using System.Collections.Generic; using System.Globalization; class GreenvilleRevenue { static void Main() { int contestantsLastYear; int contestantsThisYear; string contestantName;
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
THIS IS FOR C#. I need help fixing the code.
Here is my code:
using System;
using System.Collections.Generic;
using System.Globalization;
class GreenvilleRevenue
{
static void Main()
{
int contestantsLastYear;
int contestantsThisYear;
string contestantName;
string talentCode;
List<string> talentCodes = new List<string>();
List<string> contestantNames = new List<string>();
Console.Write("Enter number of contestants last year >> ");
while (!int.TryParse(Console.ReadLine(), out contestantsLastYear))
{
Console.WriteLine("Invalid format - entry must be a number");
Console.Write("Enter number of contestants last year >> ");
}
Console.Write("Enter number of contestants this year >> ");
while (!int.TryParse(Console.ReadLine(), out contestantsThisYear))
{
Console.WriteLine("Invalid format - entry must be a number");
Console.Write("Enter number of contestants this year >> ");
}
Console.WriteLine("Last year's competition had {0} contestants, and this year's has {1} contestants", contestantsLastYear, contestantsThisYear);
Console.WriteLine("Revenue expected this year is {0:C}", contestantsThisYear * 25);
if (contestantsThisYear > contestantsLastYear)
{
Console.WriteLine("The competition is bigger than ever!");
}
else
{
Console.WriteLine("The competition is the same as last year");
}
while (true)
{
Console.Write("Enter contestant name >> ");
contestantName = Console.ReadLine();
if (contestantName == "")
{
break;
}
Console.WriteLine("Talent codes are:");
Console.WriteLine(" S Singing");
Console.WriteLine(" D Dancing");
Console.WriteLine(" M Musical instrument");
Console.WriteLine(" O Other");
Console.Write(" Enter talent code >> ");
talentCode = Console.ReadLine();
while (talentCode != "S" && talentCode != "D" && talentCode != "M" && talentCode != "O")
{
Console.WriteLine("Invalid format - entry must be a single character");
Console.WriteLine("That is not a valid code");
Console.Write(" Enter talent code >> ");
talentCode = Console.ReadLine();
}
talentCodes.Add(talentCode);
contestantNames.Add(contestantName);
}
Console.WriteLine("\nThe types of talent are:");
Console.WriteLine("Singing {0}", getLists(talentCodes, "S"));
Console.WriteLine("Dancing {0}", getLists(talentCodes, "D"));
Console.WriteLine("Musical instrument {0}", getLists(talentCodes, "M"));
Console.WriteLine("Other {0}", getLists(talentCodes, "O"));
string talentType;
Console.Write("\nEnter a talent type or Z to quit >> ");
talentType = Console.ReadLine();
while (talentType != "Z")
{
Console.WriteLine();
getContestantData(talentType, talentCodes, contestantNames);
Console.Write("\nEnter a talent type or Z to quit >> ");
talentType = Console.ReadLine();
}
}
static int getLists(List<string> talentCodes, string talentCode)
{
int count = 0;
foreach (string code in talentCodes)
{
if (code == talentCode)
{
count++;
}
}
return count;
}
static void getContestantData(string talentType, List<string> talentCodes, List<string> contestantNames)
{
Console.WriteLine("Contestants with talent type {0} are:", talentType);
for (int i = 0; i < talentCodes.Count; i++)
{
if (talentCodes[i] == talentType)
{
Console.WriteLine(" {0}", contestantNames[i]);
}
}
}
}
In Chapter 7, you modified the GreenvilleRevenue program to include a number of methods.
Now, using your code from Chapter 7 Case Study 1, modify your program so every data entry statement uses a TryParse() method to ensure that each piece of data is the correct type.
Any invalid user entries should generate an appropriate message that contains the word Invalid, and the user should be required to reenter the data.
I have submitted this multiple times, so please help fix this.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

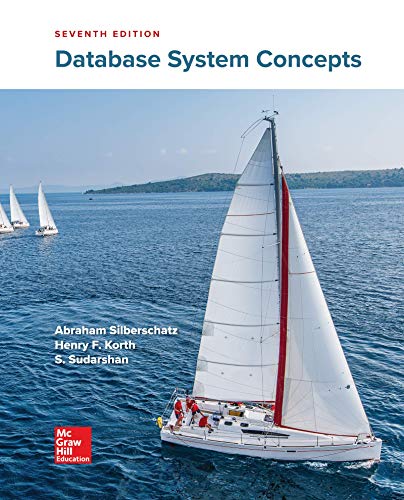
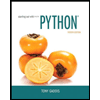
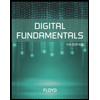
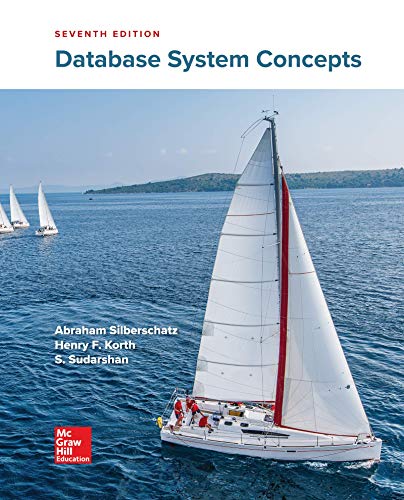
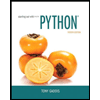
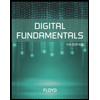
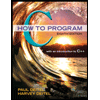
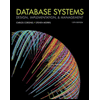
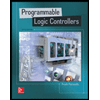