The program should print out a 10x10 multiplication table, using nested for() loops. It should ALSO print column headers (1 through 10) and row numbers, as shown. All numbers should be equally spaced using cout << setw(5) << variableName; so that the columns align, though the example below may not look that way in your browser. You must have #include at the top of your program to use the setw() function of the cout object, which sets the width of field for printing the variable's value. Expected output: * 1 2 3 4 5 6 7 8 9 10 1 1 2 3 4 5 6 7 8 9 10 2 2 4 6 8 10 12 14 16 18 20 3 3 6 9 12 15 18 21 24 27 30 4 4 8 12 16 20 24 28 32 36 40 5 5 10 15 20 25 30 35 40 45 50 6 6 12 18 24 30 36 42 48 54 60 7 7 14 21 28 35 42 49 56 63 70 8 8 16 24 32 40 48 56 64 72 80 9 9 18 27 36 45 54 63 72 81 90 10 10 20 30 40 50 60 70 80 90 100 Note that you will need an extra for () loop at the beginning to print the column headers, after you print the asterisk. And in each row (outer) loop, print the row index before entering the column loop, to provide the row headers on the left.
The program should print out a 10x10 multiplication table, using nested for() loops.
It should ALSO print column headers (1 through 10) and row numbers, as shown. All numbers should be equally spaced using
cout << setw(5) << variableName;
so that the columns align, though the example below may not look that way in your browser.
You must have
#include <iomanip>
at the top of your program to use the setw() function of the cout object, which sets the width of field for printing the variable's value.
Expected output:
* 1 2 3 4 5 6 7 8 9 10 1 1 2 3 4 5 6 7 8 9 10 2 2 4 6 8 10 12 14 16 18 20 3 3 6 9 12 15 18 21 24 27 30 4 4 8 12 16 20 24 28 32 36 40 5 5 10 15 20 25 30 35 40 45 50 6 6 12 18 24 30 36 42 48 54 60 7 7 14 21 28 35 42 49 56 63 70 8 8 16 24 32 40 48 56 64 72 80 9 9 18 27 36 45 54 63 72 81 90 10 10 20 30 40 50 60 70 80 90 100
Note that you will need an extra for () loop at the beginning to print the column headers, after you print the asterisk. And in each row (outer) loop, print the row index before entering the column loop, to provide the row headers on the left.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

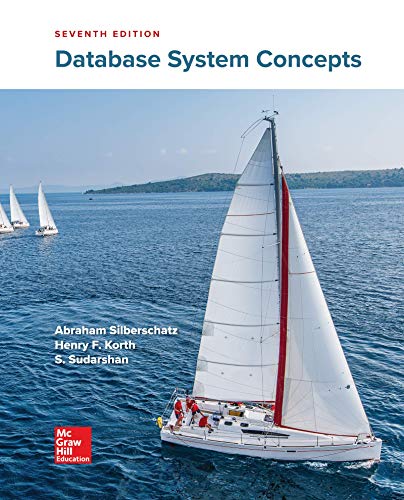
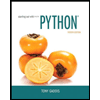
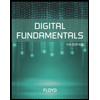
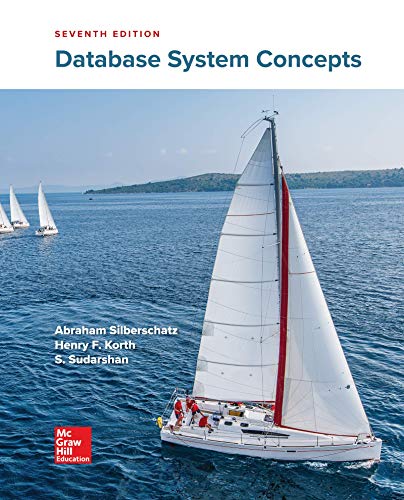
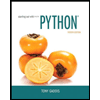
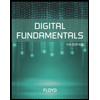
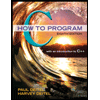
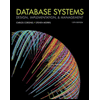
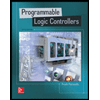