The class diagram below shows an Order class that "has a" Customer and zero or more Items. Order class should contain private Customer m_cust; private ArrayList m_items; public Order(Customer cus t) public void additem(Item i) public String toString()
The class diagram below shows an Order class that "has a" Customer and zero or more Items.
Order class should contain
private Customer m_cust;
private ArrayList<Item> m_items;
public Order(Customer cus t)
public void additem(Item i)
public String toString()
Item class should contain
private String m_desc
private int m_qty
private double m_price
public Item(String des c, double price, int qty)
public String toString()
Customer class should contain
private String m_first;
private String m_last;
public Customer(String first, String last)
public String toString()
Please create the Order, Customer, and Item classes shown above.
I have provided you with a read-only Main class that you can use to test your code.
When you have completed the Order, Customer, and Item classes, running the Main program should produce the following output:
Order for Smith, Tom
Order Items: Greeting Card: 1 at 1.5
Baseball Glove: 1 at 54.0
Notebook: 3 at 2.5
public class Main
{
public static void main(String args[])
{
Main m = new Main();
m.go();
}
public void go()
{
Customer c = new Customer("Tom", "Smith");
Order o = new Order(c);
o.addItem(new Item("Greeting Card", 1.50, 1));
o.addItem(new Item("Baseball Glove", 54.00, 1));
o.addItem(new Item("Notebook", 2.50, 3));
System.out.println(o);
}
}

Step by step
Solved in 3 steps with 1 images

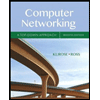
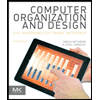
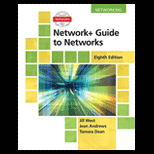
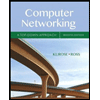
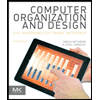
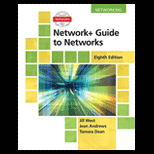
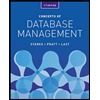
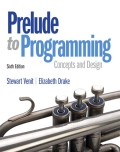
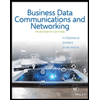