Using TKinter, display the Dictionary in the Dictionary Based Object streamed in question #4. question #4: import socket import json def receive_co2_data(host, port): """ Connects to a CO2 data server at the specified host and port, receives and prints the CO2 data fields, and gracefully closes the connection. Args: - host (str): The hostname or IP address of the server. - port (int): The port number to connect to. """ # Initialize socket client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) try: # Connect to server client_socket.connect((host, port)) print(f"Connected to {host}:{port}") # Receive and print the CO2 data fields buffer = "" while True: data = client_socket.recv(1024).decode() if not data: break # Buffer data and split into JSON-encoded objects buffer += data objects = buffer.split("\n") buffer = objects.pop() # Parse and print each object for obj in objects: try: field_data = json.loads(obj) print(field_data) except json.JSONDecodeError: pass print("Connection closed") except ConnectionRefusedError: print(f"Error: connection to {host}:{port} refused") except OSError as e: print(f"Error: {e}") finally: # Close the socket client_socket.close() if __name__ == "__main__": host = input("Enter server hostname or IP address: ") port = int(input("Enter server port number: ")) receive_co2_data(host, port)
Using TKinter, display the Dictionary in the Dictionary Based Object streamed in question #4.
question #4:
import socket
import json
def receive_co2_data(host, port):
"""
Connects to a CO2 data server at the specified host and port,
receives and prints the CO2 data fields, and gracefully closes
the connection.
Args:
- host (str): The hostname or IP address of the server.
- port (int): The port number to connect to.
"""
# Initialize socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
# Connect to server
client_socket.connect((host, port))
print(f"Connected to {host}:{port}")
# Receive and print the CO2 data fields
buffer = ""
while True:
data = client_socket.recv(1024).decode()
if not data:
break
# Buffer data and split into JSON-encoded objects
buffer += data
objects = buffer.split("\n")
buffer = objects.pop()
# Parse and print each object
for obj in objects:
try:
field_data = json.loads(obj)
print(field_data)
except json.JSONDecodeError:
pass
print("Connection closed")
except ConnectionRefusedError:
print(f"Error: connection to {host}:{port} refused")
except OSError as e:
print(f"Error: {e}")
finally:
# Close the socket
client_socket.close()
if __name__ == "__main__":
host = input("Enter server hostname or IP address: ")
port = int(input("Enter server port number: "))
receive_co2_data(host, port)

Step by step
Solved in 3 steps

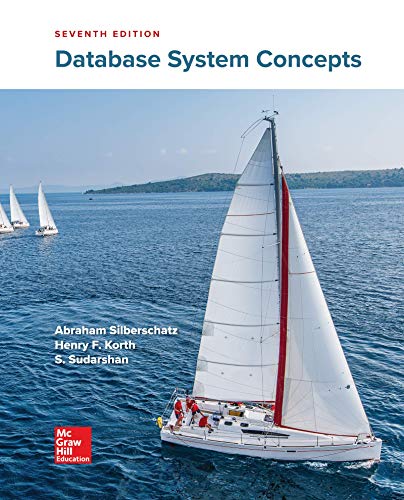
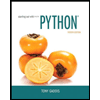
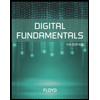
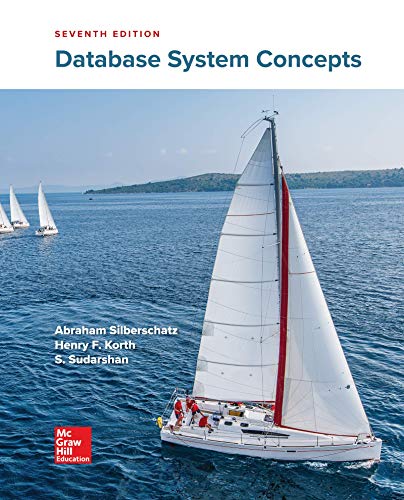
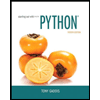
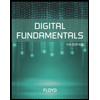
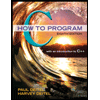
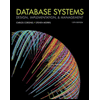
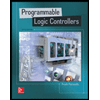