USING JAVA Modify the code to use Arraylist Instead of Hashmap
Please do not give solution in image format thanku
USING JAVA Modify the code to use Arraylist Instead of Hashmap
CODE:
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
class Student {
private String name;
private List<String> certificates;
public Student(String name) {
this.name = name;
this.certificates = new ArrayList<>();
}
public void addCertificate(String certificate) {
certificates.add(certificate);
}
public boolean hasCertificate(String certificate) {
return certificates.contains(certificate);
}
public String getName() {
return name;
}
}
public class CertificateManagement {
public static void main(String[] args) {
Map<String, Student> students = new HashMap<>();
Student student1 = new Student("John");
student1.addCertificate("Java");
student1.addCertificate("Python");
students.put("John", student1);
Student student2 = new Student("Alice");
student2.addCertificate("C++");
students.put("Alice", student2);
Student student3 = new Student("Emma");
student3.addCertificate("Java");
students.put("Emma", student3);
//CHANGE FOR WHEN YOU SEARCH FOR STUDENT AS INSTRUCTED
String targetCertificate = "Java";
List<String> studentsWithCertificate = new ArrayList<>();
for (Student student : students.values()) {
if (student.hasCertificate(targetCertificate)) {
studentsWithCertificate.add(student.getName());
}
}
System.out.println("Students with " + targetCertificate + " certificate:");
for (String name : studentsWithCertificate) {
System.out.println(name);
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

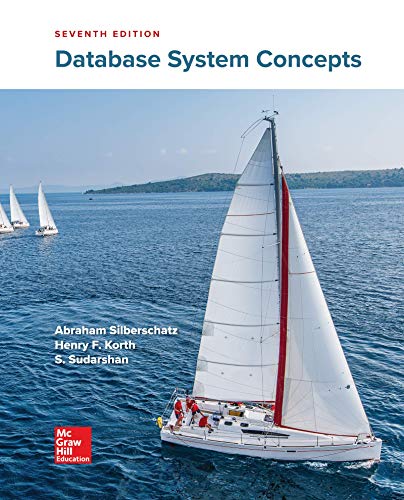
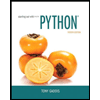
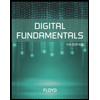
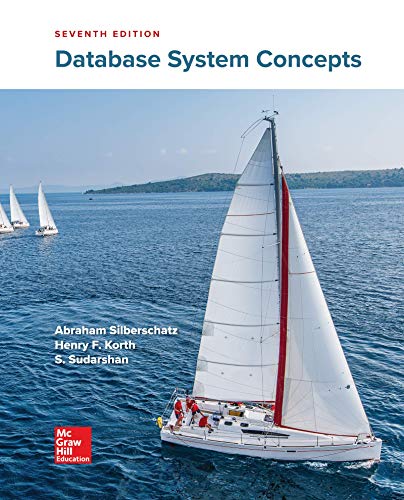
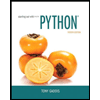
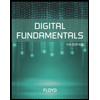
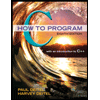
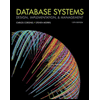
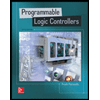