Write a program to take the name of a file in the root directory and extract its contents to your local disk. Hint: • • Sector number to byte offset Sector number to next sector number (using the FAT) readCluster(clusterNumber) -> bytes array readSector(sectorNumber) -> bytes array readFromSector(sectorNumber, bytes) -> bytes array read MBR -> boot_t getRootDirEntries()-> dir_entry_t[] findRootDirEntry(string filename, dir_entry_t entries[])-> dir_entry_t // This has to do a search with a loop .fseventsd ATTRIB.EXE AUTOEXEC.BAT CHKDSK.EXE COMMAND.COM CONFIG.SYS COUNTRY.SYS COUNTRY.TX DEBUG.EXE DEFRAG.EXE DEFRAG.HL DOSSETUP.INI DRVSPACE.BIN EDIT.COM EGA.CP EGA2.CP EGA3.CP EMM386.EX EXPAND.EXE FDISK.EXE FORMAT.COM IO.SYS ISO.CP KEYB.COM KEYBOARD.SYS KEYBRD2.SY MEM.EX MSCDEX.EXE MSDOS.SYS NETWORKS.TXT NLSFUNC.EXE PACKING.LST QBASIC.EXE README.TXT REPLACE.EX RESTORE.EX SCANDISK.EXE SCANDISK.INI SETUP.EXE SETUP.MSG SYS.COM XCOPY.EX The Wikipedia lays out the equation for converting that number into a sector address (after which, you just multiply by bytes per sector to get there). I wrote a little script to make that equation more readable. Here's what it looks like: A simple formula translates a volume's given cluster number CN to a logical sector number LSN : [24][25][26] 1. Determine (once) Size_of_System_Area = Reserved Sector_Count + number_of_FATS x Sectors_per_FAT +ceil((32x Root Directory_Entries )/ Sector_Size), where the reserved sector count RSC is stored at offset 0x00E, the number of FATS FN at offset 0x010, the sectors per FAT SF at offset 0x016 (FAT12/FAT16) or ex024 (FAT32), the root directory entries RDE at offset 0x011, the sector size ss at offset 0x00в, and ceil(x) rounds up to a whole number. 2. Determine Logical_Sector_Number = Size_of_System_Area +( Cluster_number -2)x Sectors_per_Cluster, where the sectors per cluster SC are stored at offset 0x00D. esc 1 2 # W3 $ 4 % 85 Λ <6 & 7 18 8 9
Write a program to take the name of a file in the root directory and extract its contents to your local disk. Hint: • • Sector number to byte offset Sector number to next sector number (using the FAT) readCluster(clusterNumber) -> bytes array readSector(sectorNumber) -> bytes array readFromSector(sectorNumber, bytes) -> bytes array read MBR -> boot_t getRootDirEntries()-> dir_entry_t[] findRootDirEntry(string filename, dir_entry_t entries[])-> dir_entry_t // This has to do a search with a loop .fseventsd ATTRIB.EXE AUTOEXEC.BAT CHKDSK.EXE COMMAND.COM CONFIG.SYS COUNTRY.SYS COUNTRY.TX DEBUG.EXE DEFRAG.EXE DEFRAG.HL DOSSETUP.INI DRVSPACE.BIN EDIT.COM EGA.CP EGA2.CP EGA3.CP EMM386.EX EXPAND.EXE FDISK.EXE FORMAT.COM IO.SYS ISO.CP KEYB.COM KEYBOARD.SYS KEYBRD2.SY MEM.EX MSCDEX.EXE MSDOS.SYS NETWORKS.TXT NLSFUNC.EXE PACKING.LST QBASIC.EXE README.TXT REPLACE.EX RESTORE.EX SCANDISK.EXE SCANDISK.INI SETUP.EXE SETUP.MSG SYS.COM XCOPY.EX The Wikipedia lays out the equation for converting that number into a sector address (after which, you just multiply by bytes per sector to get there). I wrote a little script to make that equation more readable. Here's what it looks like: A simple formula translates a volume's given cluster number CN to a logical sector number LSN : [24][25][26] 1. Determine (once) Size_of_System_Area = Reserved Sector_Count + number_of_FATS x Sectors_per_FAT +ceil((32x Root Directory_Entries )/ Sector_Size), where the reserved sector count RSC is stored at offset 0x00E, the number of FATS FN at offset 0x010, the sectors per FAT SF at offset 0x016 (FAT12/FAT16) or ex024 (FAT32), the root directory entries RDE at offset 0x011, the sector size ss at offset 0x00в, and ceil(x) rounds up to a whole number. 2. Determine Logical_Sector_Number = Size_of_System_Area +( Cluster_number -2)x Sectors_per_Cluster, where the sectors per cluster SC are stored at offset 0x00D. esc 1 2 # W3 $ 4 % 85 Λ <6 & 7 18 8 9
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please help with this program. something is not right as i dont think the file is being read. the filename has to be adapatable to any length of filename so it can search the directory in disk img . i am getting this error "End of file reached unexpectedly."
and i think sector number is being calculated wrong. please help make this program compile and run and also generate an extracted output file. please if you can show on your end that this program worked with a successfully extracted output that would be great.
#include
#include
#include
#include
#pragma pack(push, 1)
typedef struct {
int8_tjump_to_bootstrap[3];
int8_toem_id[8];
uint16_tbytes_per_sector;
uint8_tsectors_per_cluster;
uint16_treserved_sectors;
uint8_tfat_copies;
uint16_troot_dir_entries;
uint16_ttotal_sectors;
uint8_tmedia_descriptor_type;
uint16_tsectors_per_fat;
uint16_tsectors_per_track;
uint16_theads;
uint32_thidden_sectors;
uint32_ttotal_sectors2;
uint8_tdrive_index;
uint8_t_stuff;
uint8_tsignature;
uint32_tid;
int8_tlabel[11];
int8_ttype[8];
int8_t_more_stuff[448];
uint16_tsig;
} boot_t;
typedef struct {
int8_tfilename[8];
int8_textension[3];
int8_tattributes;
int8_t_reserved[10];
uint16_tupdate_time;
uint16_tupdate_date;
uint16_tstarting_cluster;
uint32_tfile_size;
} dir_entry_t;
#pragma pack(pop)
void readSector(FILE *disk, uint32_t sectorNumber, uint8_t *buffer, uint16_t bytesPerSector) {
if (disk==NULL) {
fprintf(stderr, "Invalid file pointer.\n");
return;
}
if (buffer==NULL) {
fprintf(stderr, "Invalid buffer pointer.\n");
return;
}
if (fseek(disk, sectorNumber*bytesPerSector, SEEK_SET) !=0) {
perror("Error seeking file");
return;
}
size_tbytesRead=fread(buffer, 1, bytesPerSector, disk);
if (bytesRead!=bytesPerSector) {
if (feof(disk)) {
fprintf(stderr, "End of file reached unexpectedly.\n");
} else if (ferror(disk)) {
perror("Error reading file");
}
return;
}
}
void readMBR(FILE *disk, boot_t *bootSector) {
uint8_tbuffer[512];
readSector(disk, 0, buffer, 512);
memcpy(bootSector, buffer, sizeof(boot_t));
}
dir_entry_t* getRootDirEntries(FILE *disk, boot_t *bootSector) {
uint16_trootDirSectors= ((bootSector->root_dir_entries*32) + (bootSector->bytes_per_sector-1)) /bootSector->bytes_per_sector;
uint8_t*buffer= (uint8_t*)malloc(rootDirSectors*bootSector->bytes_per_sector);
readSector(disk, bootSector->reserved_sectors+ (bootSector->fat_copies*bootSector->sectors_per_fat), buffer, rootDirSectors*bootSector->bytes_per_sector);
return (dir_entry_t*)buffer;
}
dir_entry_t* findRootDirEntry(const char *filename, dir_entry_t *entries, uint16_t numEntries) {
for (inti=0; ireserved_sectors+ (bootSector->fat_copies*bootSector->sectors_per_fat) + ((bootSector->root_dir_entries*32+bootSector->bytes_per_sector-1) /bootSector->bytes_per_sector);
uint32_tfirstSectorOfCluster= ((clusterNumber-2) *bootSector->sectors_per_cluster) +firstDataSector;
for (inti=0; isectors_per_cluster; i++) {
readSector(disk, firstSectorOfCluster+i, buffer+ (i*bootSector->bytes_per_sector), bootSector->bytes_per_sector);
}
}
void extractFile(FILE *disk, dir_entry_t *entry, boot_t *bootSector) {
uint8_t*buffer= (uint8_t*)malloc(entry->file_size);
uint32_tcluster=entry->starting_cluster;
uint32_tbytesRead=0;
while (bytesReadfile_size) {
readCluster(disk, cluster, buffer+bytesRead, bootSector);
bytesRead+=bootSector->bytes_per_sector*bootSector->sectors_per_cluster;
// In a real implementation, we should follow the FAT to find the next cluster
cluster++; // This is just a simplification for the example
}
FILE*output=fopen("output_file", "wb");
fwrite(buffer, 1, entry->file_size, output);
fclose(output);
free(buffer);
}
int main(int argc, char *argv[]) {
if (argc!=3) {
fprintf(stderr, "Usage: %s \n", argv[0]);
return1;
}
constchar*diskImage=argv[1];
constchar*filename=argv[2];
FILE*disk=fopen(argv[1], "rb");
if (disk==NULL) {
perror("Error opening disk image");
return1;
}
boot_tbootSector;
readMBR(disk, &bootSector);
dir_entry_t*entries=getRootDirEntries(disk, &bootSector);
dir_entry_t*entry=findRootDirEntry(filename, entries, bootSector.root_dir_entries);
if (entry) {
extractFile(disk, entry, &bootSector);
printf("File extracted successfully.\n");
} else {
fprintf(stderr, "File not found in root directory.\n");
}
free(entries);
fclose(disk);
return0;
}
![Write a program to take the name of a file in the root directory and extract its contents to your
local disk.
Hint:
•
•
Sector number to byte offset
Sector number to next sector number (using the FAT)
readCluster(clusterNumber) -> bytes array
readSector(sectorNumber) -> bytes array
readFromSector(sectorNumber, bytes) -> bytes array
read MBR -> boot_t
getRootDirEntries()-> dir_entry_t[]
findRootDirEntry(string filename, dir_entry_t entries[])-> dir_entry_t // This has to do a
search with a loop
.fseventsd
ATTRIB.EXE
AUTOEXEC.BAT
CHKDSK.EXE
COMMAND.COM
CONFIG.SYS
COUNTRY.SYS
COUNTRY.TX
DEBUG.EXE
DEFRAG.EXE
DEFRAG.HL
DOSSETUP.INI
DRVSPACE.BIN
EDIT.COM
EGA.CP
EGA2.CP
EGA3.CP
EMM386.EX
EXPAND.EXE
FDISK.EXE
FORMAT.COM
IO.SYS
ISO.CP
KEYB.COM
KEYBOARD.SYS
KEYBRD2.SY
MEM.EX
MSCDEX.EXE
MSDOS.SYS
NETWORKS.TXT
NLSFUNC.EXE
PACKING.LST
QBASIC.EXE
README.TXT
REPLACE.EX
RESTORE.EX
SCANDISK.EXE
SCANDISK.INI
SETUP.EXE
SETUP.MSG
SYS.COM
XCOPY.EX](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4217e83c-b4b2-47da-9d7a-2d6031448fca%2F18d95eac-4595-43fe-b6e0-a48c41710ab2%2F2db7639_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write a program to take the name of a file in the root directory and extract its contents to your
local disk.
Hint:
•
•
Sector number to byte offset
Sector number to next sector number (using the FAT)
readCluster(clusterNumber) -> bytes array
readSector(sectorNumber) -> bytes array
readFromSector(sectorNumber, bytes) -> bytes array
read MBR -> boot_t
getRootDirEntries()-> dir_entry_t[]
findRootDirEntry(string filename, dir_entry_t entries[])-> dir_entry_t // This has to do a
search with a loop
.fseventsd
ATTRIB.EXE
AUTOEXEC.BAT
CHKDSK.EXE
COMMAND.COM
CONFIG.SYS
COUNTRY.SYS
COUNTRY.TX
DEBUG.EXE
DEFRAG.EXE
DEFRAG.HL
DOSSETUP.INI
DRVSPACE.BIN
EDIT.COM
EGA.CP
EGA2.CP
EGA3.CP
EMM386.EX
EXPAND.EXE
FDISK.EXE
FORMAT.COM
IO.SYS
ISO.CP
KEYB.COM
KEYBOARD.SYS
KEYBRD2.SY
MEM.EX
MSCDEX.EXE
MSDOS.SYS
NETWORKS.TXT
NLSFUNC.EXE
PACKING.LST
QBASIC.EXE
README.TXT
REPLACE.EX
RESTORE.EX
SCANDISK.EXE
SCANDISK.INI
SETUP.EXE
SETUP.MSG
SYS.COM
XCOPY.EX
![The Wikipedia lays out the equation for converting that number into a sector address (after which, you just multiply by bytes per sector to get there).
I wrote a little script to make that equation more readable. Here's what it looks like:
A simple formula translates a volume's given cluster number CN to a logical sector number LSN : [24][25][26]
1. Determine (once) Size_of_System_Area = Reserved Sector_Count + number_of_FATS x Sectors_per_FAT +ceil((32x
Root Directory_Entries )/ Sector_Size), where the reserved sector count RSC is stored at offset 0x00E, the number of
FATS FN at offset 0x010, the sectors per FAT SF at offset 0x016 (FAT12/FAT16) or ex024 (FAT32), the root directory entries RDE at
offset 0x011, the sector size ss at offset 0x00в, and ceil(x) rounds up to a whole number.
2. Determine Logical_Sector_Number
=
Size_of_System_Area +( Cluster_number -2)x Sectors_per_Cluster, where the
sectors per cluster SC are stored at offset 0x00D.
esc
1
2
#
W3
$
4
%
85
Λ
<6
&
7
18
8
9](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4217e83c-b4b2-47da-9d7a-2d6031448fca%2F18d95eac-4595-43fe-b6e0-a48c41710ab2%2Fuh98zoo_processed.jpeg&w=3840&q=75)
Transcribed Image Text:The Wikipedia lays out the equation for converting that number into a sector address (after which, you just multiply by bytes per sector to get there).
I wrote a little script to make that equation more readable. Here's what it looks like:
A simple formula translates a volume's given cluster number CN to a logical sector number LSN : [24][25][26]
1. Determine (once) Size_of_System_Area = Reserved Sector_Count + number_of_FATS x Sectors_per_FAT +ceil((32x
Root Directory_Entries )/ Sector_Size), where the reserved sector count RSC is stored at offset 0x00E, the number of
FATS FN at offset 0x010, the sectors per FAT SF at offset 0x016 (FAT12/FAT16) or ex024 (FAT32), the root directory entries RDE at
offset 0x011, the sector size ss at offset 0x00в, and ceil(x) rounds up to a whole number.
2. Determine Logical_Sector_Number
=
Size_of_System_Area +( Cluster_number -2)x Sectors_per_Cluster, where the
sectors per cluster SC are stored at offset 0x00D.
esc
1
2
#
W3
$
4
%
85
Λ
<6
&
7
18
8
9
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
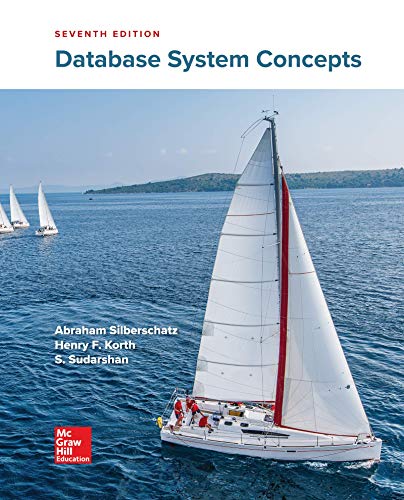
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
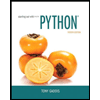
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
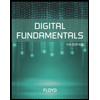
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
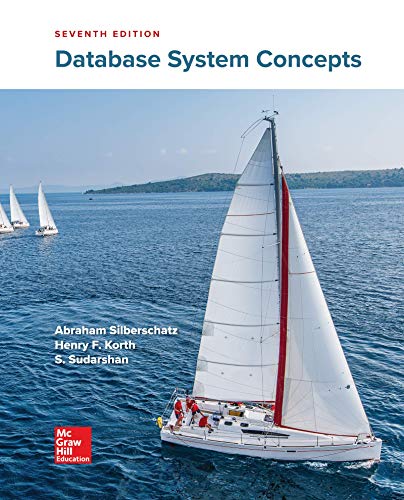
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
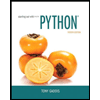
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
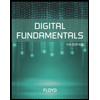
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
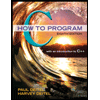
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
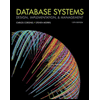
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
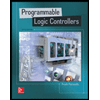
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education