The following program contains 3 files. 1) absWelcome.java public abstract class absWelcome { // abstract method : public abstract void printMessage(); // regular method : public void printWelcome() { System.out.println("Welcome to"); } } 2) clsHello.java public class clsHello extends absWelcome { // overriding the abstract method : public void printMessage() { System.out.println("Java Programming"); } } 3) Main.java public class Main { public static void main(String[] args) { // object creation : clsHello obj = new clsHello(); // using object to call methods : obj.printWelcome(); obj.printMessage(); } }
The following program contains 3 files.
1) absWelcome.java
public abstract class absWelcome {
// abstract method :
public abstract void printMessage();
// regular method :
public void printWelcome() {
System.out.println("Welcome to");
}
}
2) clsHello.java
public class clsHello extends absWelcome {
// overriding the abstract method :
public void printMessage() {
System.out.println("Java
}
}
3) Main.java
public class Main {
public static void main(String[] args) {
// object creation :
clsHello obj = new clsHello();
// using object to call methods :
obj.printWelcome();
obj.printMessage();
}
}


An interface in Java is a blueprint of a class.
The following are the properties of an interface:
- We cannot instantiate an interface.
- It does not contain any constructors.
- All of the methods in an interface are abstract.
- Interface attributes must be declared both static and final.
- An interface is implemented by a class.
- A class must implement all the methods of an interface. If it does not provide method bodies for all functions, then the class must be declared abstract.
The purpose of the interface:
- Java doesn’t support multiple inheritance. However, using interfaces, we can achieve multiple inheritance.
- Loose-Coupling: We define the method separately and the signature separately.
It provides the way for total abstraction. Abstraction is an OOP concept that hides the background details. It shows only those pieces of information to the user which are most relevant for that specific process.
Step by step
Solved in 5 steps with 1 images

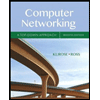
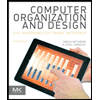
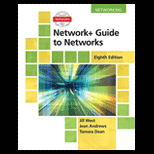
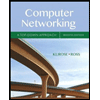
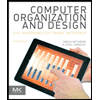
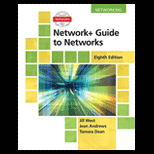
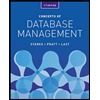
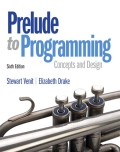
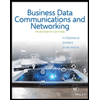