using import java.util.Collections; make the merge sort algorithm to implement the MergeSort sort method public class Person implements Comparable { private String firstName; private String lastName; private int age; Person(String firstName, String lastName, int age) { this.firstName = firstName; this.lastName = lastName; this.age = age; } @Override public String toString() { return lastName + ", " + firstName + " " + age; } @Override public int compareTo(Person other) { return this.lastName.compareTo(other.lastName); } } import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { List people = new ArrayList<>(); people.add(new Person("Frank", "Denton", 73)); people.add(new Person("Mark", "Cohen", 44)); people.add(new Person("Tim", "Smith", 22)); people.add(new Person("Steve", "Denton", 16)); people.add(new Person("Andy", "Ashton", 44)); people.add(new Person("Albert", "Denton", 58)); System.out.println("Before: " + people); List result = MergeSort.sort(people); System.out.println("After: " + result); } } import java.util.Collections; import java.util.List; public class MergeSort { public static List sort(List people) { } }
using import java.util.Collections; make the merge sort
public class Person implements Comparable<Person> {
private String firstName;
private String lastName;
private int age;
Person(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
@Override
public String toString() {
return lastName + ", " + firstName + " " + age;
}
@Override
public int compareTo(Person other) {
return this.lastName.compareTo(other.lastName);
}
}
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Person> people = new ArrayList<>();
people.add(new Person("Frank", "Denton", 73));
people.add(new Person("Mark", "Cohen", 44));
people.add(new Person("Tim", "Smith", 22));
people.add(new Person("Steve", "Denton", 16));
people.add(new Person("Andy", "Ashton", 44));
people.add(new Person("Albert", "Denton", 58));
System.out.println("Before: " + people);
List<Person> result = MergeSort.sort(people);
System.out.println("After: " + result);
}
}
import java.util.Collections;
import java.util.List;
public class MergeSort {
public static List<Person> sort(List<Person> people) {
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

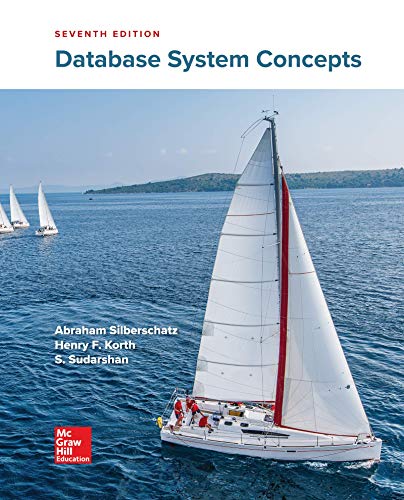
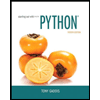
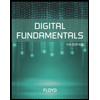
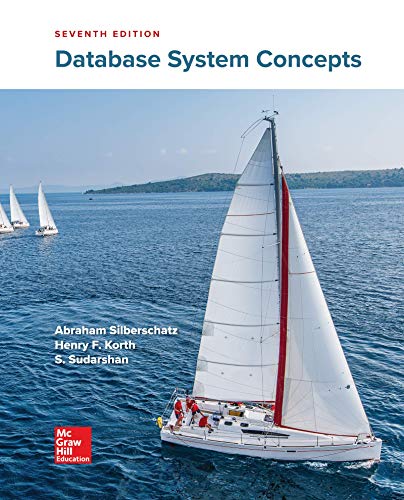
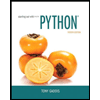
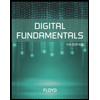
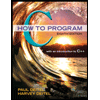
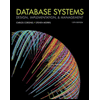
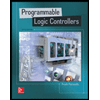