Lab1 PROGRAMMING TASK 7.3 A program is needed to ask the user to input the price of products they have bought. Calculate and output the total cost they spent. Getting started 1 What are the steps required to find the total? For example, initialising the total to 0 will be the first step. CONTINUED 2 You will need to work out how to continue asking for the price of products until they do not have any more. Have a look at some of the previous algorithms in this chapter that perform similar actions to this. What did they use? How did they limit the number that could be entered? Practice 1 2 3 REPEAT TOTAI TOTAL +0. Create a structure diagram for the problem. Write an algorithm to ask users to repeatedly input the price of products they have bought until there are no more to enter. Amend the algorithm to total the cost of all the products and output this at the end of the algorithm. Challenge 1 2 Amend the algorithm to also count how many items they entered. You can look ahead to the next section or work out how to count them yourself. Now you have the total and the quantity, amend your program to work out the average cost. Price o WHILE Price 40. OUTPUT "Input the price of product they bought" 8 Lab2 1 Difference ifferences betwe ore about arrays PROGRAMMING TASK 7.4 A program needs to ask the user to input the weight of parcels being posted. It needs to count how many parcels are less than 1 kg, how many are between 1 kg and 2kg, and how many are above 2 kg. Getting started 1 2 Recap how to count in an algorithm. What are the different pieces of code you need? For example, initialise the count to start at 0. You will need to use selection to compare the inputs with the weights. Recap the use of selection and the format, including the differences between the operators >, >=, < and <=. Practice owcharts & Tra Pre- 1 Differ P swer T ile Answer != ' Answer inpu 1 Draw a structure diagram for the problem. 2 3 4 Write an algorithm to read in the weights of the parcels until the user has finished. Amend the algorithm to count and output how many parcels are below 1kg. Amend the algorithm to also count and output how many parcels are between 1 and 2kg. 5 Amend the algorithm to also count and output how many parcels are more than 2kg. Challenge The user would like to enter the limits that they are counting themselves when the program starts. For example, counting how many parcels are less than 1.5 kg instead of 1 kg. Amend the program to make this change. START INPUT the weight of panels] AND X +Count=0 Cont2=AN (Count3=0 | [lant3 = [ortze No /OUTPUT County, count: AND Counts/ Yes Fxxx | Cant2= Caft₂+1 x42 3 Count to IF Weight < 1 OUT Put Court Count Count +1 UNTIL finished
Lab1 PROGRAMMING TASK 7.3 A program is needed to ask the user to input the price of products they have bought. Calculate and output the total cost they spent. Getting started 1 What are the steps required to find the total? For example, initialising the total to 0 will be the first step. CONTINUED 2 You will need to work out how to continue asking for the price of products until they do not have any more. Have a look at some of the previous algorithms in this chapter that perform similar actions to this. What did they use? How did they limit the number that could be entered? Practice 1 2 3 REPEAT TOTAI TOTAL +0. Create a structure diagram for the problem. Write an algorithm to ask users to repeatedly input the price of products they have bought until there are no more to enter. Amend the algorithm to total the cost of all the products and output this at the end of the algorithm. Challenge 1 2 Amend the algorithm to also count how many items they entered. You can look ahead to the next section or work out how to count them yourself. Now you have the total and the quantity, amend your program to work out the average cost. Price o WHILE Price 40. OUTPUT "Input the price of product they bought" 8 Lab2 1 Difference ifferences betwe ore about arrays PROGRAMMING TASK 7.4 A program needs to ask the user to input the weight of parcels being posted. It needs to count how many parcels are less than 1 kg, how many are between 1 kg and 2kg, and how many are above 2 kg. Getting started 1 2 Recap how to count in an algorithm. What are the different pieces of code you need? For example, initialise the count to start at 0. You will need to use selection to compare the inputs with the weights. Recap the use of selection and the format, including the differences between the operators >, >=, < and <=. Practice owcharts & Tra Pre- 1 Differ P swer T ile Answer != ' Answer inpu 1 Draw a structure diagram for the problem. 2 3 4 Write an algorithm to read in the weights of the parcels until the user has finished. Amend the algorithm to count and output how many parcels are below 1kg. Amend the algorithm to also count and output how many parcels are between 1 and 2kg. 5 Amend the algorithm to also count and output how many parcels are more than 2kg. Challenge The user would like to enter the limits that they are counting themselves when the program starts. For example, counting how many parcels are less than 1.5 kg instead of 1 kg. Amend the program to make this change. START INPUT the weight of panels] AND X +Count=0 Cont2=AN (Count3=0 | [lant3 = [ortze No /OUTPUT County, count: AND Counts/ Yes Fxxx | Cant2= Caft₂+1 x42 3 Count to IF Weight < 1 OUT Put Court Count Count +1 UNTIL finished
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Lab1
PROGRAMMING TASK 7.3
A program is needed to ask the user to input the price of products they have
bought. Calculate and output the total cost they spent.
Getting started
1
What are the steps required to find the total? For example, initialising the
total to 0 will be the first step.
CONTINUED
2
You will need to work out how to continue asking for the price of products
until they do not have any more. Have a look at some of the previous
algorithms in this chapter that perform similar actions to this. What did
they use? How did they limit the number that could be entered?
Practice
1
2
3
REPEAT
TOTAI TOTAL +0.
Create a structure diagram for the problem.
Write an algorithm to ask users to repeatedly input the price of products
they have bought until there are no more to enter.
Amend the algorithm to total the cost of all the products and output this
at the end of the algorithm.
Challenge
1
2
Amend the algorithm to also count how many items they entered. You can
look ahead to the next section or work out how to count them yourself.
Now you have the total and the quantity, amend your program to work
out the average cost.
Price o
WHILE Price 40.
OUTPUT "Input the price of product
they bought"
8
![Lab2
1 Difference
ifferences betwe
ore about arrays
PROGRAMMING TASK 7.4
A program needs to ask the user to input the weight of parcels being posted.
It needs to count how many parcels are less than 1 kg, how many are between
1 kg and 2kg, and how many are above 2 kg.
Getting started
1
2
Recap how to count in an algorithm. What are the different pieces of code
you need? For example, initialise the count to start at 0.
You will need to use selection to compare the inputs with the weights.
Recap the use of selection and the format, including the differences
between the operators >, >=, < and <=.
Practice
owcharts & Tra
Pre-
1 Differ
P
swer T
ile Answer != '
Answer inpu
1
Draw a structure diagram for the problem.
2
3
4
Write an algorithm to read in the weights of the parcels until the user has
finished.
Amend the algorithm to count and output how many parcels are below 1kg.
Amend the algorithm to also count and output how many parcels are
between 1 and 2kg.
5
Amend the algorithm to also count and output how many parcels are
more than 2kg.
Challenge
The user would like to enter the limits that they are counting themselves when
the program starts. For example, counting how many parcels are less than
1.5 kg instead of 1 kg. Amend the program to make this change.
START
INPUT the weight of panels]
AND
X
+Count=0 Cont2=AN (Count3=0 |
<IF xel flaut Caf
No
TEX> [lant3 = [ortze
No
/OUTPUT County, count: AND Counts/
Yes
Fxxx
| Cant2= Caft₂+1
x42
3 Count to
IF Weight < 1
OUT Put Court
Count Count +1
UNTIL finished](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff3f8fd09-be0c-463a-9f2e-f984b3eac55b%2F202126cb-59be-4d80-9825-a273c089f860%2Fp0crno3_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Lab2
1 Difference
ifferences betwe
ore about arrays
PROGRAMMING TASK 7.4
A program needs to ask the user to input the weight of parcels being posted.
It needs to count how many parcels are less than 1 kg, how many are between
1 kg and 2kg, and how many are above 2 kg.
Getting started
1
2
Recap how to count in an algorithm. What are the different pieces of code
you need? For example, initialise the count to start at 0.
You will need to use selection to compare the inputs with the weights.
Recap the use of selection and the format, including the differences
between the operators >, >=, < and <=.
Practice
owcharts & Tra
Pre-
1 Differ
P
swer T
ile Answer != '
Answer inpu
1
Draw a structure diagram for the problem.
2
3
4
Write an algorithm to read in the weights of the parcels until the user has
finished.
Amend the algorithm to count and output how many parcels are below 1kg.
Amend the algorithm to also count and output how many parcels are
between 1 and 2kg.
5
Amend the algorithm to also count and output how many parcels are
more than 2kg.
Challenge
The user would like to enter the limits that they are counting themselves when
the program starts. For example, counting how many parcels are less than
1.5 kg instead of 1 kg. Amend the program to make this change.
START
INPUT the weight of panels]
AND
X
+Count=0 Cont2=AN (Count3=0 |
<IF xel flaut Caf
No
TEX> [lant3 = [ortze
No
/OUTPUT County, count: AND Counts/
Yes
Fxxx
| Cant2= Caft₂+1
x42
3 Count to
IF Weight < 1
OUT Put Court
Count Count +1
UNTIL finished
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
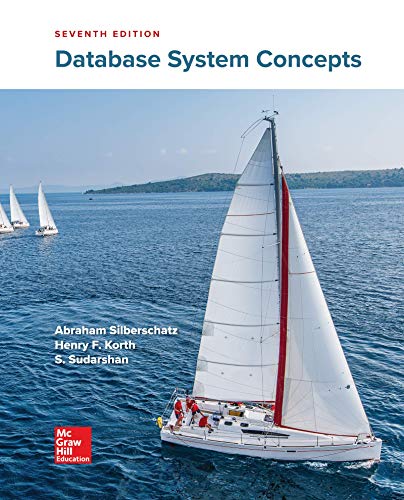
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
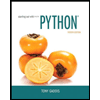
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
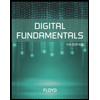
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
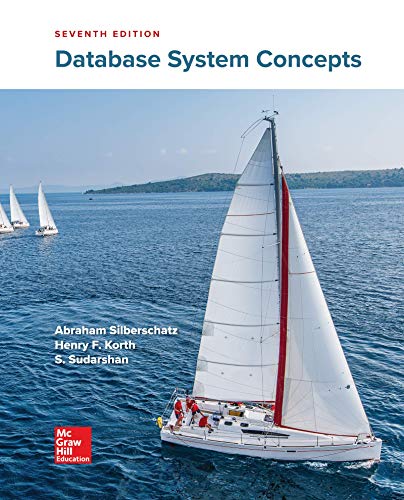
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
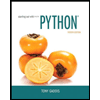
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
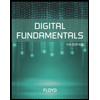
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
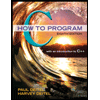
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
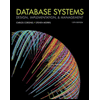
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
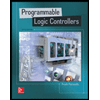
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education