We want to create a program that accepts exactly 100 recycled can donations. Each donation consists of 1 or more cans. Each can represents a $0.05 donation. After collecting all donations, the program prints its monetary value. Complete the code below to create this program: for i in range(100): = int(input("How many cans are you donating? ")) donations += print("The donation value is $", * 0.05)
We want to create a program that accepts exactly 100 recycled can donations. Each donation consists of 1 or more cans. Each can represents a $0.05 donation. After collecting all donations, the program prints its monetary value. Complete the code below to create this program: for i in range(100): = int(input("How many cans are you donating? ")) donations += print("The donation value is $", * 0.05)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:## Collecting Recycled Can Donations
We want to create a program that accepts exactly 100 recycled can donations. Each donation consists of 1 or more cans. Each can represents a $0.05 donation. After collecting all donations, the program prints its monetary value.
### Complete the code below to create this program:
```python
donations = 0
for i in range(100):
cans = int(input("How many cans are you donating? "))
donations += cans
print("The donation value is $", donations * 0.05)
```
### Explanation of Code
This code performs the following tasks:
1. **Initialization**: Initializes the `donations` variable to 0. This variable will accumulate the total number of cans donated.
2. **Loop (for i in range(100))**: Repeats the next block of code 100 times, which corresponds to accepting 100 donations.
3. **Input Collection**: For each iteration, asks the donor to enter the number of cans they are donating.
4. **Accumulation**: Adds the number of cans donated to the total `donations`.
5. **Calculation & Output**: After collecting all donations, calculates the total monetary value of the cans and prints it.
### Drag the appropriate blocks to fill the slots above
- **cans**: Represents the number of cans donated in each iteration.
- **100**: Sets the loop to run exactly 100 times.
- **0**: Initializes the `donations` variable to 0.
- **donations**: Variable that accumulates the total number of cans.
### Program Example Type
This program is an example of an **accumulator** loop pattern.
### Diagram Explanation
The diagram presents an incomplete code snippet with blanks to be filled by dragging appropriate code blocks from a given set. Below the code snippet, several draggable code blocks (`cans`, `100`, `0`, `donations`, `stepwise`, `accumulator`) are provided for completing the code, focusing on using the correct loop pattern and accumulation logic.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
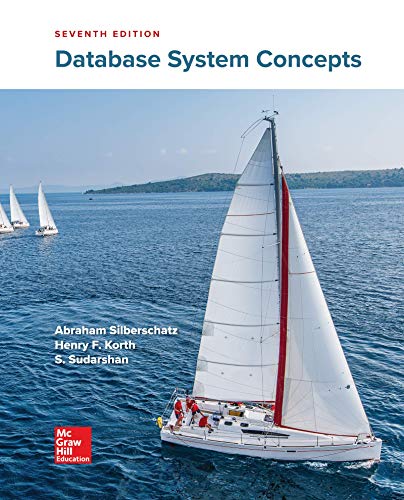
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
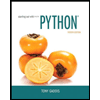
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
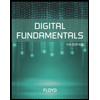
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
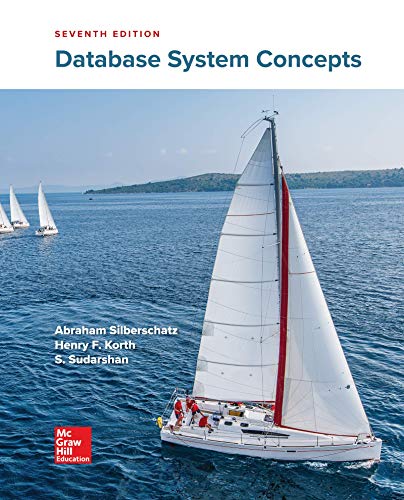
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
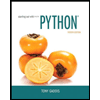
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
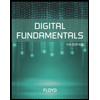
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
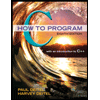
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
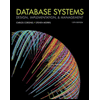
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
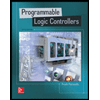
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education