10. Implement Binary search algorithm to search a number in the sorted array of integers given below: int sA[10] = {1,2,3,4,5,6,7,8,9,10} Read input a number from the user and search it in the array.
10. Implement Binary search algorithm to search a number in the sorted array of integers given below: int sA[10] = {1,2,3,4,5,6,7,8,9,10} Read input a number from the user and search it in the array.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++
![### Exercise 10: Implementing a Binary Search Algorithm
**Objective:** Implement a binary search algorithm to locate a number within a given sorted array of integers.
#### Array:
```plaintext
int sA[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
```
#### Task:
Write a program to accept a number input from the user and search for this number within the array using the binary search method.
**Steps to Implement:**
1. **Initialize Parameters:**
- Start with pointers that represent the beginning (`low`), middle (`mid`), and end (`high`) of the array.
2. **Iterative Search:**
- Continuously compare the target number with the middle element of the array.
- If the target number equals the middle element, the search is complete.
- If the target number is less than the middle element, adjust the `high` pointer to `mid - 1`.
- If the target number is greater than the middle element, adjust the `low` pointer to `mid + 1`.
- Repeat until the `low` pointer exceeds the `high` pointer, indicating that the number is not present in the array.
3. **Output Result:**
- If found, return the position of the number in the array.
- If not found, indicate that the number does not exist in the array.
This exercise demonstrates the efficiency of binary search, which significantly reduces the search time in sorted arrays compared to a linear search.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9aa45855-5908-4799-a62b-2520490177e1%2Fff2f78cd-3bab-4796-9094-3cc3bab6e61b%2F4vagjep_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Exercise 10: Implementing a Binary Search Algorithm
**Objective:** Implement a binary search algorithm to locate a number within a given sorted array of integers.
#### Array:
```plaintext
int sA[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
```
#### Task:
Write a program to accept a number input from the user and search for this number within the array using the binary search method.
**Steps to Implement:**
1. **Initialize Parameters:**
- Start with pointers that represent the beginning (`low`), middle (`mid`), and end (`high`) of the array.
2. **Iterative Search:**
- Continuously compare the target number with the middle element of the array.
- If the target number equals the middle element, the search is complete.
- If the target number is less than the middle element, adjust the `high` pointer to `mid - 1`.
- If the target number is greater than the middle element, adjust the `low` pointer to `mid + 1`.
- Repeat until the `low` pointer exceeds the `high` pointer, indicating that the number is not present in the array.
3. **Output Result:**
- If found, return the position of the number in the array.
- If not found, indicate that the number does not exist in the array.
This exercise demonstrates the efficiency of binary search, which significantly reduces the search time in sorted arrays compared to a linear search.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
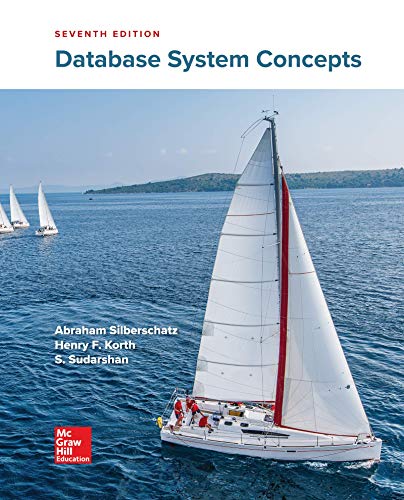
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
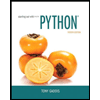
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
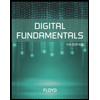
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
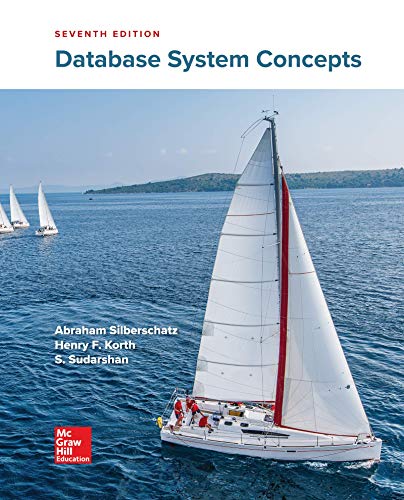
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
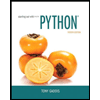
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
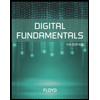
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
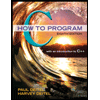
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
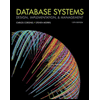
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
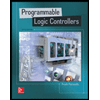
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education