In [ ]: Exercise 1b Write code to calculate and print the quantities from the data sheet. For the momenta pli and p2i, the code is given. For the other quantities, uncomment the respective code lines and add your own code. You must use the given variable names; do not change their case (e.g. from Kli to kli). For full credit: • Use "f-strings" to print your calculated values. • Make sure each quantity is printed with its correct unit. Follow the example for pli. • Verify that your code produces sensible results. pli ml*vli p2i = m2*v2i print (f'Momentum of m1 before collision: print (f'Momentum of m2 before collision: #pi = #mtot = # pf = #p_ratio= # print () #print() # print () # print () # Kli = #K2i = #print() #print() #Ki = #Kf = #K_ratio= # print () #print() # print () # Momentum of m1 before collision. # Momentum of m2 before collision {pli: eP) kg m/s') {p2i: eP} kg-m/s') #YOUR CODE HERE raise Not ImplementedError() # Total momentum before collision # Combined mass # Total momentum after collision # Ratio of total momentum after/before collision #Print your calculated values (one per print statement. Use f-strings) # Kinetic energy of m1 before collision #Kinetic energy of m2 before collision # Total kinetic energy before collision #Total kinetic energy after collision # Ratio of total kinetic energy after/before collision
In [ ]: Exercise 1b Write code to calculate and print the quantities from the data sheet. For the momenta pli and p2i, the code is given. For the other quantities, uncomment the respective code lines and add your own code. You must use the given variable names; do not change their case (e.g. from Kli to kli). For full credit: • Use "f-strings" to print your calculated values. • Make sure each quantity is printed with its correct unit. Follow the example for pli. • Verify that your code produces sensible results. pli ml*vli p2i = m2*v2i print (f'Momentum of m1 before collision: print (f'Momentum of m2 before collision: #pi = #mtot = # pf = #p_ratio= # print () #print() # print () # print () # Kli = #K2i = #print() #print() #Ki = #Kf = #K_ratio= # print () #print() # print () # Momentum of m1 before collision. # Momentum of m2 before collision {pli: eP) kg m/s') {p2i: eP} kg-m/s') #YOUR CODE HERE raise Not ImplementedError() # Total momentum before collision # Combined mass # Total momentum after collision # Ratio of total momentum after/before collision #Print your calculated values (one per print statement. Use f-strings) # Kinetic energy of m1 before collision #Kinetic energy of m2 before collision # Total kinetic energy before collision #Total kinetic energy after collision # Ratio of total kinetic energy after/before collision
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Exercise 1b**
**Objective:** Write code to calculate and print the quantities from the data sheet. For the momenta \( \texttt{p1i} \) and \( \texttt{p2i} \), the code is given. For the other quantities, uncomment the respective code lines and add your own code. You must use the given variable names; do not change their case (e.g., from \texttt{K1i} to \texttt{k1i}).
**For full credit:**
- Use "f-strings" to print your calculated values.
- Make sure each quantity is printed with its correct unit. Follow the example for \texttt{p1i}.
- Verify that your code produces sensible results.
```python
p1i = m1*v1i # Momentum of m1 before collision
p2i = m2*v2i # Momentum of m2 before collision
print(f'Momentum of m1 before collision: {p1i:eP} kg·m/s')
print(f'Momentum of m2 before collision: {p2i:eP} kg·m/s')
# pi = # Total momentum before collision
# mtot = # Combined mass
# pf = # Total momentum after collision
# p_ratio = # Ratio of total momentum after/before collision
# Print your calculated values (one per print statement. Use f-strings)
# K1i = # Kinetic energy of m1 before collision
# K2i = # Kinetic energy of m2 before collision
# print() #
# print() #
# Ki = # Total kinetic energy before collision
# Kf = # Total kinetic energy after collision
# K_ratio = # Ratio of total kinetic energy after/before collision
# print() #
# print() #
# print() #
# YOUR CODE HERE
raise NotImplementedError()
```
**Instructions:**
1. **Calculate Required Quantities:**
- Total initial momentum (\( \texttt{pi} \)).
- Combined mass (\( \texttt{mtot} \)).
- Total final momentum (\( \texttt{pf} \)).
- Momentum ratio (\( \texttt{p\_ratio} \)).
2. **Compute Kinetic Energies:**
- Initial kinetic energy of
Expert Solution

Step 1: Algorithm:
- Start
- Define the masses (m1 and m2) and initial velocities (v1i and v2i) of two objects.
- Calculate the initial momenta (p1i and p2i) by multiplying the mass and initial velocity for each object.
- Calculate the total momentum before the collision (mtot) by summing up the individual momenta.
- Calculate the combined mass (mf) of the two objects.
- Calculate the final momenta (p1f and p2f) after the collision, assuming an elastic collision where momentum is conserved (they remain the same as initial momenta).
- Calculate the total momentum after the collision (pftot) by summing up the final momenta.
- Calculate the ratio of total momentum after the collision to total momentum before the collision (p_ratio).
- Calculate the initial kinetic energies (K1i and K2i) for each object using the kinetic energy formula.
- Calculate the total initial kinetic energy (Ki) by summing up the individual kinetic energies.
- Assuming an elastic collision, calculate the total final kinetic energy (Kf), which remains the same as the total initial kinetic energy.
- Calculate the ratio of total kinetic energy after the collision to total kinetic energy before the collision (K_ratio).
- Print the calculated values for momenta, total momenta, combined mass, kinetic energies, and the respective ratios.
- End.
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
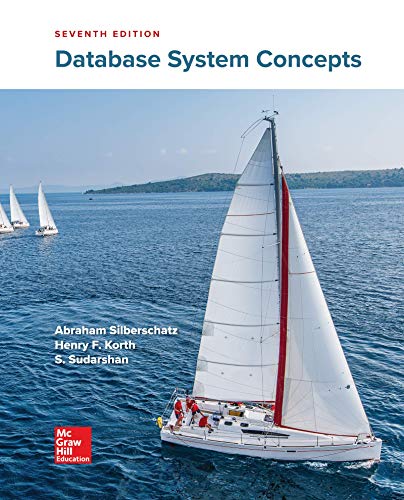
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
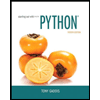
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
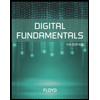
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
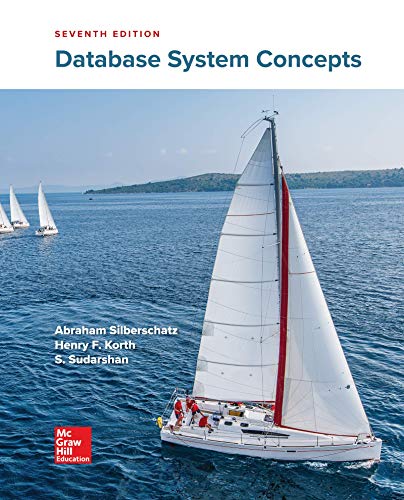
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
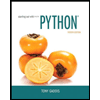
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
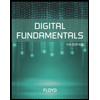
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
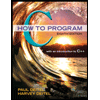
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
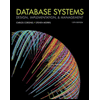
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
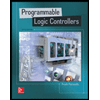
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education