The calculator works as follows: if number, push to a stack if operator, pop two numbers, operate on them, and push the result if I hit an operator and there are not two numbers on the stack, it's a bad expression if I get to the end of the expression and there is not exactly one number on the stack, it's a bad expression. YOUR IMPLEMENTATION, 30 points: 10 - the input to output happening without a crash 10 for implementing LinkedListBasedStack 10 for implementing ArrayList YOUR CALCULATOR ONLY NEEDS TO WORK FOR +, -, and * YOUR CALCULATOR ONLY NEEDS TO WORK ON INTEGERS. RPNStringTokenizer.java package template; import java.util.ArrayList; public interface RPNStringTokenizer { public static ArrayList tokenize (String expression) { // take a string. If it is valid RPN stuff - integers or operators // - then put them in a list for processing. // anything bad (not int or one of the operators we like) and return a null. // YOU WRITE THIS! return null; } } RPNTester.java package template; import java.util.ArrayList; public class RPNTester { public static void main(String[] args) { // don't change this method! YourRPNCalculator calc = new YourRPNCalculator(new YourArrayListStack()); System.out.println("Testing ArrayList version"); testRPNCalculator(calc); calc = new YourRPNCalculator(new YourLinkedListStack()); System.out.println("Testing LinkedList version"); testRPNCalculator(calc); } private static void testRPNCalculator(SimpleRPNCalculator calc) { ArrayList testExpressions = new ArrayList<>(); testExpressions.add("1 1 +"); // 2 testExpressions.add("1 3 -"); // 2 testExpressions.add("1 1 + 2 *"); // 4 testExpressions.add("1 1 2 + *"); // 3 testExpressions.add("1 1 + 2 2 * -"); // 2 testExpressions.add("11 bv +"); // bad token testExpressions.add("2 3 + -"); // underflow on an operator testExpressions.add("2 3 + 4 5 -"); // leftover tokens // YOU SHOULD ADD MORE TEST CASES! for (String s : testExpressions) { System.out.println(calc.calculate(s)); } } } SimpleRPNCalculator.java package template; public interface SimpleRPNCalculator { public String calculate(String inputString); /* * RPN (Reverse Polish Notation) is a postfix method of expression mathematical functions. * It is traditionally used to eliminate the necessity of parenthesis by removing the * order of operations and acting on operators as they occur. * * Very simply, INFIX notation "1 + 1" is represented in POSTFIX notation as "1 1 +" * Longer expressions may change radically. "1 + 2 * 3" assumes an order of operations, * while in POSTFIX, "2 3 * 1 +" or "1 2 3 * +" * * The calculator works as follows: * if number, push to a stack * if operator, pop two numbers, operate on them, and push the result * if I hit an operator and there are not two numbers on the stack, it's a bad expression * if I get to the end of the expression and there is not exactly one number on the stack, * it's a bad expression. * * FOR YOUR IMPLEMENTATION, 30 points: * 10 for making something that looks like it works - the input to output happening without a crash * 10 for implementing LinkedListBasedStack * 10 for implementing ArrayList * * YOUR CALCULATOR ONLY NEEDS TO WORK FOR +, -, and * * YOUR CALCULATOR ONLY NEEDS TO WORK ON INTEGERS. * * 5 bonus points for working on Doubles (this is a gimme) * 5 bonus points for implementing division / (this is deceptively hard, DO THIS LAST!) * * */ } YourArrayListStack.java package template; import java.util.ArrayList; public class YourArrayListStack implements YourStack { // YOU MUST USE THIS IMPLEMENTATION - just code the methods ArrayList theStack = new ArrayList<>(); @Override public void push(Integer i) { } @Override public Integer pop() { return null; } @Override public Integer size() { return null; } } YourLinkedListStack.java package template; public class YourLinkedListStack implements YourStack { // YOU MUST USE THIS IMPLEMENTATION - just code the methods private YourStackNode head = null; private Integer size = 0; @Override public void push(Integer i) { } @Override public Integer pop() { return null; } @Override public Integer size() { return null; } } YourRPNCalculator.java package template; import java.util.ArrayList; public class YourRPNCalculator implements SimpleRPNCalculator { // don't change these... YourStack theStack = null; public YourRPNCalculator(YourStack stack) { theStack = stack; } @Override public String calculate(String inputString) { // this is probably helpful, but you can remove... ArrayList tokens = RPNStringTokenizer.tokenize(inputString); // here's the calculator logic! return null; } } YourStack.java package template; public interface YourStack { public void push (Integer i); public Integer pop(); public Integer size(); } YourStackNode.java package template; public class YourStackNode { // DO NOT CHANGE THIS! private Integer item; private YourStackNode next; public Integer getItem() { return item; } public YourStackNode getNext() { return next; } public void setItem (Integer i) { item = i; } public void setNext (YourStackNode node) { next = node; } }
package template;
import java.util.ArrayList;
public interface RPNStringTokenizer {
public static ArrayList<String> tokenize (String expression) {
// take a string. If it is valid RPN stuff - integers or operators
// - then put them in a list for processing.
// anything bad (not int or one of the operators we like) and return a null.
// YOU WRITE THIS!
return null;
}
}
package template;
import java.util.ArrayList;
public class RPNTester {
public static void main(String[] args) {
// don't change this method!
YourRPNCalculator calc = new YourRPNCalculator(new YourArrayListStack());
System.out.println("Testing ArrayList version");
testRPNCalculator(calc);
calc = new YourRPNCalculator(new YourLinkedListStack());
System.out.println("Testing LinkedList version");
testRPNCalculator(calc);
}
private static void testRPNCalculator(SimpleRPNCalculator calc) {
ArrayList<String> testExpressions = new ArrayList<>();
testExpressions.add("1 1 +"); // 2
testExpressions.add("1 3 -"); // 2
testExpressions.add("1 1 + 2 *"); // 4
testExpressions.add("1 1 2 + *"); // 3
testExpressions.add("1 1 + 2 2 * -"); // 2
testExpressions.add("11 bv +"); // bad token
testExpressions.add("2 3 + -"); // underflow on an operator
testExpressions.add("2 3 + 4 5 -"); // leftover tokens
// YOU SHOULD ADD MORE TEST CASES!
for (String s : testExpressions) {
System.out.println(calc.calculate(s));
}
}
}
package template;
public interface SimpleRPNCalculator {
public String calculate(String inputString);
/*
* RPN (Reverse Polish Notation) is a postfix method of expression mathematical functions.
* It is traditionally used to eliminate the necessity of parenthesis by removing the
* order of operations and acting on operators as they occur.
*
* Very simply, INFIX notation "1 + 1" is represented in POSTFIX notation as "1 1 +"
* Longer expressions may change radically. "1 + 2 * 3" assumes an order of operations,
* while in POSTFIX, "2 3 * 1 +" or "1 2 3 * +"
*
* The calculator works as follows:
* if number, push to a stack
* if operator, pop two numbers, operate on them, and push the result
* if I hit an operator and there are not two numbers on the stack, it's a bad expression
* if I get to the end of the expression and there is not exactly one number on the stack,
* it's a bad expression.
*
* FOR YOUR IMPLEMENTATION, 30 points:
* 10 for making something that looks like it works - the input to output happening without a crash
* 10 for implementing LinkedListBasedStack
* 10 for implementing ArrayList
*
* YOUR CALCULATOR ONLY NEEDS TO WORK FOR +, -, and *
* YOUR CALCULATOR ONLY NEEDS TO WORK ON INTEGERS.
*
* 5 bonus points for working on Doubles (this is a gimme)
* 5 bonus points for implementing division / (this is deceptively hard, DO THIS LAST!)
*
*
*/
}
package template;
import java.util.ArrayList;
public class YourArrayListStack implements YourStack {
// YOU MUST USE THIS IMPLEMENTATION - just code the methods
ArrayList<Integer> theStack = new ArrayList<>();
@Override
public void push(Integer i) {
}
@Override
public Integer pop() {
return null;
}
@Override
public Integer size() {
return null;
}
}
package template;
public class YourLinkedListStack implements YourStack {
// YOU MUST USE THIS IMPLEMENTATION - just code the methods
private YourStackNode head = null;
private Integer size = 0;
@Override
public void push(Integer i) {
}
@Override
public Integer pop() {
return null;
}
@Override
public Integer size() {
return null;
}
}
package template;
import java.util.ArrayList;
public class YourRPNCalculator implements SimpleRPNCalculator {
// don't change these...
YourStack theStack = null;
public YourRPNCalculator(YourStack stack) {
theStack = stack;
}
@Override
public String calculate(String inputString) {
// this is probably helpful, but you can remove...
ArrayList<String> tokens = RPNStringTokenizer.tokenize(inputString);
// here's the calculator logic!
return null;
}
}
package template;
public interface YourStack {
public void push (Integer i);
public Integer pop();
public Integer size();
}
package template;
public class YourStackNode {
// DO NOT CHANGE THIS!
private Integer item;
private YourStackNode next;
public Integer getItem() {
return item;
}
public YourStackNode getNext() {
return next;
}
public void setItem (Integer i) {
item = i;
}
public void setNext (YourStackNode node) {
next = node;
}
}

We have to write the code of given data we have to complete the given code by using the given data. We will write two classes implementing the YourStack interface using an ArrayList and a linked list. we have to create YourArrayListStack, YourLinkedListStack and RPNStringTokenizer.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

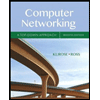
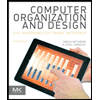
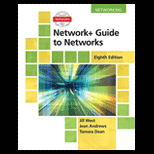
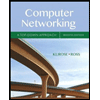
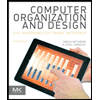
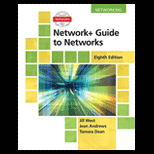
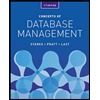
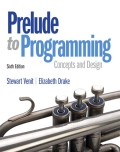
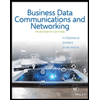