we want to map out a garden in a coordinate plane using integers. O will represent an empty space and 1 will represent a flower. For example, Kevin's garden will look like the following: 0001 0100 0001 In java Use a 2D array to store a garden (in this case, hardcode the flowers) Create a method called printGarden that takes a 2D array as an argument and outputs the garden in a grid/coordinate plane Using: public static void printGarden(int[][] garden) for the method also, use the following code to print the garden using the printGarden method: for (int row=0; row
we want to map out a garden in a coordinate plane using integers. O will represent an empty space and 1 will represent a flower. For example, Kevin's garden will look like the following: 0001 0100 0001 In java Use a 2D array to store a garden (in this case, hardcode the flowers) Create a method called printGarden that takes a 2D array as an argument and outputs the garden in a grid/coordinate plane Using: public static void printGarden(int[][] garden) for the method also, use the following code to print the garden using the printGarden method: for (int row=0; row
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![**Mapping a Garden Using a 2D Array in Java**
In this exercise, we will map out a garden using a coordinate plane and Java. Each point in the plane will hold an integer: '0' will represent an empty space, and '1' will represent a flower. For instance, a sample garden might look like this:
```
0 0 0 1
0 1 0 0
0 0 0 1
```
We'll use a 2D array to store this garden and display it using Java methods.
### Step 1: Display the Garden
Create a method called `printGarden` that takes a 2D array and prints the garden in a grid format.
```java
public static void printGarden(int[][] garden) {
for (int row=0; row < garden.length; row++) {
for (int col = 0; col < garden[row].length; col++) {
// Printing logic for each cell
}
}
}
```
### Step 2: Add a Flower
Implement `addFlower` to add a flower at a specific position.
```java
public static void addFlower(int[][] garden, int row, int column) {
// Add a flower to the specified location
}
```
Use this method to place a flower at row 0, column 1, then display the updated garden with `printGarden`.
### Step 3: Count Flowers in a Column
To determine how many flowers exist in a specific column, use the following method:
```java
public static int countFlowersColumn(int[][] garden, int column) {
// Logic to count flowers in the specified column
}
```
For example, executing `countFlowersColumn(garden, 1)` should return `2`.
### Step 4: Count Flowers in a Row
To determine how many flowers exist in a specific row, implement:
```java
public static int countFlowersRow(int[][] garden, int row) {
// Logic to count flowers in the specified row
}
```
Executing `countFlowersRow(garden, 1)` will return `1`.
### Sample Output
The output will display the garden grid and the count of flowers in specified rows and columns:
```
0 0 0 1
0 1 0 0
0 0 0 1
Flowers in Col 1: 2
Flowers in Row](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4eaca33a-bcfa-4336-aecf-f320ab80f63b%2F5bd20ebb-8ef9-48cd-9e83-d0066734cb79%2Fdd42e3_processed.png&w=3840&q=75)
Transcribed Image Text:**Mapping a Garden Using a 2D Array in Java**
In this exercise, we will map out a garden using a coordinate plane and Java. Each point in the plane will hold an integer: '0' will represent an empty space, and '1' will represent a flower. For instance, a sample garden might look like this:
```
0 0 0 1
0 1 0 0
0 0 0 1
```
We'll use a 2D array to store this garden and display it using Java methods.
### Step 1: Display the Garden
Create a method called `printGarden` that takes a 2D array and prints the garden in a grid format.
```java
public static void printGarden(int[][] garden) {
for (int row=0; row < garden.length; row++) {
for (int col = 0; col < garden[row].length; col++) {
// Printing logic for each cell
}
}
}
```
### Step 2: Add a Flower
Implement `addFlower` to add a flower at a specific position.
```java
public static void addFlower(int[][] garden, int row, int column) {
// Add a flower to the specified location
}
```
Use this method to place a flower at row 0, column 1, then display the updated garden with `printGarden`.
### Step 3: Count Flowers in a Column
To determine how many flowers exist in a specific column, use the following method:
```java
public static int countFlowersColumn(int[][] garden, int column) {
// Logic to count flowers in the specified column
}
```
For example, executing `countFlowersColumn(garden, 1)` should return `2`.
### Step 4: Count Flowers in a Row
To determine how many flowers exist in a specific row, implement:
```java
public static int countFlowersRow(int[][] garden, int row) {
// Logic to count flowers in the specified row
}
```
Executing `countFlowersRow(garden, 1)` will return `1`.
### Sample Output
The output will display the garden grid and the count of flowers in specified rows and columns:
```
0 0 0 1
0 1 0 0
0 0 0 1
Flowers in Col 1: 2
Flowers in Row
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
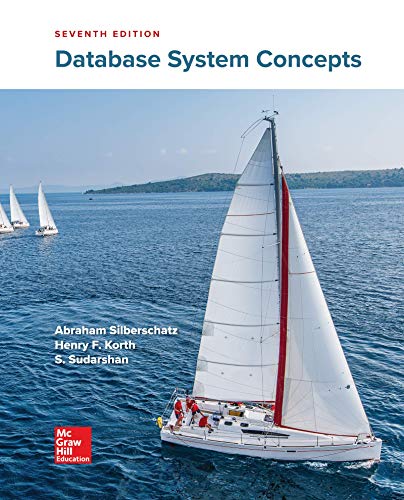
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
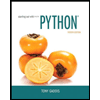
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
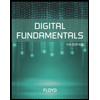
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
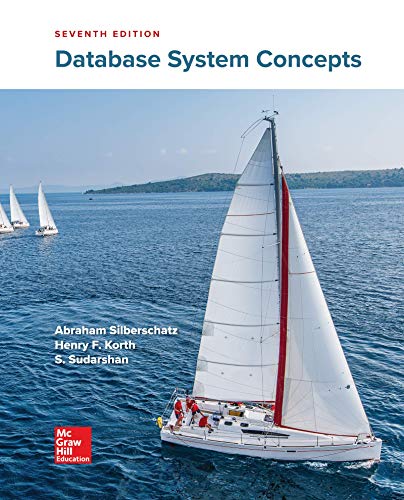
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
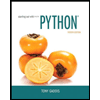
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
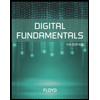
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
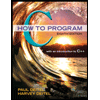
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
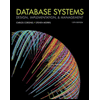
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
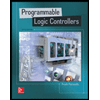
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education