There is code already created for you in the attachment. This code asks the user for their age. It should then put up a message, depending on the age. The exact message does not matter here, do not worry about that, just focus on the age ranges. Under 18 You have so much ahead of you. Between 18(inclusive) and 21(exclusive) Between 21(inclusive) and 63(exclusive) You can vote! You can drink and vote.... 63 or over You can drink, vote, and get social security The program then asks the user if they want to enter another age. If they click yes, the program starts all over, otherwise it ends.
There is code already created for you in the attachment. This code asks the user for their age. It should then put up a message, depending on the age. The exact message does not matter here, do not worry about that, just focus on the age ranges. Under 18 You have so much ahead of you. Between 18(inclusive) and 21(exclusive) Between 21(inclusive) and 63(exclusive) You can vote! You can drink and vote.... 63 or over You can drink, vote, and get social security The program then asks the user if they want to enter another age. If they click yes, the program starts all over, otherwise it ends.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please write code that would run in VBA excel attached is the code to fix and instructions

Transcribed Image Text:The provided image is a screenshot of a Visual Basic for Applications (VBA) code snippet. The subroutine, named `AgeLaws`, interacts with the user to provide age-based messages.
### Code Explanation:
1. **Variable Declaration:**
- `Dim intAge As Integer`: Declares a variable `intAge` to store the user's input age.
- `Dim intAgain As Integer`: Declares a variable `intAgain` for storing the user's response to check another age.
2. **User Input:**
- `intAge = InputBox("How old are you?", "Enter Age")`: Prompts the user to enter their age using an input box.
3. **Conditional Statements:**
- `If intAge >= 18 And intAge < 21 Then`: Checks if the age is between 18 and 20, inclusively, and sets `strAnswer` accordingly.
- `strAnswer = "You can vote!"`: Assigns a message stating the user can vote.
- `ElseIf intAge >= 21 < 63 Then`: Checks if the age is between 21 and 62, inclusively.
- `strAnswer = "You can vote and drink. Please, not at the same time."`: Assigns a message indicating the user can vote and drink.
- `ElseIf Age >= 63 Then`: Checks if the age is 63 or older.
- `strAnswer = "You can vote, drink, and you get Social Security!"`: Assigns a message about voting, drinking, and receiving Social Security.
- `Else`: For any age that does not meet the above conditions.
- `strAnswer = "You have so many things ahead of you!"`: Assigns a message of encouragement for younger users.
4. **Message Display:**
- `MsgBox strAnswer`: Displays a message box with the `strAnswer` message.
5. **Repeat Prompt:**
- `intAgain = MsgBox("Do you want to check another age?", vbYesNo)`: Asks the user if they want to enter another age.
- `If intAgain = 0 Then AgeLaws End If`: If the user selects "Yes" (0), the subroutine `AgeLaws` runs again.
### No Graphs or Diagrams:
The image solely contains text and does not include any graphs or diagrams.

Transcribed Image Text:**Programming Exercise: Age-Based Messages**
This task involves debugging a pre-existing code that asks the user for their age and outputs a message based on the age range they fall into. The exact message content is not the focus; instead, concentrate on the following age ranges:
- **Under 18**: "You have so much ahead of you."
- **Between 18 (inclusive) and 21 (exclusive)**: "You can vote!"
- **Between 21 (inclusive) and 63 (exclusive)**: "You can drink and vote...."
- **63 or over**: "You can drink, vote, and get social security."
**Program Functionality:**
After displaying the message, the program prompts the user to enter another age. If the user clicks 'yes,' the program restarts; otherwise, it ends.
**Exercise Instructions:**
- Identify and fix several errors preventing proper functionality.
- Test the code with various inputs.
- Focus on correcting existing code; no need to add new code sections.
**Note:** This exercise is designed to help you practice debugging and logical thinking in programming. Remember to check your logic against the specified age ranges carefully.
Expert Solution

Step 1
The solution of given problem need to use If-Else-If ladder, in which we test the age and print message as per range that your input follow. The first error in given code is "ElseIf intAge >= 21 < 63 " here we need to use logical And operator to combine two conditions, so the correct code is "ElseIf intAge >= 21 And intAge < 63".
The next error is "If intAgain = 0 then", because the program starts again if user click on Yes button, the value of Yes button is 6, so we need to use the code "If intAgain = 6 Then" to call subroutine again.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
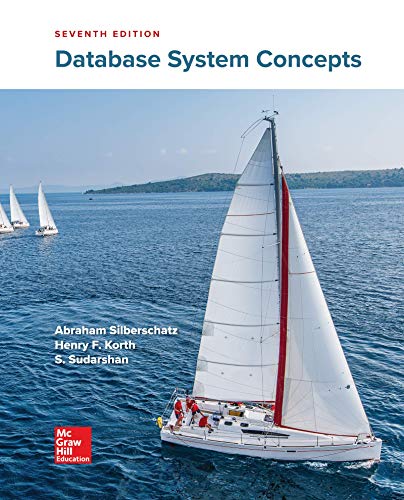
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
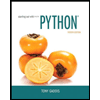
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
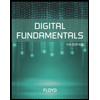
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
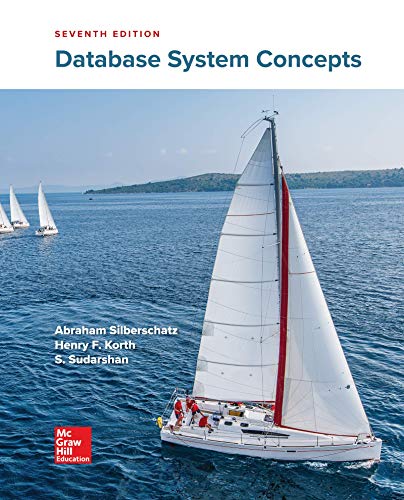
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
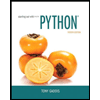
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
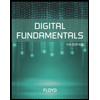
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
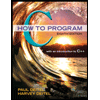
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
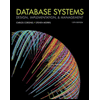
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
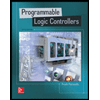
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education