Python: How do I subtract a door + windows (calculated length*width input area) from my 'painted wall', specifically I need second part to remove area from first inputs, THEN recalculate amount of paint needed. Code so far: import math def calculate_paint_needed(length, width, height): length_area = 2 * length * height width_area = 2 * width * height total_wall_area = (length_area + width_area) gallons_needed = total_wall_area / 100.0 return gallons_needed def get_valid_input(prompt): while True: try: value = float(input(prompt)) return value except ValueError: print("Invalid input. Please enter a valid number.") def main(): length = get_valid_input("Enter the length of the room in feet: ") width = get_valid_input("Enter the width of the room in feet: ") height = get_valid_input("Enter the height of the room in feet: ") gallons_needed = calculate_paint_needed(length, width, height) print(f"You will need {math.ceil(gallons_needed)} gallons of paint.") gallons_needed = calculate_paint_needed(length, width, height) print("You will need exactly", gallons_needed, "gallons of paint.") if __name__ == "__main__": main()
Python: How do I subtract a door + windows (calculated length*width input area) from my 'painted wall', specifically I need second part to remove area from first inputs, THEN recalculate amount of paint needed.
Code so far:
import math
def calculate_paint_needed(length, width, height):
length_area = 2 * length * height
width_area = 2 * width * height
total_wall_area = (length_area + width_area)
gallons_needed = total_wall_area / 100.0
return gallons_needed
def get_valid_input(prompt):
while True:
try:
value = float(input(prompt))
return value
except ValueError:
print("Invalid input. Please enter a valid number.")
def main():
length = get_valid_input("Enter the length of the room in feet: ")
width = get_valid_input("Enter the width of the room in feet: ")
height = get_valid_input("Enter the height of the room in feet: ")
gallons_needed = calculate_paint_needed(length, width, height)
print(f"You will need {math.ceil(gallons_needed)} gallons of paint.")
gallons_needed = calculate_paint_needed(length, width, height)
print("You will need exactly", gallons_needed, "gallons of paint.")
if __name__ == "__main__":
main()

Step by step
Solved in 4 steps with 2 images

Last question: How do I make sure the doors portion require an integer greater than one? And windows to have 0 at the same time.
Same code:
import math
def calculate_paint_needed(length, width, height, doors, windows):
# Calculate total wall area
total_wall_area = 2 * (length + width) * height
# Calculate total area of doors and windows
total_door_area = sum(door["length"] * door["width"] for door in doors)
print("Area of door: ",total_door_area)
total_window_area = sum(window["length"] * window["width"] for window in windows)
print("Area of Window: ",total_window_area)
# Subtract total door and window area from total wall area
total_wall_area -= total_door_area + total_window_area
gallons_needed = total_wall_area / 100.0
return gallons_needed
def get_valid_input(prompt):
while True:
try:
value = float(input(prompt))
return value
except ValueError:
print("Invalid input. Please enter a valid number.")
def main():
length = get_valid_input("Enter the length of the room in feet: ")
width = get_valid_input("Enter the width of the room in feet: ")
height = get_valid_input("Enter the height of the room in feet: ")
num_doors = int(input("Enter the number of doors: "))
doors = [{"length": get_valid_input(f"Enter the length of door {i+1} in feet: "),
"width": get_valid_input(f"Enter the width of door {i+1} in feet: ")}
for i in range(num_doors)]
num_windows = int(input("Enter the number of windows: "))
windows = [{"length": get_valid_input(f"Enter the length of window {i+1} in feet: "),
"width": get_valid_input(f"Enter the width of window {i+1} in feet: ")}
for i in range(num_windows)]
gallons_needed = calculate_paint_needed(length, width, height, doors, windows)
print(f"You will need {math.ceil(gallons_needed)} gallons of paint.")
if __name__ == "__main__":
main()
Need help in python getting number adding amount of doors/windows, calculating area of each individual door/window (length*width), then subtracting door/window area from paint needed for room!
My code so far (really need help with changing total door/window area to adding individual and separate ones):
import math
def calculate_paint_needed(length, width, height, door_area, window_area):
length_area = 2 * length * height
width_area = 2 * width * height
total_wall_area = length_area + width_area
total_wall_area -= door_area + window_area
gallons_needed = total_wall_area / 100.0
return gallons_needed
def get_valid_input(prompt):
while True:
try:
value = float(input(prompt))
return value
except ValueError:
print("Invalid input. Please enter a valid number.")
def main():
length = get_valid_input("Enter the length of the room in feet: ")
width = get_valid_input("Enter the width of the room in feet: ")
height = get_valid_input("Enter the height of the room in feet: ")
door_area = get_valid_input("Enter the total area of doors in square feet: ")
window_area = get_valid_input("Enter the total area of windows in square feet: ")
gallons_needed = calculate_paint_needed(length, width, height, door_area, window_area)
print(f"You will need {math.ceil(gallons_needed)} gallons of paint.")
if __name__ == "__main__":
main()
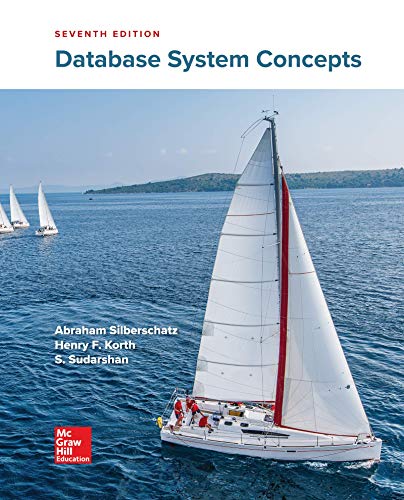
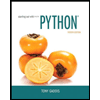
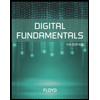
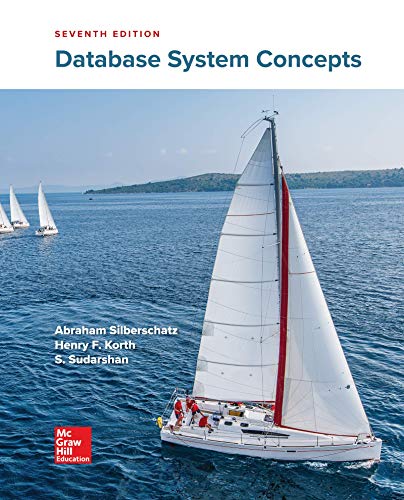
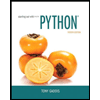
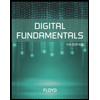
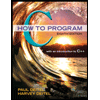
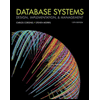
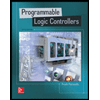