TRUCTION: make this a flowchart import java.util.HashSet; import java.util.Set; public class elements { public static void main(String[] args) { Set A = new HashSet<>(); A.add("one"); A.add("two"); A.add("three"); A.add("four"); Set B = new HashSet<>(); B.add("two"); B.add("three"); B.add("five"); B.add("six"); System.out.println("A: " + A); System.out.println("B: " + B); unionOp(A, B); intersectionOp(A, B); differenceOp(A, B); checkSubset(A, B); } public static void unionOp(Set A, Set B) { // To perform union Set AUnionB = new HashSet<>(A); AUnionB.addAll(B); System.out.println("A union B: " + AUnionB); } public static void intersectionOp(Set A, Set B) { // To perform Intersection Set AIntersectionB = new HashSet<>(A); AIntersectionB.retainAll(B); System.out.println("A intersection B: " + AIntersectionB); } public static void differenceOp(Set A, Set B) { // To find difference Set ADifferenceB = new HashSet<>(A); ADifferenceB.removeAll(B); Set BDifferenceA = new HashSet<>(B); BDifferenceA.removeAll(A); System.out.println("A difference B: " + ADifferenceB); System.out.println("B difference A: " + BDifferenceA); } public static void checkSubset(Set A, Set B) { // To check for subset System.out.println("B is subset A: " + A.containsAll(B)); System.out.println("A is subset B: " + B.containsAll(A));
TRUCTION: make this a flowchart import java.util.HashSet; import java.util.Set; public class elements { public static void main(String[] args) { Set A = new HashSet<>(); A.add("one"); A.add("two"); A.add("three"); A.add("four"); Set B = new HashSet<>(); B.add("two"); B.add("three"); B.add("five"); B.add("six"); System.out.println("A: " + A); System.out.println("B: " + B); unionOp(A, B); intersectionOp(A, B); differenceOp(A, B); checkSubset(A, B); } public static void unionOp(Set A, Set B) { // To perform union Set AUnionB = new HashSet<>(A); AUnionB.addAll(B); System.out.println("A union B: " + AUnionB); } public static void intersectionOp(Set A, Set B) { // To perform Intersection Set AIntersectionB = new HashSet<>(A); AIntersectionB.retainAll(B); System.out.println("A intersection B: " + AIntersectionB); } public static void differenceOp(Set A, Set B) { // To find difference Set ADifferenceB = new HashSet<>(A); ADifferenceB.removeAll(B); Set BDifferenceA = new HashSet<>(B); BDifferenceA.removeAll(A); System.out.println("A difference B: " + ADifferenceB); System.out.println("B difference A: " + BDifferenceA); } public static void checkSubset(Set A, Set B) { // To check for subset System.out.println("B is subset A: " + A.containsAll(B)); System.out.println("A is subset B: " + B.containsAll(A));
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
INSTRUCTION: make this a flowchart
import java.util.HashSet;
import java.util.Set;
public class elements {
public static void main(String[] args) {
Set A = new HashSet<>();
A.add("one");
A.add("two");
A.add("three");
A.add("four");
Set B = new HashSet<>();
B.add("two");
B.add("three");
B.add("five");
B.add("six");
System.out.println("A: " + A);
System.out.println("B: " + B);
unionOp(A, B);
intersectionOp(A, B);
differenceOp(A, B);
checkSubset(A, B);
}
public static void unionOp(Set A, Set B) { // To perform union
Set AUnionB = new HashSet<>(A);
AUnionB.addAll(B);
System.out.println("A union B: " + AUnionB);
}
public static void intersectionOp(Set A, Set B) { // To perform Intersection
Set AIntersectionB = new HashSet<>(A);
AIntersectionB.retainAll(B);
System.out.println("A intersection B: " + AIntersectionB);
}
public static void differenceOp(Set A, Set B) { // To find difference
Set ADifferenceB = new HashSet<>(A);
ADifferenceB.removeAll(B);
Set BDifferenceA = new HashSet<>(B);
BDifferenceA.removeAll(A);
System.out.println("A difference B: " + ADifferenceB);
System.out.println("B difference A: " + BDifferenceA);
}
public static void checkSubset(Set A, Set B) { // To check for subset
System.out.println("B is subset A: " + A.containsAll(B));
System.out.println("A is subset B: " + B.containsAll(A));
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
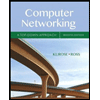
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
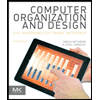
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
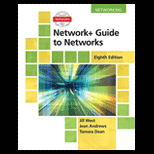
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
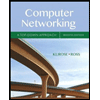
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
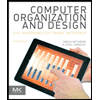
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
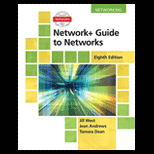
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
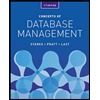
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
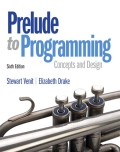
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
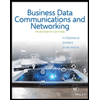
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY