Using this Java code convert it to C++: public class Program2 { public static void main(String arg[]) { //variables to store converted temperature value double Fahreinheit; double Celcius; //Check variable to control while loop char Check = 'Y'; Scanner input = new Scanner(System.in); System.out.println("This program converts Temperatures from Fahrenheit to Celsius and vice versa."); while (Check == 'Y' || Check == 'y') { //try block to catch the invalid input exception try { //get user input for temperature System.out.print("Please enter your temperature: "); double Temperature = input.nextDouble(); //get user input for the temperature unit designation System.out.print("Please enter the units (F/C): "); char Unit = input.next().charAt(0); //if unit is Fahrenheit if (Unit == 'F') { //convert to Celsius Celcius = (Temperature - 32) * 5 / 9; System.out.println("A temperature of " + Temperature + " degrees Fahrenheit is equivalent to " + Celcius + " degrees Celsius."); } //unit is Celsius else if (Unit == 'C') { //convert to Fahrenheit Fahreinheit = ((9 * Temperature) / 5) + 32; System.out.println("A temperature of " + Temperature + " degrees Celsius is equivalent to " + Fahreinheit + " degrees Fahrenheit."); } //ask user if they want to continue? System.out.print("Do you wish another conversion? (Y/N): "); Check = input.next().charAt(0); System.out.println(); } //catch block to handle and display the exception message and stop the program catch (Exception e) { System.out.println("Invalid Input"); break; } } //when user wishes to discontinue, prints Thank You. Goodbye message to console if (Check == 'N') { System.out.println("Thank you. Goodbye."); } }}
Using this Java code convert it to C++:
public class Program2 {
public static void main(String arg[]) {
//variables to store converted temperature value
double Fahreinheit;
double Celcius;
//Check variable to control while loop
char Check = 'Y';
Scanner input = new Scanner(System.in);
System.out.println("This
while (Check == 'Y' || Check == 'y') {
//try block to catch the invalid input exception
try {
//get user input for temperature
System.out.print("Please enter your temperature: ");
double Temperature = input.nextDouble();
//get user input for the temperature unit designation
System.out.print("Please enter the units (F/C): ");
char Unit = input.next().charAt(0);
//if unit is Fahrenheit
if (Unit == 'F') {
//convert to Celsius
Celcius = (Temperature - 32) * 5 / 9;
System.out.println("A temperature of " + Temperature + " degrees Fahrenheit is equivalent to " + Celcius + " degrees Celsius.");
} //unit is Celsius
else if (Unit == 'C') {
//convert to Fahrenheit
Fahreinheit = ((9 * Temperature) / 5) + 32;
System.out.println("A temperature of " + Temperature + " degrees Celsius is equivalent to " + Fahreinheit + " degrees Fahrenheit.");
}
//ask user if they want to continue?
System.out.print("Do you wish another conversion? (Y/N): ");
Check = input.next().charAt(0);
System.out.println();
} //catch block to handle and display the exception message and stop the program
catch (Exception e) {
System.out.println("Invalid Input");
break;
}
}
//when user wishes to discontinue, prints Thank You. Goodbye message to console
if (Check == 'N') {
System.out.println("Thank you. Goodbye.");
}
}
}

Step by step
Solved in 2 steps with 3 images

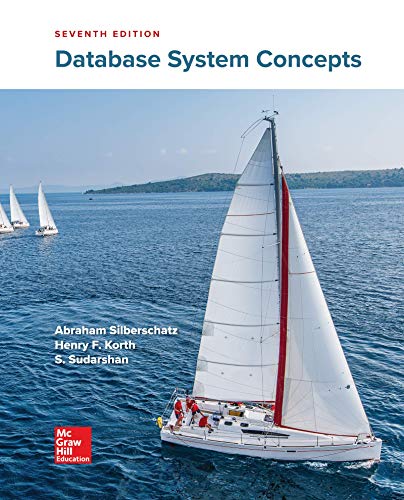
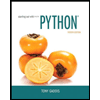
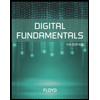
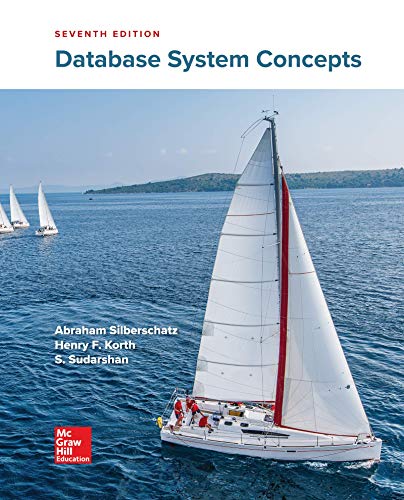
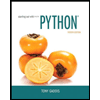
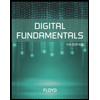
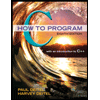
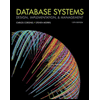
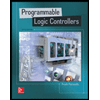