The ADT stack lets you peek at its top entry without removing it. For some applications of stacks, you also need to peek at the entry beneath the top entry without removing it. We will call such an operation peekNxt. If the stack has more than one entry, peekNxt returns the second entry from the top without altering the stack. If the stack has fewer than two entries, peekNxt throws an exception. Write a linked implementation of a stack class call LinkedStack.java that includes a method peekNxt.
The ADT stack lets you peek at its top entry without removing it. For some applications of stacks, you also need to peek at the entry beneath the top entry without removing it. We will call such an operation peekNxt. If the stack has more than one entry, peekNxt returns the second entry from the top without altering the stack. If the stack has fewer than two entries, peekNxt throws an exception. Write a linked implementation of a stack class call LinkedStack.java that includes a method peekNxt.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The ADT stack lets you peek at its top entry without removing it. For some applications of stacks, you
also need to peek at the entry beneath the top entry without removing it. We will call such an operation
peekNxt. If the stack has more than one entry, peekNxt returns the second entry from the top without altering the stack. If the stack has fewer than two entries, peekNxt throws an exception. Write a linked
implementation of a stack class call LinkedStack.java that includes a method peekNxt.
Implement p
import java.util.EmptyStackException;
import java.util.NoSuchElementException;
public final class LinkedStack<T> implements StackInterface<T>
{
private Node topNode;
public YourFirst_YourLast_LinkedStack()
{
topNode = null;
}
public void push(T newEntry)
{
Node newNode = new Node(newEntry, topNode);
topNode = newNode;
} // end push
public T peek()
{
if (isEmpty())
throw new EmptyStackException();
else
return topNode.getData();
} // end peek
public T peekNxt() // Code here
{
import java.util.EmptyStackException;
import java.util.NoSuchElementException;
public final class LinkedStack<T> implements StackInterface<T>
{
private Node topNode;
public YourFirst_YourLast_LinkedStack()
{
topNode = null;
}
public void push(T newEntry)
{
Node newNode = new Node(newEntry, topNode);
topNode = newNode;
} // end push
public T peek()
{
if (isEmpty())
throw new EmptyStackException();
else
return topNode.getData();
} // end peek
public T peekNxt() // Code here
{
}
public T pop()
{
T top = peek();
topNode = topNode.getNextNode();
return top;
}
public boolean isEmpty()
{
return topNode == null;
}
public void clear()
{
topNode = null;
}
private class Node
{
private T data;
private Node next;
private Node(T dataPortion)
{
this(dataPortion, null);
}
private Node(T dataPortion, Node linkPortion)
{
data = dataPortion;
next = linkPortion;
}
private T getData()
{
return data;
} // end getData
private void setData(T newData)
{
data = newData;
}
private Node getNextNode()
{
return next;
}
private void setNextNode(Node nextNode)
{
next = nextNode;
}
}
}
--------------------------------------
public interface StackInterface<T>
{
/** Adds a new entry to the top of this stack.
@param newEntry An object to be added to the stack. */
public void push(T newEntry);
/** Removes and returns this stack's top entry.
@return The object at the top of the stack.
@throws EmptyStackException if the stack is empty before the operation. */
public T pop();
/** Retrieves this stack's top entry.
@return The object at the top of the stack.
@throws EmptyStackException if the stack is empty. */
public T peek();
/** Retrieves the entry just below this stack's top entry.
@return The object just below the top of the stack.
@throws EmptyStackException if the stack is empty.
@throws NoSuchElementException if the stack contains only one entry.
*/
public T peekNxt();
/** Detects whether this stack is empty.
@return True if the stack is empty. */
public boolean isEmpty();
/** Removes all entries from this stack. */
public void clear();
}
this(dataPortion, null);
}
private Node(T dataPortion, Node linkPortion)
{
data = dataPortion;
next = linkPortion;
}
private T getData()
{
return data;
} // end getData
private void setData(T newData)
{
data = newData;
}
private Node getNextNode()
{
return next;
}
private void setNextNode(Node nextNode)
{
next = nextNode;
}
}
}
--------------------------------------
public interface StackInterface<T>
{
/** Adds a new entry to the top of this stack.
@param newEntry An object to be added to the stack. */
public void push(T newEntry);
/** Removes and returns this stack's top entry.
@return The object at the top of the stack.
@throws EmptyStackException if the stack is empty before the operation. */
public T pop();
/** Retrieves this stack's top entry.
@return The object at the top of the stack.
@throws EmptyStackException if the stack is empty. */
public T peek();
/** Retrieves the entry just below this stack's top entry.
@return The object just below the top of the stack.
@throws EmptyStackException if the stack is empty.
@throws NoSuchElementException if the stack contains only one entry.
*/
public T peekNxt();
/** Detects whether this stack is empty.
@return True if the stack is empty. */
public boolean isEmpty();
/** Removes all entries from this stack. */
public void clear();
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
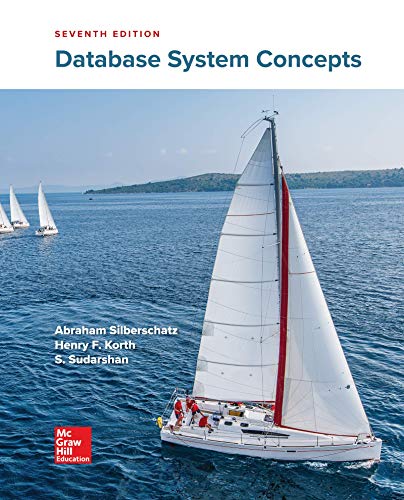
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
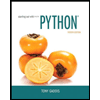
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
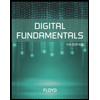
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
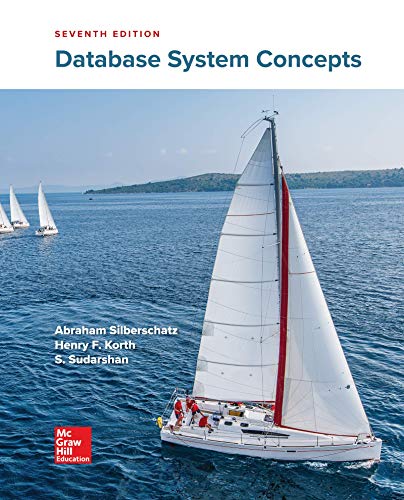
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
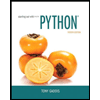
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
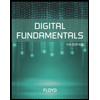
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
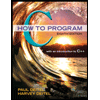
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
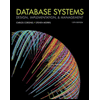
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
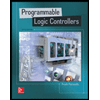
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education