t employees by rate of pay from high to low. •returns the average earnings of all employees •performs the search for a specific employee and returns the employee ID if found and 0 if not. •test the functions in the main function #include #include #include using name
I need help with:
•sort employees by rate of pay from high to low.
•returns the average earnings of all employees
•performs the search for a specific employee and returns the employee ID if found and 0 if not.
•test the functions in the main function
#include <iostream>
#include <iomanip>
#include <string>
using namespace std;
class Employee
{
private:
int empNumber; // Employee number
string name; // Employee name
double hours, // Hours worked
payRate; // Hourly pay rate
public:
Employee(int empN, string n, double h, double p)
{
empNumber = empN;
name = n;
hours = h;
payRate = p;
}
Employee()
{
empNumber = 0;
name = "No Name";
hours = 0;
payRate = 0;
}
void setEmpNumber(int number)
{
empNumber = number;
}
void setName(string n)
{
name = n;
}
void setPayRate(double rate)
{
payRate = rate;
}
void setHours(double h)
{
hours = h;
}
int getEmpNumber()
{
return empNumber;
}
string getName()
{
return name;
}
double getHours()
{
return hours;
}
double getPayRate()
{
return payRate;
}
double getWage()
{
return payRate * hours;
}
string getEmpData()
{
string data = "\nEmployee ID Number ===> " + to_string(empNumber);
data += "\nEmployee Name ========> " + name;
data += "\nHours Worked =========> " + to_string(hours);
data += "\nPay Rate =============> " + to_string(payRate);
data += "\nTotal Wage ===========> " + to_string(getWage());
data += "\n";
return data;
}
};
Employee findHigh(Employee list[], int size)
{
Employee high = list[0];
for (int i = 0; i < size; i++)
if (list[i].getWage() > high.getWage())
{
high = list[i];
}
return high;
}
Employee findLow(Employee list[], int size)
{
Employee low = list[0];
for (int i = 0; i < size; i++)
if (list[i].getWage() < low.getWage())
{
low = list[i];
}
return low;
}
void sortEmployeesAZ(Employee list[], int size)
{
Employee emp;
for (int i = 0; i < size - 1; i++)
for (int j = i + 1; j < size; j++)
if (list[i].getName() > list[j].getName())
{
emp = list[i];
list[i] = list[j];
list[j] = emp;
}
}
void printList(Employee list[], int size){
for (int i = 0; i < size; i++)
{
cout << list[i].getEmpData();
}
}
//sort employees by rate of pay from high to low.
void sortEmployeesHighLowRate(Employee list[], int size)
{
//put code here
}
// here, code returns of the average earnings of all employees
double averageWage(Employee list[], int size)
{
//code hereee
return 0;
}
// code something that performs the search for a specific employee and returns the employee ID if found and 0 if not.
int findEmployeeById(Employee list[], int size, int myId)
{
//code
return 0;
}
int main()
{
const int NUM_EMPLOYEES = 4;
Employee list[NUM_EMPLOYEES];
list[0] = Employee(1, "John", 40, 25);
list[1] = Employee(2, "Mary", 30, 40);
list[2] = Employee(3, "Grace", 35, 30);
list[3] = Employee(4, "Mark", 45, 20);
Employee x = findLow(list, NUM_EMPLOYEES);
std::cout << "Lowest wage:" << x.getEmpData() << std::endl;
x = findHigh(list, NUM_EMPLOYEES);
std::cout << "Highest wage:" << x.getEmpData() << std::endl;
printList(list, NUM_EMPLOYEES);
sortEmployeesAZ(list, NUM_EMPLOYEES);
cout << "\n++++++++++++++ Sorted by name ++++++++++++++++++++" << endl;
printList(list, NUM_EMPLOYEES);
//and here is testing code
/////////////////////////////////
return 0;
}

Step by step
Solved in 4 steps with 2 images

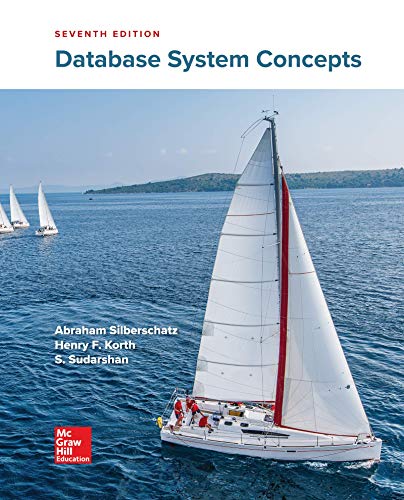
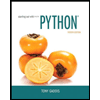
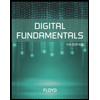
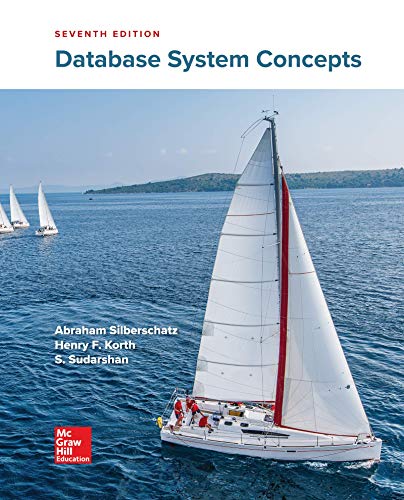
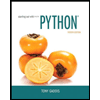
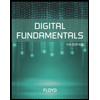
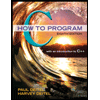
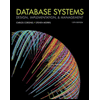
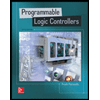