This is my MIPS Assembly Program: its supposed to define two integer arrays that are pre-sorted and the same size (e.g., [3, 7, 9, 11, 15, 21] and [1, 4, 6, 14, 18, 19]) and a function merge that takes the two arrays and their sizes as inputs and makes a single array of twice the size of either input array that contains the elements of both arrays in ascending order. In the example arrays given, then output would be [1, 3, 4, 6, 7, 9, 11, 14, 15, 18, 19, 21]. Have your 'main' function (not the merge function) print out the final array using a comma as a delimiter. .data array1: .word 3, 7, 9, 11, 15, 21 # First sorted array array2: .word 1, 4, 6, 14, 18, 19 # Second sorted array size: .word 6 # Size of each array merged: .space 48 # Space for merged array (2 * 6 * 4 bytes) output: .asciiz "Merged array: " comma: .asciiz ", " .text.globl main main: # Load size of the arrays la $t0, size lw $t1, 0($t0) # $t1 = size of each array (6) # Call merge function la $a0, array1 # $a0 = first array la $a1, array2 # $a1 = second array la $a2, merged # $a2 = merged array move $a3, $t1 # $a3 = size of arrays jal merge # Call merge function # Display merged array li $v0, 4 # Display string syscall la $a0, output # Load address of output string syscall li $t2, 0 # Index for merged arraydisplay_loop: lw $a0, 0($a2) # Load value from merged array li $v0, 1 # Display integer syscall syscall addi $t2, $t2, 1 # Increment index addi $a2, $a2, 4 # Move to the next element in merged array # Check if it's the last element to avoid trailing comma bge $t2, $a3, end_display li $v0, 4 # Display string syscall la $a0, comma # Load address of comma string syscall j display_loop # Continue displaying end_display: # Display a newline li $v0, 11 # Display char syscall li $a0, 10 # ASCII newline syscall li $v0, 10 # Exit syscall syscall merge: # Inputs: $a0 = array1, $a1 = array2, $a2 = merged, $a3 = size move $t0, $zero # Index for array1 move $t1, $zero # Index for array2 move $t2, $zero # Index for merged array merge_loop: # Check if we've finished merging bge $t0, $a3, copy_array2 bge $t1, $a3, copy_array1 # Load elements from both arrays lw $t4, 0($a0) # $t4 = array1[$t0] lw $t5, 0($a1) # $t5 = array2[$t1] # Compare and merge blt $t4, $t5, insert_array1 j insert_array2 insert_array1: sw $t4, 0($a2) # merged[$t2] = array1[$t0] addi $t0, $t0, 1 # Increment index for array1 j increment_index insert_array2: sw $t5, 0($a2) # merged[$t2] = array2[$t1] addi $t1, $t1, 1 # Increment index for array2 increment_index: addi $t2, $t2, 1 # Increment index for merged array addi $a2, $a2, 4 # Move to the next position in merged array j merge_loop copy_array1: # Copy remaining elements from array1 bge $t0, $a3, merge_end # If finished, jump to end lw $t4, 0($a0) # Load from array1 sw $t4, 0($a2) # Store in merged addi $t0, $t0, 1 # Increment array1 index addi $t2, $t2, 1 # Increment merged index addi $a2, $a2, 4 # Move to the next position in merged array j copy_array1 copy_array2: # Copy remaining elements from array2 bge $t1, $a3, merge_end # If finished, jump to end lw $t5, 0($a1) # Load from array2 sw $t5, 0($a2) # Store in merged addi $t1, $t1, 1 # Increment array2 index addi $t2, $t2, 1 # Increment merged index addi $a2, $a2, 4 # Move to the next position in merged array j copy_array2 merge_end: jr $ra # Return from merge #end of program =========================================================================================== However I am getting this output "Merged array: 1735550285, 1629512805, 2036429426, 738205754, 32, 0" which is wrong please help debug
This is my MIPS Assembly Program: its supposed to define two integer arrays that are pre-sorted and the same size (e.g., [3, 7, 9, 11, 15, 21] and [1, 4, 6, 14, 18, 19]) and a function merge that takes the two arrays and their sizes as inputs and makes a single array of twice the size of either input array that contains the elements of both arrays in ascending order. In the example arrays given, then output would be [1, 3, 4, 6, 7, 9, 11, 14, 15, 18, 19, 21]. Have your 'main' function (not the merge function) print out the final array using a comma as a delimiter.
.data
array1: .word 3, 7, 9, 11, 15, 21 # First sorted array
array2: .word 1, 4, 6, 14, 18, 19 # Second sorted array
size: .word 6 # Size of each array
merged: .space 48 # Space for merged array (2 * 6 * 4 bytes)
output: .asciiz "Merged array: "
comma: .asciiz ", "
.text
.globl main
main:
# Load size of the arrays
la $t0, size
lw $t1, 0($t0) # $t1 = size of each array (6)
# Call merge function
la $a0, array1 # $a0 = first array
la $a1, array2 # $a1 = second array
la $a2, merged # $a2 = merged array
move $a3, $t1 # $a3 = size of arrays
jal merge # Call merge function
# Display merged array
li $v0, 4 # Display string syscall
la $a0, output # Load address of output string
syscall
li $t2, 0 # Index for merged array
display_loop:
lw $a0, 0($a2) # Load value from merged array
li $v0, 1 # Display integer syscall
syscall
addi $t2, $t2, 1 # Increment index
addi $a2, $a2, 4 # Move to the next element in merged array
# Check if it's the last element to avoid trailing comma
bge $t2, $a3, end_display
li $v0, 4 # Display string syscall
la $a0, comma # Load address of comma string
syscall
j display_loop # Continue displaying
end_display:
# Display a newline
li $v0, 11 # Display char syscall
li $a0, 10 # ASCII newline
syscall
li $v0, 10 # Exit syscall
syscall
merge:
# Inputs: $a0 = array1, $a1 = array2, $a2 = merged, $a3 = size
move $t0, $zero # Index for array1
move $t1, $zero # Index for array2
move $t2, $zero # Index for merged array
merge_loop:
# Check if we've finished merging
bge $t0, $a3, copy_array2
bge $t1, $a3, copy_array1
# Load elements from both arrays
lw $t4, 0($a0) # $t4 = array1[$t0]
lw $t5, 0($a1) # $t5 = array2[$t1]
# Compare and merge
blt $t4, $t5, insert_array1
j insert_array2
insert_array1:
sw $t4, 0($a2) # merged[$t2] = array1[$t0]
addi $t0, $t0, 1 # Increment index for array1
j increment_index
insert_array2:
sw $t5, 0($a2) # merged[$t2] = array2[$t1]
addi $t1, $t1, 1 # Increment index for array2
increment_index:
addi $t2, $t2, 1 # Increment index for merged array
addi $a2, $a2, 4 # Move to the next position in merged array
j merge_loop
copy_array1:
# Copy remaining elements from array1
bge $t0, $a3, merge_end # If finished, jump to end
lw $t4, 0($a0) # Load from array1
sw $t4, 0($a2) # Store in merged
addi $t0, $t0, 1 # Increment array1 index
addi $t2, $t2, 1 # Increment merged index
addi $a2, $a2, 4 # Move to the next position in merged array
j copy_array1
copy_array2:
# Copy remaining elements from array2
bge $t1, $a3, merge_end # If finished, jump to end
lw $t5, 0($a1) # Load from array2
sw $t5, 0($a2) # Store in merged
addi $t1, $t1, 1 # Increment array2 index
addi $t2, $t2, 1 # Increment merged index
addi $a2, $a2, 4 # Move to the next position in merged array
j copy_array2
merge_end:
jr $ra # Return from merge
#end of program
===========================================================================================
However I am getting this output "Merged array: 1735550285, 1629512805, 2036429426, 738205754, 32, 0"
which is wrong
please help debug
Unlock instant AI solutions
Tap the button
to generate a solution
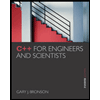
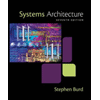
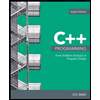
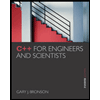
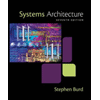
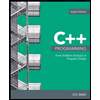
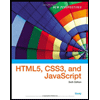
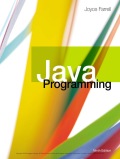
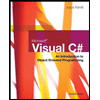