Trace the following fragment for a Stack s and an empty queue q (type Queue). Stacks First Second Third Queue a String item; while (!s.empty()) { item = s.pop(); q.offer (item); } q.offer (" hi"); } while (!q.isEmpty()) { item = q.remove() s.push (item); s.push (" there");
Trace the following fragment for a Stack s and an empty queue q (type Queue). Stacks First Second Third Queue a String item; while (!s.empty()) { item = s.pop(); q.offer (item); } q.offer (" hi"); } while (!q.isEmpty()) { item = q.remove() s.push (item); s.push (" there");
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Trace the following fragment for a Stack<String> s and an empty queue q (type Queue<String>).**
---
**Diagram Explanation:**
- **Stack s:**
- The stack initially contains three elements:
- "First"
- "Second"
- "Third" (at the top)
- **Queue q:**
- The queue is initially empty.
---
**Code Explanation:**
```java
String item;
while (!s.empty()) {
item = s.pop();
q.offer(item);
q.offer(" hi");
}
while (!q.isEmpty()) {
item = q.remove();
s.push(item);
s.push(" there");
}
```
This code consists of two main loops. Here's a step-by-step explanation of what each part of the code does:
1. **First Loop:**
- The loop continues as long as the stack `s` is not empty.
- Each iteration:
- Removes the top item from the stack `s` and stores it in `item`.
- Adds `item` to the queue `q`.
- Adds the string " hi" to the queue `q`.
2. **Second Loop:**
- This loop continues as long as the queue `q` is not empty.
- Each iteration:
- Removes the front item from the queue `q` and stores it in `item`.
- Pushes `item` onto the stack `s`.
- Pushes the string " there" onto the stack `s`.
Expert Solution

Step 1
The above question is solved in step 2 :-
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
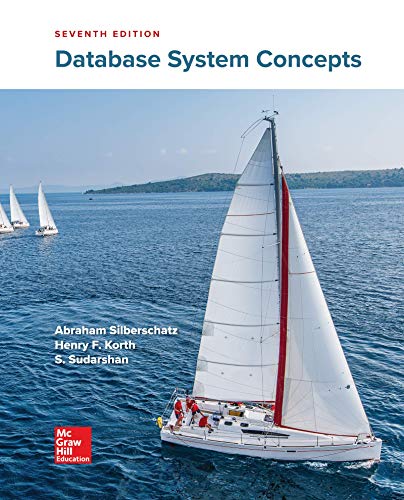
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
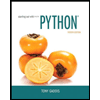
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
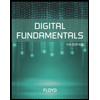
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
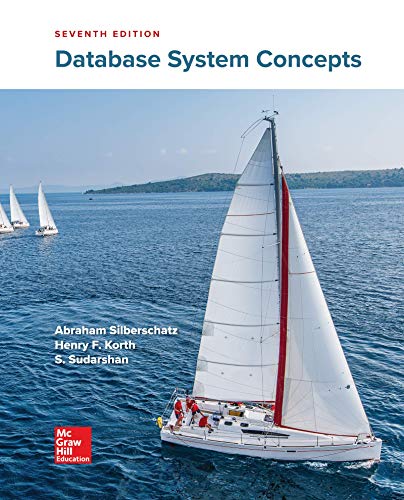
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
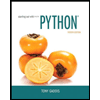
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
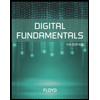
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
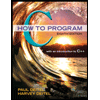
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
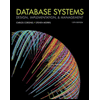
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
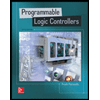
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education