Tests that FindText() returns 5 for parameters "more" and "We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue!" Test feedback FindText() incorrectly returns 0."
I wrote this program, but the solution on zybooks didn't let it full credit.
It showed:
#include <iostream>
#include <string>
using namespace std;
//Function prototype
char PrintMenu(string&);
int GetNumOfNonWSCharacters(string);
int GetNumOfWords(const string);
int FindText(string, string);
void ReplaceExclamation(string&);
void ShortenSpace(string&);
int main() {
/* Type your code here. */
//Declare variables
char option;
string text, phraseToFind;
//Get text from user
cout << "Enter a sample text:\n";
getline (cin, text);
//Print that text
cout << "\nYou entered: " << text;
//Loop until user type q - Quit
do {
option = PrintMenu(text);
} while (option != 'q');
return 0;
}
/*Print out the menu*/
char PrintMenu(string& text) {
char ch;
string phraseToFind;
cout << "\n\nMENU\n"
<< "c - Number of non-whitespace characters\n"
<< "w - Number of words\n"
<< "f - Find text\n"
<< "r - Replace all !'s\n"
<< "s - Shorten spaces\n"
<< "q - Quit\n"
<< "\nChoose an option:\n";
cin >> ch;
switch (ch) {
//User types q - quit the program
case 'q':
exit(0);
//User types c - count non-whitespace characters
case 'c':
cout << "Number of non-whitespace characters: "
<< GetNumOfNonWSCharacters(text);
break;
//User types w - count number of words
case 'w':
cout << "Number of words: "
<< GetNumOfWords(text);
break;
//User types f - find a word into the string that enter before
case 'f':
cin.ignore();
cout << "Enter a word or phrase to be found:\n";
getline(cin, phraseToFind);
cout << "\"" << phraseToFind << "\""
<< " instances: " << FindText(text, phraseToFind);
break;
//User types r - replace all ! by .
case 'r':
ReplaceExclamation(text);
cout << "Edited text: " << text;
break;
//User types s - remove multiple spaces by single space
case 's':
ShortenSpace(text);
cout << "Edited text: " << text;
break;
default:
break;
}//end switch
return ch;
}//PrintMenu
/*Count number of non-whitespace characters - Option c*/
int GetNumOfNonWSCharacters(const string text) {
int count = 0;
int length = text.size();
for (int i = 0; i < length; i++) {
if (!isspace(text[i]))
count++;
}
return count;
}//GetNumOfNonWSCharacters
/*Count number of words - Option w*/
int GetNumOfWords(const string text) {
int count = 1;
for (long unsigned int i = 1; i < text.size() - 1; i++) {
if (text.at(i) == ' ' && text.at(i - 1) != ' ') {
count++;
}
}
return count;
}//GetNumOfWords
/*Find a word into the string that enter before - Option f*/
int FindText(string text, string phrase) {
int count = 0;
long unsigned int i, j;
for (i = 0; i < text.size(); i++) {
j = 0;
if (text[i] == phrase[j]) {
while (text[i] == phrase[j] && j < phrase.size()) {
j++;
i++;
}
if (j == phrase.size()) {
count++;
}
}
}
return count;
}//FindText
/*Replace ! with . - Option r*/
void ReplaceExclamation(string& text) {
string newText = text;
int length = text.size();
for (int i = 0; i < length; i++) {
if (text[i] == '!')
newText[i] = '.';
}
text = newText;
}//ReplaceExclamation
/*Replace multiple spaces with single space - Option s*/
void ShortenSpace(string &text) {
int i, len = text.size();
string newText = "";
for (i = 0; i < len; i++) {
if (isspace(text[i])) {
while (isspace(text[i]))
i++;
i--;
newText = newText + " ";
}
else {
newText = newText + text[i];
}
}
text = newText;
}//ShortenSpace

Here I'm attaching the whole working code: find text was not implmented
#include<iostream>
#include<bits/stdc++.h>
using namespace std;
//Function prototypes
char PrintMenu(string);
int GetNumOfNonWSCharacters(string);
int GetNumOfWords(string);
void ReplaceExclamation(string &);
void ShortenSpace(string &);
int FindText(string, string);
//Main function
int main()
{
//variable decalaration
char option;
string text, phraseToFind;
//Reading text from user
cout << "Enter a sample text:" << endl;
getline(cin, text);
//Printing text
cout << "\nYou entered: " << text<<endl;
//Loop till user wants to quit
do
{
//Printing menu
option = PrintMenu(text);
cout << "";
}
while (option != 'Q' && option != 'q');
//system("pause");
return 0;
}
//Function that prints menu
char PrintMenu(string text)
{
char ch;
string phraseToFind;
//Printing menu
cout << "\nMENU";
cout << "\nc - Number of non-whitespace characters\nw - Number of words\nf - Find text\nr - Replace all !'s\ns - Shorten spaces\nq - Quit"<
cout << "\nChoose an option:"<<endl;
//Reading user choice
cin >> ch;
//Calling functions based on option selected by //user
switch (ch)
{
//User wants to quit
case 'q':
case 'Q':
exit(0);
// break; //try this if exit(0) does not work.
//Counting non-whitespace characters
case 'c':
case 'C':
cout << "\nNumber of non-whitespace characters: " << GetNumOfNonWSCharacters(text) << "\n";
break;
//Counting number of words
case 'w':
case 'W':
cout << "\nNumber of words: " << GetNumOfWords(text) << "\n";
break;
//Counting number of occurrences phrase in //given string
case 'f':
case 'F':
cin.ignore();
cout << "\nEnter a word or phrase to be found:";
getline(cin, phraseToFind);
cout << "\n\"" << phraseToFind << "\" instances: " << FindText(text, phraseToFind) << "\n";
break;
//Replacing ! with .
case 'r':
case 'R':
ReplaceExclamation(text);
cout << "\nEdited text: " << text << "\n";
break;
//Replacing multiple spaces with single //space
case 's':
case 'S':
ShortenSpace(text);
cout << "\n Edited text: " << text << "\n";
break;
default:
cout << "\n\n Invalid Choice.... Try Again \n";
break;
}
return ch;
}
//Function that count number of non space characters
int GetNumOfNonWSCharacters(const string text)
{
int cnt = 0, i;
int len = text.size();
//Looping over given text
for (i = 0; i<len; i++)
{
//Counting spaces
if (!isspace(text[i]))
cnt++;
}
return cnt;
}
//Function that count number of words in the string
int GetNumOfWords(const string text)
{
int words = 0, i;
int len = text.size();
//Looping over text
for (i = 0; i < len; i++)
{
//Checking for space
if (isspace(text[i]))
{
//Handling multiple spaces
while (isspace(text[i]))
i++;
//Incrementing words
i--;
words++;
}
}
//Handling last word
words = words + 1;
return words;
}
//Function that replaces ! with .
void ReplaceExclamation(string &text)
{
string newText = text;
int i, len = text.size();
//Looping over string
for (i = 0; i < len; i++)
{
//Replacing ! with .
if (text[i] == '!')
newText[i] = '.';
}
text = newText;
}
//Function that replaces Multiple spaces with single space
void ShortenSpace(string &text)
{
int i, len = text.size(), k = 0;
string newText = "";
//Looping over string
for (i = 0; i < len; i++)
{
//Assign individual characters
//Handling multiple spaces
if (isspace(text[i]))
{
//Replacing multiple spaces with single //space
while (isspace(text[i]))
i++;
i--;
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

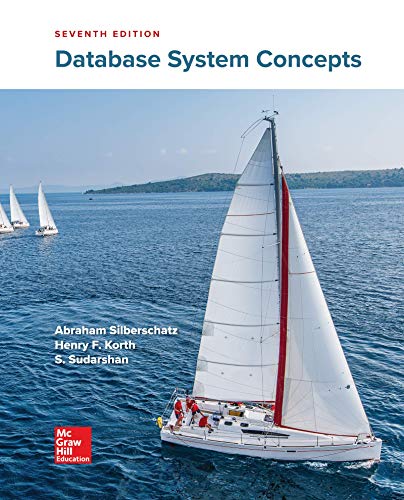
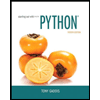
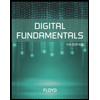
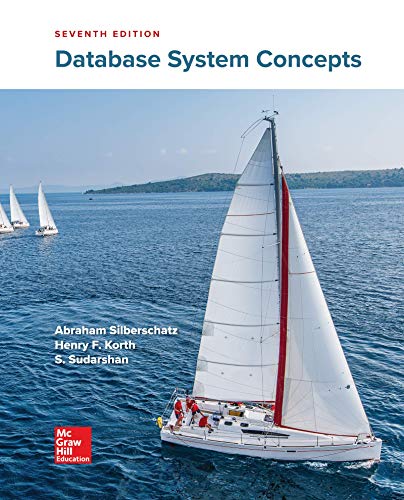
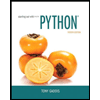
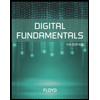
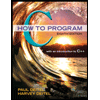
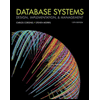
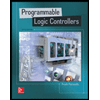