1. Write a method that returns an int array using user's inputs from the console window. The method should have the following header. The formal parameter size is the size of the return array. public static int[] inputArray (int size) 2. Write a method that calculates and returns the average of an array with the following headers. public static double average (int[] list) 3. Write a method to add two int arrays, assuming that arrays referred by variables a and b are of the same size. The header of the method is as follows. public static int[] add (int[] listl, int[] list2) The add method should return an array of the same size as the input arrays, with each element to be the sum of the elements from the input arrays. For example, if the two input arrays are [3, 5, 2, 6, 4, 9] and [1, 2, 3, 4, 5, 6], the returned sum array should be [4, 7, 5, 10, 9, 15]. 4. Write a method to display an int array's contents on the console window. The header of the method is as below. public static void displayArray (int[] list) 5. Write the main method to: a. Create two input arrays with the same size by invoking the inputArray method twice in the main method. b. Calculate the average of the first int array by invoking the average method. Display the average result on the console window. c. Calculate the array sum of both input arrays by invoking the add method. d. Display the sum array by invoking the display Array method.
1. Write a method that returns an int array using user's inputs from the console window. The method should have the following header. The formal parameter size is the size of the return array. public static int[] inputArray (int size) 2. Write a method that calculates and returns the average of an array with the following headers. public static double average (int[] list) 3. Write a method to add two int arrays, assuming that arrays referred by variables a and b are of the same size. The header of the method is as follows. public static int[] add (int[] listl, int[] list2) The add method should return an array of the same size as the input arrays, with each element to be the sum of the elements from the input arrays. For example, if the two input arrays are [3, 5, 2, 6, 4, 9] and [1, 2, 3, 4, 5, 6], the returned sum array should be [4, 7, 5, 10, 9, 15]. 4. Write a method to display an int array's contents on the console window. The header of the method is as below. public static void displayArray (int[] list) 5. Write the main method to: a. Create two input arrays with the same size by invoking the inputArray method twice in the main method. b. Calculate the average of the first int array by invoking the average method. Display the average result on the console window. c. Calculate the array sum of both input arrays by invoking the add method. d. Display the sum array by invoking the display Array method.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need help writing the code. Attatching the problem instruction and code outline and display result in the images.
![### Tutorial: Methods for Array Operations in Java
Welcome to this tutorial on creating and utilizing methods for array operations in Java. This section will guide you through writing methods that perform various operations on integer arrays.
#### 1. Method to Create an Integer Array from User Input
First, we will write a method that returns an integer array using user inputs from the console window. The method should have the following header:
```java
public static int[] inputArray(int size)
```
The formal parameter `size` is the size of the return array.
#### 2. Method to Calculate the Average of an Array
Next, we will write a method that calculates and returns the average of an array. The header for this method is:
```java
public static double average(int[] list)
```
#### 3. Method to Add Two Integer Arrays
We will create a method to add two integer arrays, given that the arrays referred to by variables `a` and `b` are of the same size. The header of the method is:
```java
public static int[] add(int[] list1, int[] list2)
```
The `add` method should return an array of the same size as the input arrays, with each element being the sum of the elements from the input arrays.
*Example*: If the two input arrays are `[3, 5, 2, 6, 4, 9]` and `[1, 2, 3, 4, 5, 6]`, the returned sum array should be `[4, 7, 5, 10, 9, 15]`.
#### 4. Method to Display the Contents of an Integer Array
We will write a method to display an integer array's contents on the console window. The header of the method is:
```java
public static void displayArray(int[] list)
```
#### 5. Writing the `main` Method
Finally, we will write the `main` method that will:
a. Create two integer arrays with the same size by invoking the `inputArray` method twice.
b. Calculate the average of the first int array by invoking the `average` method and display the result on the console window.
c. Calculate the array sum of both input arrays by invoking the `add` method.
d. Display the sum array by invoking the `displayArray` method.
By following these steps, you will](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2F96b6bd47-f082-4e40-96f7-3f6e9d353c13%2Fohxwn8n_processed.png&w=3840&q=75)
Transcribed Image Text:### Tutorial: Methods for Array Operations in Java
Welcome to this tutorial on creating and utilizing methods for array operations in Java. This section will guide you through writing methods that perform various operations on integer arrays.
#### 1. Method to Create an Integer Array from User Input
First, we will write a method that returns an integer array using user inputs from the console window. The method should have the following header:
```java
public static int[] inputArray(int size)
```
The formal parameter `size` is the size of the return array.
#### 2. Method to Calculate the Average of an Array
Next, we will write a method that calculates and returns the average of an array. The header for this method is:
```java
public static double average(int[] list)
```
#### 3. Method to Add Two Integer Arrays
We will create a method to add two integer arrays, given that the arrays referred to by variables `a` and `b` are of the same size. The header of the method is:
```java
public static int[] add(int[] list1, int[] list2)
```
The `add` method should return an array of the same size as the input arrays, with each element being the sum of the elements from the input arrays.
*Example*: If the two input arrays are `[3, 5, 2, 6, 4, 9]` and `[1, 2, 3, 4, 5, 6]`, the returned sum array should be `[4, 7, 5, 10, 9, 15]`.
#### 4. Method to Display the Contents of an Integer Array
We will write a method to display an integer array's contents on the console window. The header of the method is:
```java
public static void displayArray(int[] list)
```
#### 5. Writing the `main` Method
Finally, we will write the `main` method that will:
a. Create two integer arrays with the same size by invoking the `inputArray` method twice.
b. Calculate the average of the first int array by invoking the `average` method and display the result on the console window.
c. Calculate the array sum of both input arrays by invoking the `add` method.
d. Display the sum array by invoking the `displayArray` method.
By following these steps, you will
![## Code Implementation for Array Operations
### Objective
This educational tutorial will guide you through implementing an array manipulation program in Java. The program performs the following tasks:
1. Takes two integer arrays as input.
2. Calculates the average of the first array.
3. Adds the two arrays element-wise.
4. Displays the resulting array.
### Code Structure
Below is the skeleton code for the program. Your task is to complete the missing parts.
### Main Class
```java
public static void main(String[] args) {
int[] arr1 = inputArray(8); // Enter any input array size for testing
int[] arr2 = inputArray(8); // Enter the same input array size for testing
// Prints the average of the elements in the first array.
System.out.println("The average of the first array provided is " + /*Please write your code here*/ + ".");
// Creates an array that holds the value of the sums from adding the two integer arrays.
int[] resultingArray = add(arr1, arr2);
// Prints the array with the sum of the two added integer arrays.
displayArray(resultingArray);
}
```
### Input Array Method
```java
public static int[] inputArray(int size) {
// Creates an array of size provided.
int[] inputArr = new int[size];
// Asks user to input values for elements to be.
System.out.println("Enter " + size + " integer values to add to the array:");
// Please write your code here
return inputArr;
}
```
### Compute Average Method
```java
public static double average(int[] list) {
// Please write your code here
}
```
### Add Arrays Method
```java
public static int[] add(int[] list1, int[] list2) {
// Please write your code here
}
```
### Display Array Method
```java
public static void displayArray(int[] list) {
System.out.println("The sum resulting array is " + /*Please write your code here*/);
}
```
### Output Example
Your code's formatting should look exactly like the output mentioned below and should be able to run test cases on different values of `size`.
```
Enter 8 integer values to add to the array.
1 2 3 4 5 6 7 8
Enter 8 integer values to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2F96b6bd47-f082-4e40-96f7-3f6e9d353c13%2Fsrih48_processed.png&w=3840&q=75)
Transcribed Image Text:## Code Implementation for Array Operations
### Objective
This educational tutorial will guide you through implementing an array manipulation program in Java. The program performs the following tasks:
1. Takes two integer arrays as input.
2. Calculates the average of the first array.
3. Adds the two arrays element-wise.
4. Displays the resulting array.
### Code Structure
Below is the skeleton code for the program. Your task is to complete the missing parts.
### Main Class
```java
public static void main(String[] args) {
int[] arr1 = inputArray(8); // Enter any input array size for testing
int[] arr2 = inputArray(8); // Enter the same input array size for testing
// Prints the average of the elements in the first array.
System.out.println("The average of the first array provided is " + /*Please write your code here*/ + ".");
// Creates an array that holds the value of the sums from adding the two integer arrays.
int[] resultingArray = add(arr1, arr2);
// Prints the array with the sum of the two added integer arrays.
displayArray(resultingArray);
}
```
### Input Array Method
```java
public static int[] inputArray(int size) {
// Creates an array of size provided.
int[] inputArr = new int[size];
// Asks user to input values for elements to be.
System.out.println("Enter " + size + " integer values to add to the array:");
// Please write your code here
return inputArr;
}
```
### Compute Average Method
```java
public static double average(int[] list) {
// Please write your code here
}
```
### Add Arrays Method
```java
public static int[] add(int[] list1, int[] list2) {
// Please write your code here
}
```
### Display Array Method
```java
public static void displayArray(int[] list) {
System.out.println("The sum resulting array is " + /*Please write your code here*/);
}
```
### Output Example
Your code's formatting should look exactly like the output mentioned below and should be able to run test cases on different values of `size`.
```
Enter 8 integer values to add to the array.
1 2 3 4 5 6 7 8
Enter 8 integer values to
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
The last part of the code is not completed.
public static void displayArray(int[] list)
{
System.out.println("The sum resulting array is" + Arrays.toString(list));
}
}
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
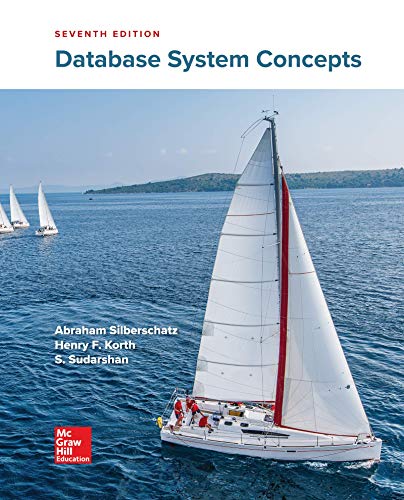
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
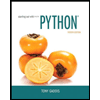
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
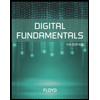
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
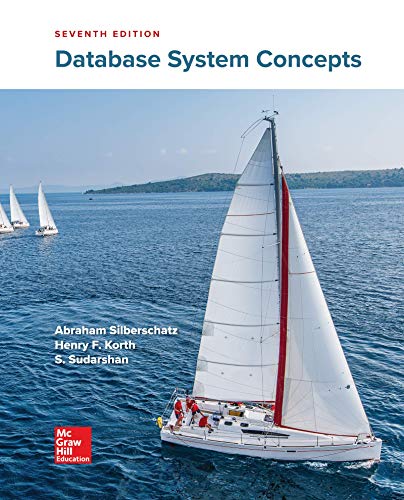
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
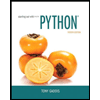
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
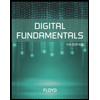
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
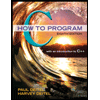
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
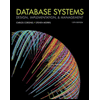
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
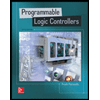
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education