In c++ LinkedList class You will create a class “LinkedList” with the following private data attributes: headPtr – raw pointer to the head of the list numItems – number of items in the list Put your class definition in a header file and the implementation of the methods in a .cpp file. Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods: Accessor to get the current size (numItems) Constructor that initializes numItems to zero and headPtr to nullptr addNode – this method will take as input one string value. It will then create a node object and set the attribute. Then it will put it in the linked list at the correct position – ascending order – avoiding duplicates. You will do all of the work while building the linked list. toVector – returns vector with the contents of the list. You will use only the “push_back” method to get the strings into the vector.
In c++
-
LinkedList class
You will create a class “LinkedList” with the following private data attributes:
-
headPtr – raw pointer to the head of the list
-
numItems – number of items in the list
Put your class definition in a header file and the implementation of the methods in a .cpp file.
Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods:-
Accessor to get the current size (numItems)
-
Constructor that initializes numItems to zero and headPtr to nullptr
-
addNode – this method will take as input one string value. It will then create a node object and set the attribute. Then it will put it in the linked list at the correct position – ascending order – avoiding duplicates. You will do all of the work while building the linked list.
-
toVector – returns
vector with the contents of the list. You will use only the “push_back” method to get the strings into the vector.
-
-

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Unfortunately instructions do not allow for a method that removes duplicates after linked list is created. How would you sort the file through the addNode Method while the list is being built?
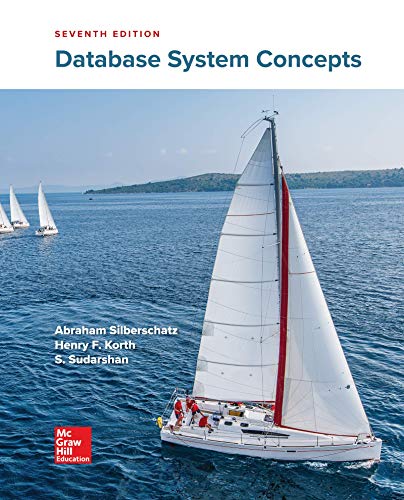
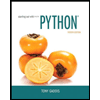
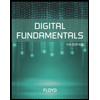
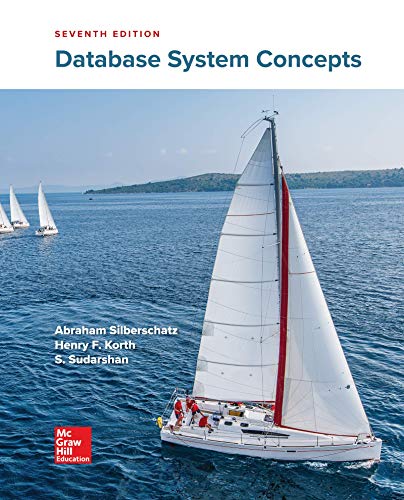
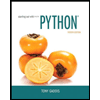
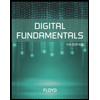
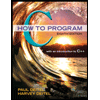
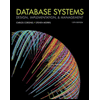
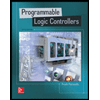