Need help with writing the program creating two different class files (Instruction are attatched in the images). Please provide with ss of your code if possible.
Need help with writing the program creating two different class files (Instruction are attatched in the images). Please provide with ss of your code if possible.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Need help with writing the program creating two different class files (Instruction are attatched in the images). Please provide with ss of your code if possible.

Transcribed Image Text:### Class Design for Cylinder Representation
In this exercise, we will design a class named `Cylinder` to represent cylinders.
#### Class Specifications
The `Cylinder` class will contain the following features:
- **Private Data Fields:**
- `radius`: A double that specifies the radius of a cylinder with a default value of 1.0.
- `length`: A double that specifies the length of a cylinder with a default value of 1.0.
- `numberOfObjects`: An integer static data field that tracks the number of cylinder objects created. The default value is `0`.
- **Constructors:**
- A no-argument constructor that creates a cylinder with default values.
- A constructor with parameters that accepts specified values for radius and length.
- **Methods:**
- Get and set methods for the `radius` and `length` fields.
- `getArea()`: A method that returns the base area of the cylinder.
- `getVolume()`: A method that returns the volume of the cylinder.
- `getNumberOfObjects()`: A static method that returns the value of the `numberOfObjects` field.
#### Requirements
1. **UML Diagram and Implementation:**
- Draw the UML (Unified Modeling Language) diagram for the `Cylinder` class.
- Implement the class ensuring that data fields are private, with constructors and other methods set to public.
2. **Test Program:**
- Write a test program that performs the following tasks:
- Create the first cylinder object using the no-argument constructor.
- Create a second cylinder object using the constructor with parameters (setting radius to 2.5 and length to 12.3).
- Create a third cylinder object using the no-argument constructor.
- Modify the third cylinder’s radius to 5.0 and length to 6.5.
- Print the radius and length of the first cylinder.
- Print the area of the second cylinder.
- Print the volume of the third cylinder.
- Print the value of the `numberOfObjects` data field.
### Detailed Steps
#### Step 1: UML Diagram
Create a UML diagram which should visually represent the class with its data fields and methods, specifying their visibility (private or public).
#### Step 2: Cylinder Class Implementation
Write the implementation code for the `Cylinder` class based on the above-mentioned specifications.

Transcribed Image Text:### Cylinder Properties and Objects
The following information provides details about three different cylindrical objects:
1. **First Cylinder:**
- **Radius:** 1.0 units
- **Length:** 1.0 units
2. **Second Cylinder:**
- **Base Area:** 19.634954084936208 square units
3. **Third Cylinder:**
- **Volume:** 510.5088062083414 cubic units
In summary, a total of **3 objects** have been created with the specified properties.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 3 images

Recommended textbooks for you
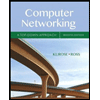
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
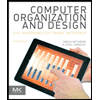
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
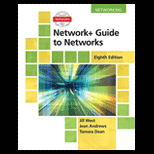
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
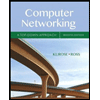
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
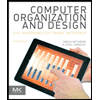
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
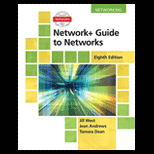
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
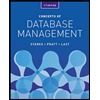
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
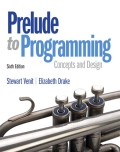
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
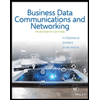
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY