The Problem: You have a function that is called repeatedly to compute the same values. This function is taking a long time to do its job. How can you make things faster? Let's take a look at an example. Below is a rectangle class. The function compute_serial returns a unique serial string which is used for sorting the shapes classes. class rectangle { private: int color; int width; int height; rectangle(int i_color, int i_width, int i_height): color(i_color), width(i_width), height(i_height) {} void set_color(int the_color) { color = the_color; } char serial_string[100]; // Unique ID string char* get_serial() { snprintf(serial_string, sizeof(serial_string), “rect-%d x %d / %d”, width, height, color); return (serial_string); } Examination of this class shows that it uses the snprintf to compute the serial string. This is an extremely costly function to use. We need optimize out this function if at all possible.
The Problem: You have a function that is called repeatedly to compute the
same values. This function is taking a long time to do its job. How can you make
things faster?
Let's take a look at an example. Below is a rectangle class. The
function compute_serial returns a unique serial string which is used for
sorting the shapes classes.
class rectangle {
private:
int color;
int width;
int height;
rectangle(int i_color, int i_width, int i_height):
color(i_color), width(i_width), height(i_height)
{}
void set_color(int the_color) {
color = the_color;
}
char serial_string[100]; // Unique ID string
char* get_serial() {
snprintf(serial_string, sizeof(serial_string),
“rect-%d x %d / %d”, width, height, color);
return (serial_string);
}
Examination of this class shows that it uses the snprintf to compute the serial string. This is an extremely costly function to use. We need optimize out this function if at all possible.
Dont copy old bartleby answer its wrong

Step by step
Solved in 3 steps

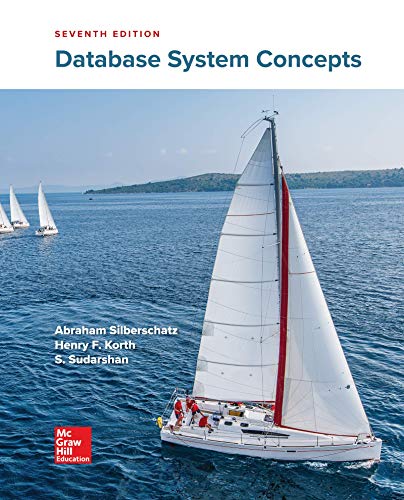
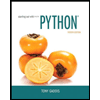
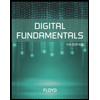
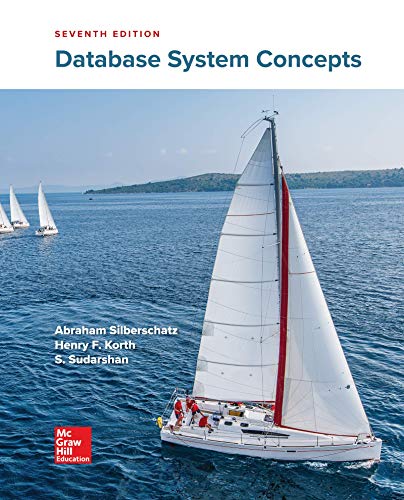
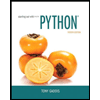
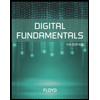
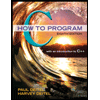
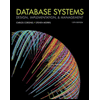
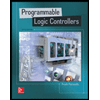