Use the following code which adds, searches and prints elements of a doubly linked list, and add a member function that will union 2 doubly linked lists and store the union elements in a new list. Call your function properly in main and print all 3 lists. Note: You can consider that each list is a set, where no redundant elements are found. #include using namespace std; struct node { int data; node *next,*prev; node(int d,node*n=0,node *p=0) { data=d; next=n; prev=p; }
Use the following code which adds, searches and prints elements of a doubly linked list,
and add a member function that will union 2 doubly linked lists and store the union elements in a new list. Call your function properly in main and print all 3 lists.
Note: You can consider that each list is a set, where no redundant elements are found.
#include <iostream>
using namespace std;
struct node
{
int data;
node *next,*prev;
node(int d,node*n=0,node *p=0)
{ data=d; next=n; prev=p; }
};
class list
{
node *head;
public:
list() { head=0; }
void print()
{
for (node *t=head;t!=0;t=t->next) cout<<t->data<<" ";
cout<<endl;
}
bool search(int el)
{
for (node *t=head;t!=0;t=t->next)
if(t->data==el) return true;
return false;
}
void add(int el)
{
if(head==0)
head=new node(el);
else
{
node *t=head;
for(;t->next!=0;t=t->next);
t->next=new node(el,0,t);
}
}
};
void main()
{
list l1,l2,l3;
l1.add(0);
l1.add(7);
l1.add(1);
l1.add(9);
l1.add(3);
cout<<"Elements of l1 list"<<endl;
l1.print();
l2.add(1);
l2.add(8);
l2.add(2);
l2.add(9);
l2.add(0);
l2.add(4);
cout<<"Elements of l2 list"<<endl;
l2.print();
}

Step by step
Solved in 2 steps with 1 images

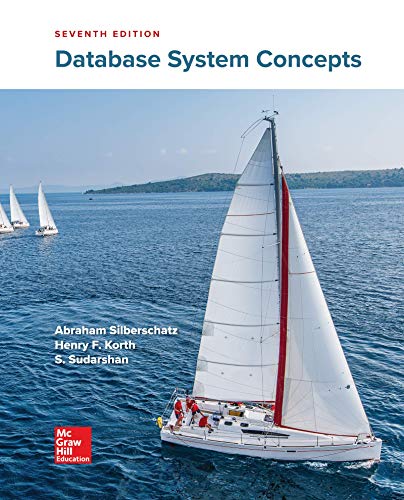
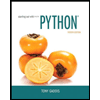
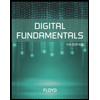
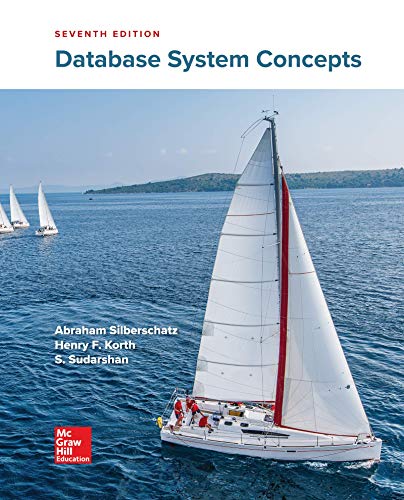
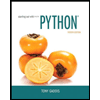
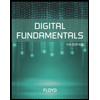
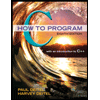
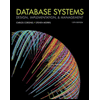
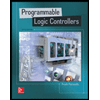