Loop through the animalList created in a previous question and print the total time taken and total time given for each animal. Example output: Zirly the Zebra - Time taken for all behaviors: 890 minutes of 1000 Henry the Hawk - Time taken for all behaviors: 350 minues of 1000 Choose one of the following from the above choices (A, B, C or D within the code): // Assume the following variables exist: Behavior[] someBehaviors = // Omitted for brevity Animal zirly = Animal("Zirly the Zebra", 812.3, someBehaviors); Animal henry = Animal("Henry the Hawk", 35.5, someBehaviors); Animal[] animalList = new Animal[]{ zirly, henry }; // --------------------------- // A for(int i = animalList.length; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; int timeTakenSum = 0; int timeGivenSum = 0; for(j = 0; j < tempAnimal.getBehaviorList().length; j++) { Behavior tempBehavior = tempAnimal.getBehaviorList()[j]; timeTakenSum += tempBehavior.getTimeToComplete(); timeGivenSum += tempBehavior.getTimeGiven(); } System.out.println(tempAnimal.getAnimalName() + " - " + "Time taken for all behaviors: " + timeTakenSum + " minutes of " + timeGivenSum); } // B for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; int timeTakenSum = 0; int timeGivenSum = 0; for(j = 0; j < tempAnimal.getBehaviorList().length; j++) { Behavior tempBehavior = tempAnimal.getBehaviorList()[j]; timeTakenSum -= tempBehavior.getTimeToComplete(); timeGivenSum -= tempBehavior.getTimeGiven(); } System.out.println(tempAnimal.getAnimalName() + " - " + "Time taken for all behaviors: " + timeTakenSum + " minutes of " + timeGivenSum); } // C for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; int timeTakenSum = 0; int timeGivenSum = 0; for(j = 0; j < tempAnimal.getBehaviorList().length; j++) { Behavior tempBehavior = tempAnimal.getBehaviorList()[j]; timeTakenSum += tempBehavior.getTimeToComplete(); timeGivenSum += tempBehavior.getTimeGiven(); } System.out.println(tempAnimal.getAnimalName() + " - " + "Time taken for all behaviors: " + timeTakenSum + " minutes of " + timeGivenSum); } // D for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; int timeTakenSum = 0; int timeGivenSum = 0; for(j = 0; j < tempAnimal.getBehaviorList().length; j++) { Behavior tempBehavior = tempAnimal.getBehaviorList()[j]; timeTakenSum /= tempBehavior.getTimeToComplete(); timeGivenSum /= tempBehavior.getTimeGiven(); } System.out.println(tempAnimal.getAnimalName() + " - " + "Time taken for all behaviors: " + timeTakenSum + " minutes of " + timeGivenSum); }
Loop through the animalList created in a previous question and print the total time taken and total time given for each animal.
Example output:
Zirly the Zebra - Time taken for all behaviors: 890 minutes of 1000 Henry the Hawk - Time taken for all behaviors: 350 minues of 1000
Choose one of the following from the above choices (A, B, C or D within the code):
// Assume the following variables exist:
Behavior[] someBehaviors = // Omitted for brevity
Animal zirly = Animal("Zirly the Zebra", 812.3, someBehaviors);
Animal henry = Animal("Henry the Hawk", 35.5, someBehaviors);
Animal[] animalList = new Animal[]{ zirly, henry };
// ---------------------------
// A
for(int i = animalList.length; i < animalList.length; i++) {
Animal tempAnimal = animalList[i];
int timeTakenSum = 0;
int timeGivenSum = 0;
for(j = 0; j < tempAnimal.getBehaviorList().length; j++) {
Behavior tempBehavior = tempAnimal.getBehaviorList()[j];
timeTakenSum += tempBehavior.getTimeToComplete();
timeGivenSum += tempBehavior.getTimeGiven();
}
System.out.println(tempAnimal.getAnimalName() + " - " +
"Time taken for all behaviors: " + timeTakenSum + " minutes of " +
timeGivenSum);
}
// B
for(int i = 0; i < animalList.length; i++) {
Animal tempAnimal = animalList[i];
int timeTakenSum = 0;
int timeGivenSum = 0;
for(j = 0; j < tempAnimal.getBehaviorList().length; j++) {
Behavior tempBehavior = tempAnimal.getBehaviorList()[j];
timeTakenSum -= tempBehavior.getTimeToComplete();
timeGivenSum -= tempBehavior.getTimeGiven();
}
System.out.println(tempAnimal.getAnimalName() + " - " +
"Time taken for all behaviors: " + timeTakenSum + " minutes of " +
timeGivenSum);
}
// C
for(int i = 0; i < animalList.length; i++) {
Animal tempAnimal = animalList[i];
int timeTakenSum = 0;
int timeGivenSum = 0;
for(j = 0; j < tempAnimal.getBehaviorList().length; j++) {
Behavior tempBehavior = tempAnimal.getBehaviorList()[j];
timeTakenSum += tempBehavior.getTimeToComplete();
timeGivenSum += tempBehavior.getTimeGiven();
}
System.out.println(tempAnimal.getAnimalName() + " - " +
"Time taken for all behaviors: " + timeTakenSum + " minutes of " +
timeGivenSum);
}
// D
for(int i = 0; i < animalList.length; i++) {
Animal tempAnimal = animalList[i];
int timeTakenSum = 0;
int timeGivenSum = 0;
for(j = 0; j < tempAnimal.getBehaviorList().length; j++) {
Behavior tempBehavior = tempAnimal.getBehaviorList()[j];
timeTakenSum /= tempBehavior.getTimeToComplete();
timeGivenSum /= tempBehavior.getTimeGiven();
}
System.out.println(tempAnimal.getAnimalName() + " - " +
"Time taken for all behaviors: " + timeTakenSum + " minutes of " +
timeGivenSum);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

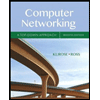
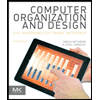
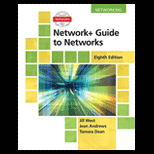
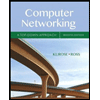
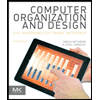
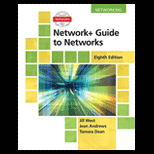
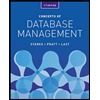
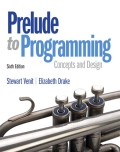
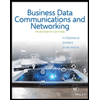