this is basically compueter architecture add main code and functions to randomly populate a .space with 50 Integers, sort and then display them.
this is basically compueter architecture
add main code and functions to randomly populate a .space with 50 Integers, sort and then display them.
-------
# Bubble Sort Example # # void bsort(int v[], const unsigned SIZE); # # int main(void) # { # const unsigned SIZE = 7; # int arr[SIZE] = { 34, 7, 112, 787, 34, 12, 66 }; # # bsort(arr, SIZE); # # return 0; # } # # void bsort(int v[], const unsigned SIZE) # { # for(unsigned pass = 0; pass < SIZE - 1; ++pass) { # for(unsigned index = 0; index < SIZE - pass - 1; ++index) { # if(v[index+1] < v[index]){ # swap(v[index+1] < v[index]); # } # } # } # } # # /* Example of function execution # # index= 0 1 2 3 4 5 6 # --- --- --- --- --- --- --- # pass=0, i=0, v={ 34, 7, 112, 787, 34, 12, 66 } //swap 34 and 7 # pass=0, i=1, v={ 7, 34, 112, 787, 34, 12, 66 } //no swap # pass=0, i=2, v={ 7, 34, 112, 787, 34, 12, 66 } //no swap # pass=0, i=3, v={ 7, 34, 112, 787, 34, 12, 66 } //swap 787 and 34 # pass=0, i=4, v={ 7, 34, 112, 34, 787, 12, 66 } //swap 787 and 12 # pass=0, i=5, v={ 7, 34, 112, 34, 12, 787, 66 } //swap 787 and 66 # pass=0, i=6, v={ 7, 34, 112, 34, 12, 66, 787 } // 787 is sorted # # pass=1, i=0, v={ 7, 34, 112, 34, 12, 66, 787 } //no swap # pass=1, i=1, v={ 7, 34, 112, 34, 12, 66, 787 } //no swap # pass=1, i=2, v={ 7, 34, 112, 34, 12, 66, 787 } //swap 112 and 34 # pass=1, i=3, v={ 7, 34, 34, 112, 12, 66, 787 } //swap 112 and 12 # pass=1, i=4, v={ 7, 34, 34, 12, 112, 66, 787 } //swap 112 and 66 # pass=1, i=5, v={ 7, 34, 34, 12, 66, 112, 787 } // 112, 787 are sorted # # pass=2, i=0, v={ 7, 34, 34, 12, 66, 112, 787 } //no swap # pass=2, i=1, v={ 7, 34, 34, 12, 66, 112, 787 } //no swap # pass=2, i=2, v={ 7, 34, 34, 12, 66, 112, 787 } //swap 34 and 12 # pass=2, i=3, v={ 7, 34, 12, 34, 66, 112, 787 } //no swap # pass=2, i=4, v={ 7, 34, 12, 34, 66, 112, 787 } // 66, 112, 787 are sorted # # pass=3, i=0, v={ 7, 34, 12, 34, 66, 112, 787 } //swap 34 and 12 # pass=3, i=1, v={ 7, 12, 34, 34, 66, 112, 787 } //no swap # pass=3, i=2, v={ 7, 12, 34, 34, 66, 112, 787 } //no swap # pass=3, i=3, v={ 7, 12, 34, 34, 66, 112, 787 } // 34, 66, 112, 787 are sorted # # pass=4, i=0, v={ 7, 12, 34, 34, 66, 112, 787 } //no swap # pass=4, i=1, v={ 7, 12, 34, 34, 66, 112, 787 } //no swap # pass=4, i=2, v={ 7, 12, 34, 34, 66, 112, 787 } // 34, 34, 66, 112, 787 are sorted # # pass=5, i=0, v={ 7, 12, 34, 34, 66, 112, 787 } //no swap # pass=5, i=1, v={ 7, 12, 34, 34, 66, 112, 787 } // 12, 34, 34, 66, 112, 787 are sorted # # pass=6, i=0, v={ 7, 12, 34, 34, 66, 112, 787 } //end of sort # */ .data arr: .word 5, 10, 15, 20, 25, 21, 16, 11, 6, 1 size: .word 10 .text .globl main main: la $a0, arr lw $a1, size jal sort li $v0, 10 syscall sort: addi $sp,$sp, -20 # make room on stack for 5 registers sw $ra, 16($sp) # save $ra on stack sw $s3, 12($sp) # save $s3 on stack sw $s2, 8($sp) # save $s2 on stack sw $s1, 4($sp) # save $s1 on stack sw $s0, 0($sp) # save $s0 on stack move $s2, $a0 # save $a0 into $s2 move $s3, $a1 # save $a1 into $s3 move $s0, $zero # i = 0 for1tst: slt $t0, $s0, $s3 # $t0 = 0 if $s0 < $s3 (i < n) beq $t0, $zero, exit1 # go to exit1 if $s0 == $s3 (i == n) addi $s1, $s0, -1 # j = i – 1 for2tst: slti $t0, $s1, 0 # $t0 = 1 if $s1 < 0 (j < 0) bne $t0, $zero, exit2 # go to exit2 if $s1 < 0 (j < 0) sll $t1, $s1, 2 # $t1 = j * 4 add $t2, $s2, $t1 # $t2 = v + (j * 4) lw $t3, 0($t2) # $t3 = v[j] lw $t4, 4($t2) # $t4 = v[j + 1] slt $t0, $t4, $t3 # $t0 = 0 if $t4 ≥ $t3 beq $t0, $zero, exit2 # go to exit2 if $t4 ≥ $t3 move $a0, $s2 # 1st param of swap is v (old $a0) move $a1, $s1 # 2nd param of swap is j jal swap # call swap procedure addi $s1, $s1, -1 # j –= 1 j for2tst # jump to test of inner loop exit2: addi $s0, $s0, 1 # i += 1 j for1tst # jump to test of outer loop exit1: lw $s0, 0($sp) # restore $s0 from stack lw $s1, 4($sp) # restore $s1 from stack lw $s2, 8($sp) # restore $s2 from stack lw $s3,12($sp) # restore $s3 from stack lw $ra,16($sp) # restore $ra from stack addi $sp,$sp, 20 # restore stack pointer jr $ra # return to calling routine swap: sll $t1, $a1, 2 # $t1 = k * 4 add $t1, $a0, $t1 # $t1 = v+(k*4) # (address of v[k]) lw $t0, 0($t1) # $t0 (temp) = v[k] lw $t2, 4($t1) # $t2 = v[k+1] sw $t2, 0($t1) # v[k] = $t2 (v[k+1]) sw $t0, 4($t1) # v[k+1] = $t0 (temp) jr $ra # return to calling routine
------------

Step by step
Solved in 4 steps with 2 images

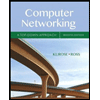
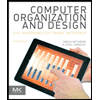
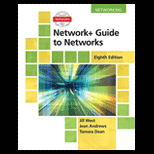
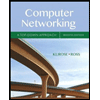
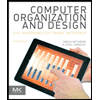
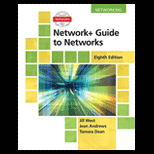
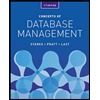
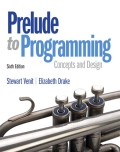
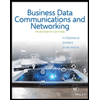