I have written a program that requires entering 3 first and last names and generates a file entitled, "ACL.txt" (Screenshot Below). Now I need a program that goes and searches the names I have typed in the "ACL.txt" file. For the program, the instructions are: A. First, read the names from the “ACL.txt” file. B. Next, create a loop to do the following: i. Query the user to enter a name. ii. Search the 3 names for the first and last name that the user entered. iii. If the user’s name is found in the “ACL.txt” file, 1. Display, “ACCESS GRANTED…! On the screen. iv. Else 1. Display “ACCESS DENIED…!” On the screen. v. Ask the user if he/she would like to search for another name in the “ACL.txt”. vi. If the user enters ‘Y’, 1. Go back to the top of the loop. vii. Else 1. Exit program
I have written a
Now I need a program that goes and searches the names I have typed in the "ACL.txt" file.
For the program, the instructions are:
A. First, read the names from the “ACL.txt” file.
B. Next, create a loop to do the following:
i. Query the user to enter a name.
ii. Search the 3 names for the first and last name that the user entered.
iii. If the user’s name is found in the “ACL.txt” file,
1. Display, “ACCESS GRANTED…! On the screen.
iv. Else
1. Display “ACCESS DENIED…!” On the screen.
v. Ask the user if he/she would like to search for another name in the “ACL.txt”.
vi. If the user enters ‘Y’,
1. Go back to the top of the loop.
vii. Else
1. Exit program.
![**C++ Program to Write Names to a File**
This example demonstrates how to write names to a file using a C++ program. The program allows the user to input a set number of names, which are then stored in a file named "ACL.txt".
### C++ Code
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
const int MAX_NAMES_IN_ACL = 3;
int main()
{
string asACLNames[MAX_NAMES_IN_ACL];
string strACLFileName = "ACL.txt";
ofstream ptrACL(strACLFileName);
for (int i = 0; i < MAX_NAMES_IN_ACL; i++)
{
cout << "Enter ACL name #" << i + 1 << ": ";
getline(cin, asACLNames[i]);
cout << endl;
}
if (ptrACL.is_open())
{
for (int i = 0; i < MAX_NAMES_IN_ACL; i++)
ptrACL << asACLNames[i] << endl;
ptrACL.close();
cout << "ACL entries successfully written to file: " << strACLFileName << "." << endl;
}
else
{
cout << "\n\nUnable to open file: " << strACLFileName << "\n\n";
}
return 0;
}
```
### Explanation
1. **Includes and Namespace**:
- `#include <iostream>`, `#include <fstream>`, `#include <string>`: These header files are included to facilitate input-output operations and string handling.
- `using namespace std;`: Allows usage of standard library classes and functions without the `std::` prefix.
2. **Constants and Variables**:
- `const int MAX_NAMES_IN_ACL = 3;`: Declares a constant for the maximum number of names.
- `string asACLNames[MAX_NAMES_IN_ACL];`: Declares an array to hold the names.
- `string strACLFileName = "ACL.txt";`: Specifies the file name.
3. **Main Function**:
- Collects names from the user and stores them in the `asACLNames` array.
- Opens the file "ACL.txt" for writing.
- Writes each collected name into the file if the file is successfully opened.
- Closes the file and outputs a success message](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff8fa7aa4-dafc-4c07-b274-462aa6ff800c%2Fe0184e2a-bd89-4ad6-ab18-669149b247b7%2Fko8gc09_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 4 images

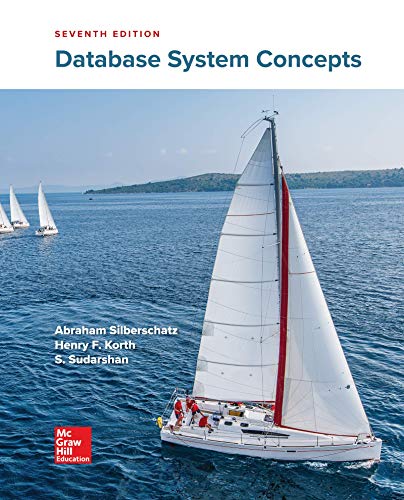
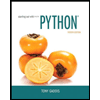
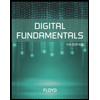
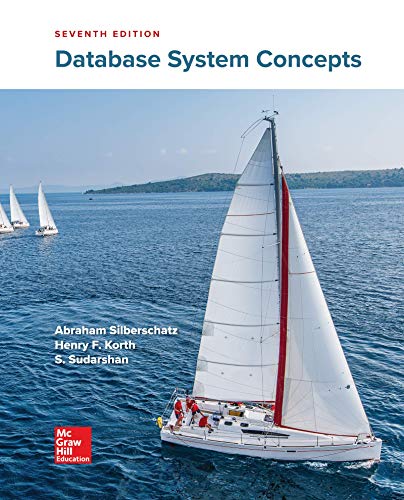
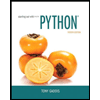
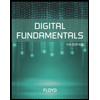
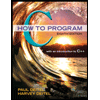
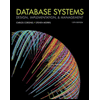
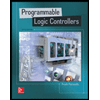