo ( int b , int c ) { b = 2 * c ; p = p + c ; c = 1 + p ; q = q * 2; print ( b + c ); } main () { foo (p , q ); print p , q ; } Explain and print the output of the above code when the parameters to the foo function are passed by value. Explain and print the output of the above code when the parameters to the foo function are passed by reference. Explain and print the output of the above code when the parameters to the foo function are passed by value result. Explain and print the output of the above code when the parameters to the foo function are passed by name
int p =5 , q =6;
void foo ( int b , int c ) {
b = 2 * c ;
p = p + c ;
c = 1 + p ;
q = q * 2;
print ( b + c );
}
main () {
foo (p , q );
print p , q ;
}
Explain and print the output of the above code when the parameters to the foo function are passed by value. Explain and print the output of the above code when the parameters to the foo function are passed by reference. Explain and print the output of the above code when the parameters to the foo function are passed by value result. Explain and print the output of the above code when the parameters to the foo function are passed by name.

When programming, the process of calling a function involves the necessity to provide data. There are multiple techniques for passing this data, each influencing how the data behaves both during and after the function call.
- Passed by Value : The value of the actual parameter is copied to the formal parameter of the function. Any modifications made to the formal parameter do not impact or reflect back on the actual parameter.
- Passed by Reference : When a reference is passed by value, the function receives a copy of the memory location where the actual parameter resides. Any modifications made within the function affect the original parameter.
- Passed by Value Result (or Copy Restore) : The process is as follows: initially, the value of the actual parameter is duplicated to the formal parameter. Once the function finishes its execution, the value of the formal parameter is then copied back to the original actual parameter.
- Passed by Name : The actual code for the parameter replaces the formal parameter in the function body. Consequently, this can result in the evaluation of the actual parameter occurring multiple times.
Step by step
Solved in 3 steps

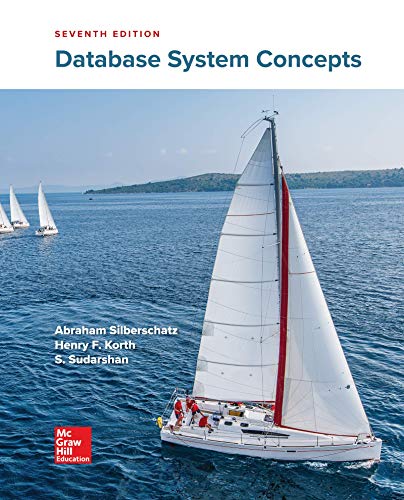
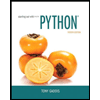
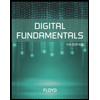
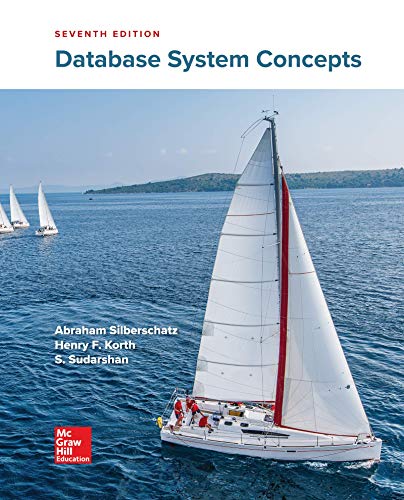
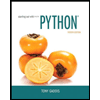
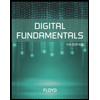
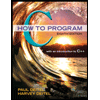
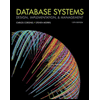
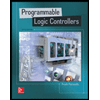