Suppose we number the bytes in a w-bit word from 0 (least significant) to w/8 – 1 (most significant). Write code for the following C function, which will return an unsigned value in which byte i of argument x has been replaced by byte b: unsigned replace_byte (unsigned x, int i, unsigned char b); Here are some examples showing how the function should work: replace_byte(0x12345678, 2, 0xAB) --> 0x12AB5678 replace_byte(0x12345678, 0, 0xAB) --> 0x123456AB Bit-Level Integer Coding Rules In several of the following problems, we will artificially restrict what programming constructs you can use to help you gain a better understanding of the bit-level, logic, and arithmetic operations of C. In answering these problems, your code must follow these rules:
Suppose we number the bytes in a w-bit word from 0 (least significant) to w/8 – 1 (most significant). Write code for the following C function, which will return an unsigned value in which byte i of argument x has been replaced by byte b:
unsigned replace_byte (unsigned x, int i, unsigned char b);
Here are some examples showing how the function should work:
replace_byte(0x12345678, 2, 0xAB) --> 0x12AB5678
replace_byte(0x12345678, 0, 0xAB) --> 0x123456AB
Bit-Level Integer Coding Rules
In several of the following problems, we will artificially restrict what
- Allowed operations
- All bit-level and logic operations.
- Left and right shifts, but only with shift amounts between 0 and w – 1.
- Addition and subtraction.
- Equality (==) and inequality (!=) tests. (Some of the problems do not allow these.)
- Integer constants INT_MIN and INT_MAX.
- Casting between data types int and unsigned, either explicitly or implicitly.
Even with these rules, you should try to make your code readable by choosing descriptive variable names and using comments to describe the logic behind your solutions. As an example, the following code extracts the most significant byte from integer argument x:
/* Get most significant byte from x */
int get_msb(int x) {
/* Shift by w-8 */
int shift_val = (sizeof(int)-1)<<3;
/* Arithmetic shift */
int xright = x >> shift_val;
/* Zero all but LSB */
return xright & 0xFF;
}
C source code . Write a short C-program, include the function named replace_byte and a call to that function. In the call to the function supply an integer as a test value.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

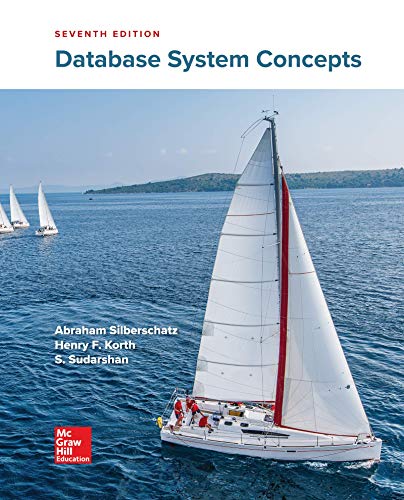
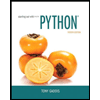
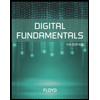
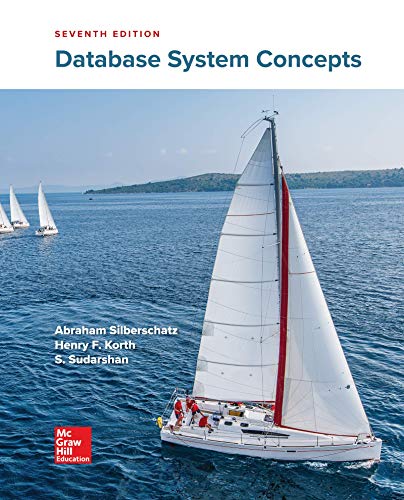
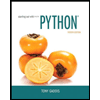
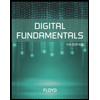
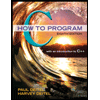
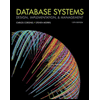
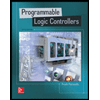