using System; namespace Employee_Class { class Employee { const double SALE_PERCENT = 0.09, FED_TAX_RATE = 0.18; const double RETIRE_CONTRIBUTION = 0.10, SS_TAX_RATE = 0.06; private int empNum; private string firstName; private string lastName; private double totalSales; public Employee() { empNum = 0; firstName = ""; lastName = ""; totalSales = 0.0; } public Employee(int empNum, string firstName, string lastName, double totalSales) { this.empNum = empNum; this.firstName = firstName; this.lastName = lastName; this.totalSales = totalSales; } public double calculateTakeHomePay() { double pay = totalSales * SALE_PERCENT; pay = pay - pay * FED_TAX_RATE - pay * RETIRE_CONTRIBUTION - pay * SS_TAX_RATE; return pay; } public void display() { Console.WriteLine("Employee Number: {0}, Name: {1} {2}, Total Sale: {3}", empNum, firstName, lastName, totalSales.ToString("C")); } public double getTotalSales() { return totalSales; } public void setTotalSales(double sale) { totalSales = sale; } public int EmpNum { get => empNum; set => empNum = value; } public string FirstName { get => firstName; set => firstName = value; } public string LastName { get => lastName; set => lastName = value; } public double TotalSale { get => totalSales; set => totalSales = value; } } class TestEmployee { static void Main(string[] args) { Employee emp1 = new Employee(1, "Mike", "Johnson", 50000); emp1.display(); Console.WriteLine("Take home Pay: {0}\n", emp1.calculateTakeHomePay().ToString("C")); Console.ReadLine(); } } }
Can someone create a UML design diagram for the code I provided here? Please and thank you!
using System;
namespace Employee_Class
{
class Employee
{
const double SALE_PERCENT = 0.09, FED_TAX_RATE = 0.18;
const double RETIRE_CONTRIBUTION = 0.10, SS_TAX_RATE = 0.06;
private int empNum;
private string firstName;
private string lastName;
private double totalSales;
public Employee()
{
empNum = 0;
firstName = "";
lastName = "";
totalSales = 0.0;
}
public Employee(int empNum, string firstName, string lastName, double totalSales)
{
this.empNum = empNum;
this.firstName = firstName;
this.lastName = lastName;
this.totalSales = totalSales;
}
public double calculateTakeHomePay()
{
double pay = totalSales * SALE_PERCENT;
pay = pay - pay * FED_TAX_RATE - pay * RETIRE_CONTRIBUTION - pay * SS_TAX_RATE;
return pay;
}
public void display()
{
Console.WriteLine("Employee Number: {0}, Name: {1} {2}, Total Sale: {3}", empNum, firstName, lastName, totalSales.ToString("C"));
}
public double getTotalSales()
{
return totalSales;
}
public void setTotalSales(double sale)
{
totalSales = sale;
}
public int EmpNum
{
get => empNum;
set => empNum = value;
}
public string FirstName
{
get => firstName;
set => firstName = value;
}
public string LastName
{
get => lastName;
set => lastName = value;
}
public double TotalSale
{
get => totalSales;
set => totalSales = value;
}
}
class TestEmployee
{
static void Main(string[] args)
{
Employee emp1 = new Employee(1, "Mike", "Johnson", 50000);
emp1.display();
Console.WriteLine("Take home Pay: {0}\n", emp1.calculateTakeHomePay().ToString("C"));
Console.ReadLine();
}
}
}

Step by step
Solved in 2 steps with 1 images

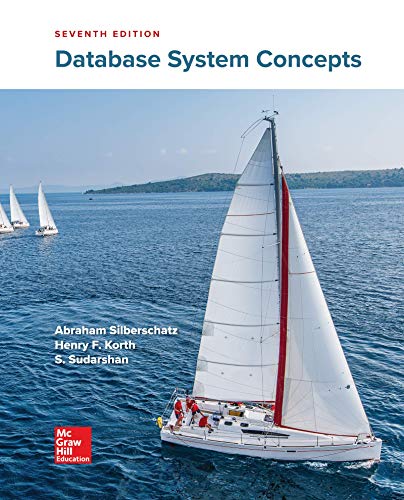
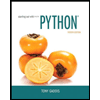
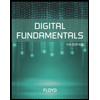
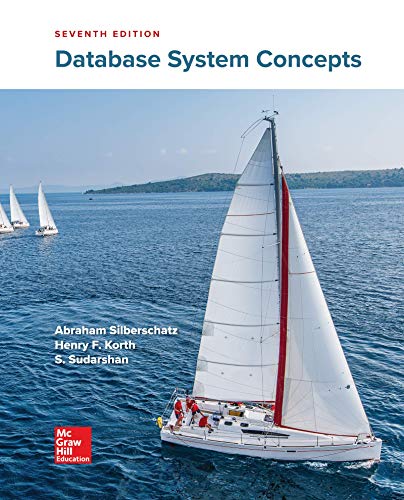
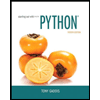
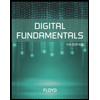
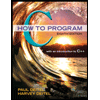
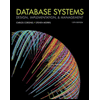
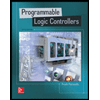