UML Diagrams Provide a UML diagram for the RetailItem class, using the table provided on page 4 of this document. Discussion on UML diagrams can be found throughout Chapter 6. Be sure to include all of the following: Member variables and member functions Access specifiers for all member variables and member functions Data types for all member variables Data types for all parameters Return types for all member functions and constructors public class RetailItem { private String description; // Item description private int unitsOnHand; // Number of units on hand private double price; // Unit price /** This constructor initializes the item's description with an empty string, units on hand to 0, and price to 0.0. */ public RetailItem() { description = ""; unitsOnHand = 0; price = 0.0; } /** This constructor initializes the item's description, units on hand, and price with values passed as arguments. @param d The item's description. @param u The number of units on hand. @param p The item's price. */ public RetailItem(String d, int u, double p) { description = d; unitsOnHand = u; price = p; } /** The setDescription method sets the item's description. @param d The item's description. */ public void setDescription(String d) { description = d; } /** The setUnitsOnHand method sets the item's number of units on hand. @param u The number of units on hand. */ public void setUnitsOnHand(int u) { unitsOnHand = u; } /** The setPrice method sets the item's price. @param p The item's price. */ public void setPrice(double p) { price = p; } /** The getDescription method returns the item's description. @return The item's description. */ public String getDescription() { return description; } /** The getUnitsOnHand method returns the item's number of units on hand. @return The units on hand. */ public int getUnitsOnHand() { return unitsOnHand; } /** The getPrice method rerurns the item's price. @return The item's price. */ public double getPrice() { return price; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
UML Diagrams
Provide a UML diagram for the RetailItem class, using the table provided on page 4 of this document. Discussion on UML diagrams can be found throughout Chapter 6. Be sure to include all of the following:
- Member variables and member functions
- Access specifiers for all member variables and member functions
- Data types for all member variables
- Data types for all parameters
- Return types for all member functions and constructors
public class RetailItem
{
private String description; // Item description
private int unitsOnHand; // Number of units on hand
private double price; // Unit price
/**
This constructor initializes the item's
description with an empty string, units on hand
to 0, and price to 0.0.
*/
public RetailItem()
{
description = "";
unitsOnHand = 0;
price = 0.0;
}
/**
This constructor initializes the item's
description, units on hand, and price with
values passed as arguments.
@param d The item's description.
@param u The number of units on hand.
@param p The item's price.
*/
public RetailItem(String d, int u, double p)
{
description = d;
unitsOnHand = u;
price = p;
}
/**
The setDescription method sets the item's
description.
@param d The item's description.
*/
public void setDescription(String d)
{
description = d;
}
/**
The setUnitsOnHand method sets the item's
number of units on hand.
@param u The number of units on hand.
*/
public void setUnitsOnHand(int u)
{
unitsOnHand = u;
}
/**
The setPrice method sets the item's price.
@param p The item's price.
*/
public void setPrice(double p)
{
price = p;
}
/**
The getDescription method returns the item's
description.
@return The item's description.
*/
public String getDescription()
{
return description;
}
/**
The getUnitsOnHand method returns the item's
number of units on hand.
@return The units on hand.
*/
public int getUnitsOnHand()
{
return unitsOnHand;
}
/**
The getPrice method rerurns the item's price.
@return The item's price.
*/
public double getPrice()
{
return price;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

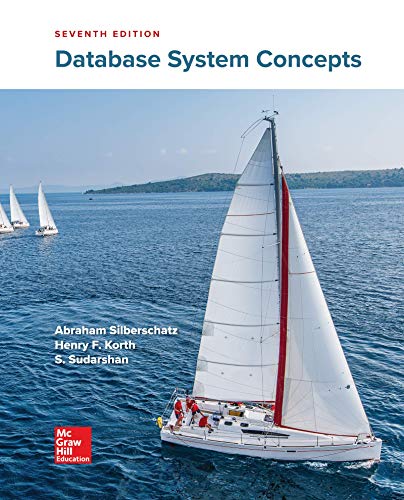
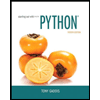
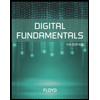
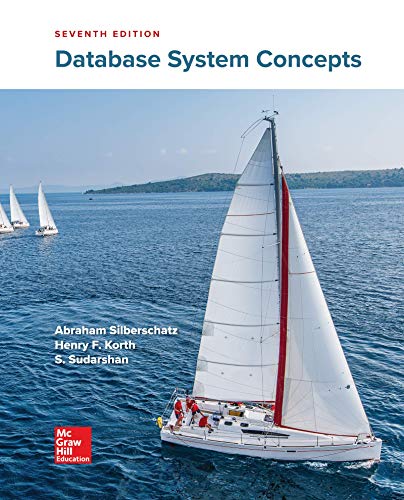
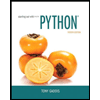
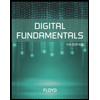
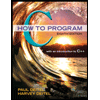
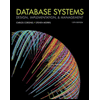
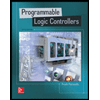