Write a program that will continually ask the user to enter a string from standard input until user enters Q or q to quit. After reading the user’s input string, the program will count number of characters, vowels, lowercase letters, uppercase letters, number of digits, number of other characters and number of words from the user’s input string. You can use getline(cin, inputString) to read entire line. Please refer to my previous lecture on data validation. http://www.cplusplus.com/reference/string/string/getline/ Characters: counts everything (user input length) Vowels: a e i o u A E I O U Lowercase letters: any characters a-z Uppercase letters: any characters A-Z Digits: 0-9 Other characters: mean any character that is not alphanumeric. You can use C++ library function to check if a character is: • islower, isupper: - http://www.cplusplus.com/reference/cctype/islower/ • Make sure you include #include
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Write a
Q or q to quit.
After reading the user’s input string, the program will count number of characters, vowels, lowercase
letters, uppercase letters, number of digits, number of other characters and number of words from the
user’s input string. You can use getline(cin, inputString) to read entire line. Please refer to my previous
lecture on data validation. http://www.cplusplus.com/reference/string/string/getline/
Characters: counts everything (user input length)
Vowels: a e i o u A E I O U
Lowercase letters: any characters a-z
Uppercase letters: any characters A-Z
Digits: 0-9
Other characters: mean any character that is not alphanumeric.
You can use C++ library function to check if a character is:
• islower, isupper: - http://www.cplusplus.com/reference/cctype/islower/
• Make sure you include
#include <ctype.h>


Step by step
Solved in 3 steps with 2 images

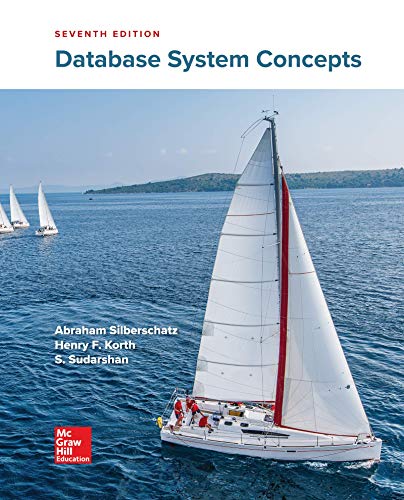
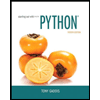
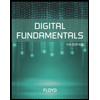
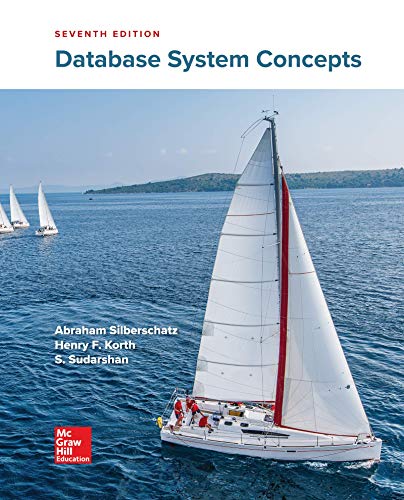
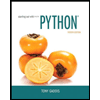
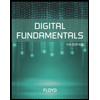
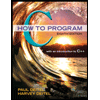
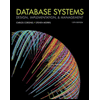
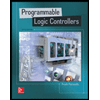