Use a Linux machine and run the system calls, fork(), wait() and exec() Write a program named as “testOS.c” that executes the “cat –b –v –t filename” command in the child process. #include <stdio.h> #include <sys/types.h> #include <unistd.h> int main (int args, char *argv[]) { pid_t fork_return; pid_t pid; pid=getpid(); fork_return = fork(); if (fork_return==-1) { // When fork() returns -1, an error has happened. printf("\nError creating process " ); return 0; } if (fork_return==0) { // When fork() returns 0, we are in the child process. printf("\n\nThe values are Child ID = %d, Parent ID=%d\n", getpid(), getppid()); execl("/bin/cat", "cat", "-b", "-v", "-t", argv[1], NULL); } else { // When fork() returns a positive number, we are in the parent process // and the return value is the PID of the newly created child process. wait(NULL); printf("\nChild Completes " ); printf("\nIn the Parent Process\n"); printf("Child Id = %d, Parent ID = %d\n", getpid(), getppid()); } return 0; } Code for “testOS.c” to compile and run: Details: Type the following program in Linux Compile it using gcc as gcc testOS.c Run the program as the following command: ./a.out filename where filename is the name of a text file that you have created. The text file should contain multiple lines of text. HiNT: What should happen: The code will fork(), The child will use the execl to call cat and use the filename passed as an argument on the command line. The parent will wait for the child to finish. Your program will print from both processes: The process id The parent id
Use a Linux machine and run the system calls, fork(), wait() and exec()
Write a
#include <stdio.h>
#include <sys/types.h>
#include <unistd.h>
int main (int args, char *argv[])
{
pid_t fork_return;
pid_t pid;
pid=getpid();
fork_return = fork();
// When fork() returns -1, an error has happened.
printf("\nError creating process " );
return 0;
}
if (fork_return==0) {
// When fork() returns 0, we are in the child process.
printf("\n\nThe values are Child ID = %d, Parent ID=%d\n", getpid(), getppid());
execl("/bin/cat", "cat", "-b", "-v", "-t", argv[1], NULL);
}
else {
// When fork() returns a positive number, we are in the parent process
// and the return value is the PID of the newly created child process.
wait(NULL);
printf("\nChild Completes " );
printf("\nIn the Parent Process\n");
printf("Child Id = %d, Parent ID = %d\n", getpid(), getppid());
}
return 0;
}
Code for “testOS.c” to compile and run:
Details:
-
Type the following program in Linux
-
Compile it using gcc as gcc testOS.c
-
Run the program as the following command: ./a.out filename where filename is the name of a text file that you have created. The text file should contain multiple lines of text.
HiNT:
What should happen:
The code will fork(), The child will use the execl to call cat and use the filename passed as an argument on the command line. The parent will wait for the child to finish. Your program will print from both processes:
-
The process id
-
The parent id
Unlock instant AI solutions
Tap the button
to generate a solution
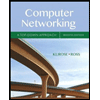
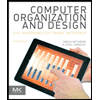
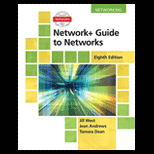
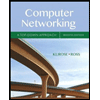
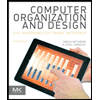
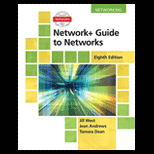
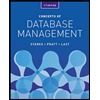
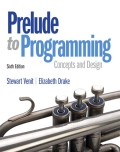
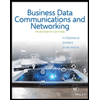