What do you see when you execute "pipe1" and Why? Modify the program pipe1.cpp to pipe1a.cpp so that it accepts a command (e.g. "ls -l") from the keyboard. For example, when you execute "./pipe1a ps -auxw", it should give you the same output as pipe1.cpp. Please explain what you changed and why. (Hint: Use string functions strcpy() and strcat() to store the commands in a buffer. Your main function should be like: int main(int argc, char *argv[])) //pipe1.cpp #include #include #include #include #include using namespace std; int main() { FILE *fpi; //for reading a pipe char buffer[BUFSIZ+1]; //BUFSIZ defined in int chars_read; memset (buffer, 0,sizeof(buffer)); //clear buffer fpi = popen ("ps -auxw", "r"); //pipe to command "ps -auxw" if (fpi != NULL) { //read data from pipe into buffer chars_read = fread(buffer, sizeof(char), BUFSIZ, fpi); if (chars_read > 0) cout << "Output from pipe: " << buffer << endl; pclose (fpi); //close the pipe return 0; } return 1; }
What do you see when you execute "pipe1" and Why? Modify the program pipe1.cpp to pipe1a.cpp so that it accepts a command (e.g. "ls -l") from the keyboard. For example, when you execute "./pipe1a ps -auxw", it should give you the same output as pipe1.cpp. Please explain what you changed and why. (Hint: Use string functions strcpy() and strcat() to store the commands in a buffer. Your main function should be like: int main(int argc, char *argv[])) //pipe1.cpp #include #include #include #include #include using namespace std; int main() { FILE *fpi; //for reading a pipe char buffer[BUFSIZ+1]; //BUFSIZ defined in int chars_read; memset (buffer, 0,sizeof(buffer)); //clear buffer fpi = popen ("ps -auxw", "r"); //pipe to command "ps -auxw" if (fpi != NULL) { //read data from pipe into buffer chars_read = fread(buffer, sizeof(char), BUFSIZ, fpi); if (chars_read > 0) cout << "Output from pipe: " << buffer << endl; pclose (fpi); //close the pipe return 0; } return 1; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
- What do you see when you execute "pipe1" and Why?
- Modify the program pipe1.cpp to pipe1a.cpp so that it accepts a command (e.g. "ls -l") from the keyboard. For example, when you execute "./pipe1a ps -auxw", it should give you the same output as pipe1.cpp. Please explain what you changed and why.
(Hint: Use string functions strcpy() and strcat() to store the commands in a buffer. Your main function should be like: int main(int argc, char *argv[]))
//pipe1.cpp
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
#include <iostream>
using namespace std;
int main()
{
FILE *fpi; //for reading a pipe
char buffer[BUFSIZ+1]; //BUFSIZ defined in <stdio.h>
int chars_read;
memset (buffer, 0,sizeof(buffer)); //clear buffer
fpi = popen ("ps -auxw", "r"); //pipe to command "ps -auxw"
if (fpi != NULL) {
//read data from pipe into buffer
chars_read = fread(buffer, sizeof(char), BUFSIZ, fpi);
if (chars_read > 0)
cout << "Output from pipe: " << buffer << endl;
pclose (fpi); //close the pipe
return 0;
}
return 1;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Recommended textbooks for you
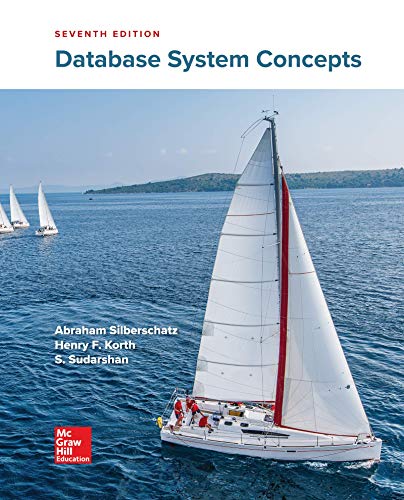
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
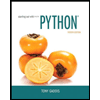
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
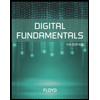
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
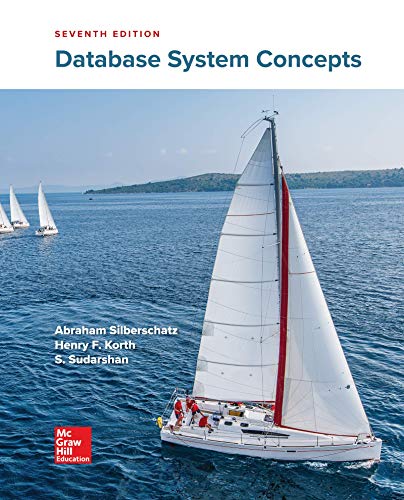
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
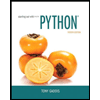
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
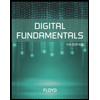
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
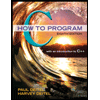
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
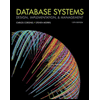
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
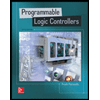
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education