Topics: Functions, Dictionary, I/O Problem description: Write a python application to simulate an online shopping system, namely "Shoppers Stop". The purpose of this assignment is to gain experience in Python dictionary structure and create basic Python function. Design solution: The program will have a list of all products sold on Shoppers Stop which is provided in the template file. Each product consists only one String of comma-separated data: product_id, category, description, cost, and average customer rating. A customer would like to purchase some products by first input the category name, then asked whether a filter should be applied based on minimum customer rating, if so, a list of filtered products is printed. The user will then enter the product_id in that category. If the user input an invalid category name, the program should provide a list of valid category names and ask the user input the category name again. The user can stop shopping by input "checkout", which trigger the checkout() function that computes the total cost of the products in the cart. A customer will be promoted the following messages while shopping at Shoppers Stop: 1. Please input a category name or input 'checkout' to quit: 1) if user inputs 'checkout' go to 4. 2) else if user inputs a valid category name go to 2. 3) otherwise, ask the user again to "Please input a category name or input 'checkout' to quit:" 2. The user is asked if he would like to see a list of filtered products based on customer rating, for the chosen category. 1) if yes, the minimum rating is retrieved from user 2) else, go to 3. 3. Display all the products in the specified category, and inside the entered range (if applicable) and ask the user "Please input product ID or type any key to return" 1) if user input a valid product ID, add the product ID to the cart (i.e. a list of product ID purchased) 2) else go back to 1. 4. Display all the purchased products with their information and the total cost of these products. Notes: • You will need to build two dictionaries before Step 1: o dict_ID: key is the product ID, value is a list of product's category, description, and cost. o dict_category: key is the category name, value is a list of product IDs. • The cart should be implemented as a list of product ID. • I f user chooses to use the customer rating, the product ID with minimum ratings will be saved in another list.
Topics: Functions, Dictionary, I/O Problem description: Write a python application to simulate an online shopping system, namely "Shoppers Stop". The purpose of this assignment is to gain experience in Python dictionary structure and create basic Python function. Design solution: The program will have a list of all products sold on Shoppers Stop which is provided in the template file. Each product consists only one String of comma-separated data: product_id, category, description, cost, and average customer rating. A customer would like to purchase some products by first input the category name, then asked whether a filter should be applied based on minimum customer rating, if so, a list of filtered products is printed. The user will then enter the product_id in that category. If the user input an invalid category name, the program should provide a list of valid category names and ask the user input the category name again. The user can stop shopping by input "checkout", which trigger the checkout() function that computes the total cost of the products in the cart. A customer will be promoted the following messages while shopping at Shoppers Stop: 1. Please input a category name or input 'checkout' to quit: 1) if user inputs 'checkout' go to 4. 2) else if user inputs a valid category name go to 2. 3) otherwise, ask the user again to "Please input a category name or input 'checkout' to quit:" 2. The user is asked if he would like to see a list of filtered products based on customer rating, for the chosen category. 1) if yes, the minimum rating is retrieved from user 2) else, go to 3. 3. Display all the products in the specified category, and inside the entered range (if applicable) and ask the user "Please input product ID or type any key to return" 1) if user input a valid product ID, add the product ID to the cart (i.e. a list of product ID purchased) 2) else go back to 1. 4. Display all the purchased products with their information and the total cost of these products. Notes: • You will need to build two dictionaries before Step 1: o dict_ID: key is the product ID, value is a list of product's category, description, and cost. o dict_category: key is the category name, value is a list of product IDs. • The cart should be implemented as a list of product ID. • I f user chooses to use the customer rating, the product ID with minimum ratings will be saved in another list.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please make sure the code follows sample output highlighted in light blue

Transcribed Image Text:Topics: Functions, Dictionary, I/0
Problem description: Write a python application to simulate an online shopping system, namely
"Shoppers Stop". The purpose of this assignment is to gain experience in Python dictionary structure
and create basic Python function.
Design solution: The program will have a list of all products sold on Shoppers Stop which is provided
in the template file. Each product consists only one String of comma-separated data: product_id,
category, description, cost, and average customer rating. A customer would like to purchase some
products by first input the category name, then asked whether a filter should be applied based on
minimum customer rating, if so, a list of filtered products is printed. The user will then enter the
product_id in that category. If the user input an invalid category name, the program should provide a
list of valid category names and ask the user input the category name again. The user can stop
shopping by input "checkout", which trigger the checkout() function that computes the total cost of
the products in the cart. A customer will be promoted the following messages while shopping at
Shoppers Stop:
1. Please input a category name or input 'checkout' to
quit: 1) if user inputs 'checkout' go to 4.
2) else if user inputs a valid category name go to 2.
3) otherwise, ask the user again to "Please input a category name or input 'checkout' to quit:"
2. The user is asked if he would like to see a list of filtered products based on customer
rating, for the chosen category.
1) if yes, the minimum rating is retrieved from user
2) else, go to 3.
3. Display all the products in the specified category, and inside the entered range (if
applicable) and ask the user "Please input product ID or type any key to return"
1) if user input a valid product ID, add the product ID to the cart (i.e. a list of product ID purchased)
2) else go back to 1.
4. Display all the purchased products with their information and the total cost of these products.
Notes:
• You will need to build two dictionaries before Step 1:
o dict_ID: key is the product ID, value is a list of product's category,
description, and cost. o dict_category: key is the category name, value is a list
of product IDs.
The cart should be implemented as a list of product ID.
• f user chooses to use the customer rating, the product ID with minimum ratings will be
saved in another list.
![The program needs to be case sensitive. Customer can enter the name that can have
all small letter but the program should still be able to find the categories and product
ID in the corresponding dictionary.
• You need to use the product list in the template file, and implement two build dictionary
functions and the checkout function. All other code should be implemented in the main
function.
• To receive full credits, you will need to figure out to generate the very similar input/output as
shown in two provided samples.
Sample I/0 1:
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing', 'food]
Please input a category name or input 'checkout' to quit: book
Would you like to see products in this category filtered by minimum customer ratings (0-5)? Y/N: Y
Please enter the minimum rating: 4
Product ID: BO04 Information: ['book', 'Grant', ' 22.50', ' 4.2']
Please input product ID or type any key to return:
BO04 BO04 added to cart
Welcome to shopping at Shoppers Stop!
Please input a category name or input 'checkout' to quit: checkout
Thanks for shopping at Shoppers Stop. You purchased the following
product(s):
BO04 ("book', 'Grant', '22.50', ' 4.2']
The amount is: $ 22.5
Sample I/0 2:
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing', 'food']
Please input a category name or input 'checkout' to quit: book
Would you like to see products in this category filtered by minimum customer ratings (0-5)? Y/N: Y
Please enter the minimum rating: 4
Product ID: BO04 Information: ['book', 'Grant', ' 22.50', ' 4.2']
Please input product ID or type any key to return:
b004 BO04 added to cart
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing', 'food']
Please input a category name or input 'checkout' to quit: clothing
Would you like to see products in this category filtered by minimum customer ratings (0-5)? Y/N: Y
Please enter the minimum rating: 3.7
Product ID: CO04 Information: ['clothing', 'Wool Hat', ' 14.00', ' 4.5')
Product ID: CO05 Information: ['clothing', 'Wrangler Jeans', ' 24.50', ' 4.1']
Product ID: CO06 Information: ['clothing', 'Nike T-shirt',' 19.00','5')
Product ID: CO08 Information: ['clothing', 'North Face Fleece Jacket', ' 89.95', ' 4.3']
Please input product ID or type any key to return: c008
Co08 added to cart:
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing',
'food')
Please input a category name or input 'checkout' to quit: checkout
Thanks for shopping at Shoppers Stop. You purchased the following
product(s):
b004 ['book', 'Grant', ' 22.50', ' 4.2']
c008 ['clothing', 'North Face Fleece Jacket', ' 89.95','
4.3'] The amount is: $ 112.45](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9eb2eb60-aea6-4c2b-bdb3-b0b3ca45de12%2Fa49d5599-0d52-46ab-be0d-abc2065cd436%2Fnm520tm_processed.png&w=3840&q=75)
Transcribed Image Text:The program needs to be case sensitive. Customer can enter the name that can have
all small letter but the program should still be able to find the categories and product
ID in the corresponding dictionary.
• You need to use the product list in the template file, and implement two build dictionary
functions and the checkout function. All other code should be implemented in the main
function.
• To receive full credits, you will need to figure out to generate the very similar input/output as
shown in two provided samples.
Sample I/0 1:
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing', 'food]
Please input a category name or input 'checkout' to quit: book
Would you like to see products in this category filtered by minimum customer ratings (0-5)? Y/N: Y
Please enter the minimum rating: 4
Product ID: BO04 Information: ['book', 'Grant', ' 22.50', ' 4.2']
Please input product ID or type any key to return:
BO04 BO04 added to cart
Welcome to shopping at Shoppers Stop!
Please input a category name or input 'checkout' to quit: checkout
Thanks for shopping at Shoppers Stop. You purchased the following
product(s):
BO04 ("book', 'Grant', '22.50', ' 4.2']
The amount is: $ 22.5
Sample I/0 2:
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing', 'food']
Please input a category name or input 'checkout' to quit: book
Would you like to see products in this category filtered by minimum customer ratings (0-5)? Y/N: Y
Please enter the minimum rating: 4
Product ID: BO04 Information: ['book', 'Grant', ' 22.50', ' 4.2']
Please input product ID or type any key to return:
b004 BO04 added to cart
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing', 'food']
Please input a category name or input 'checkout' to quit: clothing
Would you like to see products in this category filtered by minimum customer ratings (0-5)? Y/N: Y
Please enter the minimum rating: 3.7
Product ID: CO04 Information: ['clothing', 'Wool Hat', ' 14.00', ' 4.5')
Product ID: CO05 Information: ['clothing', 'Wrangler Jeans', ' 24.50', ' 4.1']
Product ID: CO06 Information: ['clothing', 'Nike T-shirt',' 19.00','5')
Product ID: CO08 Information: ['clothing', 'North Face Fleece Jacket', ' 89.95', ' 4.3']
Please input product ID or type any key to return: c008
Co08 added to cart:
Welcome to shopping at Shoppers Stop!
We sell products in the following categories: ['book', 'clothing',
'food')
Please input a category name or input 'checkout' to quit: checkout
Thanks for shopping at Shoppers Stop. You purchased the following
product(s):
b004 ['book', 'Grant', ' 22.50', ' 4.2']
c008 ['clothing', 'North Face Fleece Jacket', ' 89.95','
4.3'] The amount is: $ 112.45
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
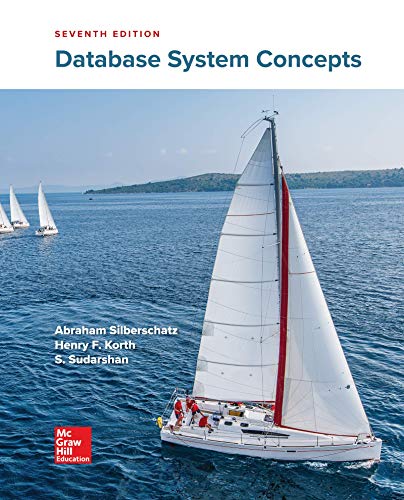
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
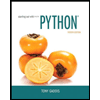
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
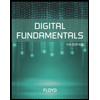
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
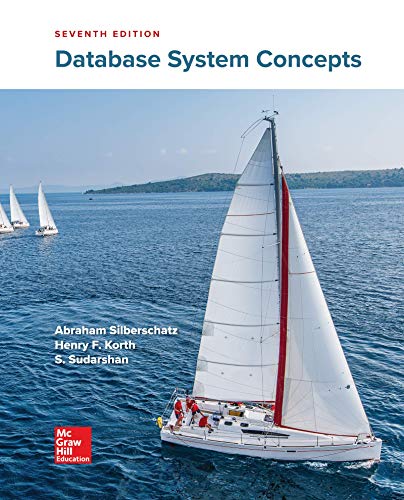
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
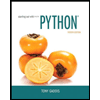
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
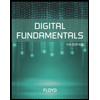
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
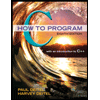
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
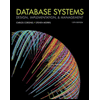
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
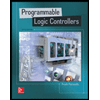
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education