Write a C function that is given a pointer to memory, the number of bytes of memory the pointer points at, and two pointers to uint32_t. Your job is to treat the pointer as if it were a pointer to an array of uint32_t, find the min and max values of the array, and write the results to the locations pointed at by the uint32_t pointers. The number of bytes will be a positive multiple of 4. Do not worry about endianess in this problem.
Write a C function that is given a pointer to memory, the number of bytes of memory the pointer points at, and two pointers to uint32_t. Your job is to treat the pointer as if it were a pointer to an array of uint32_t, find the min and max values of the array, and write the results to the locations pointed at by the uint32_t pointers. The number of bytes will be a positive multiple of 4. Do not worry about endianess in this problem.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a C function that is given a pointer to memory, the number of bytes of memory the pointer points at, and two pointers to uint32_t. Your job is to treat the pointer as if it were a pointer to an array of uint32_t, find the min and max values of the array, and write the results to the locations pointed at by the uint32_t pointers.
The number of bytes will be a positive multiple of 4. Do not worry about endianess in this problem.
Note the tester has command line options -Wall -Wextra -Werror -fsanitize=address to help you catch errors.

Transcribed Image Text:code.co
+ New
Exit Full Screen *
1 #include <stdint.h>
2
3 // Treat ptr as base address of a uint32_t array and place the min and
4 // max array values in memory where min and max point.
5 // num_bytes is a multiple of 4.
6 - void min_max(void *ptr, int num_bytes, uint32_t *min, uint32_t *max) {
uint32_t *ptr32 = (uint32_t *)ptr;
// your code goes here
9 }
10|
7
8
Expert Solution

Step 1
Code:
#include<stdio.h>
#include<stdint.h>
//Treat ptr as the base address of a uint32_t array and place the min and
//max array values in memory where min and max point.
//num_bytes is a multiple of 4.
void min_max(void *ptr, int num_bytes, uint32_t *min, uint32_t *max)
{
uint32_t *ptr32 = (uint32_t *)ptr;
//Looping to access array elemnts using pointer
//to find minimum and maximum values of array and
//place them in memory where min and max points
for(int i=0;i<(num_bytes/4);i++)
{
//If value at pointer of array is greater than value at memory location of max
//Then assign value at pointer of array to memory location of max
if(ptr32[i]>*max)
{
*max =ptr32[i];
}
//If the value at the pointer of array is less than value at memory location of min
//Then assign value at pointer of array to memory location of min
if (ptr32[i]< *min)
{
*min = ptr32[i];
}
}
}
int main()
{
//Declare variables;
int arr[10] ;
uint32_t min = 4294967295; //Set min value to (2 power 32)-1
uint32_t max = 0; //set max value to 0
//Read 10 array elements
printf("Enter 10 Array Elements: ");
for(int i=0;i<10;i++)
{
scanf("%d",&arr[i]);
}
//Declare a void pointer and assign array to it
void *ptr =arr;
//call min_max function
min_max(ptr,sizeof(arr),&min,&max);
//Display The MIN and MAX values of uint32_t array
printf("\nMIN Value in uint32 Array: %lu",min);
printf("\nMAX Value in uint32 Array: %lu", max);
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
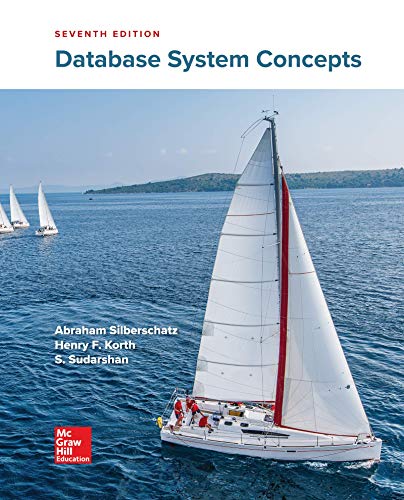
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
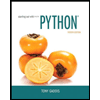
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
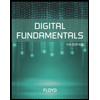
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
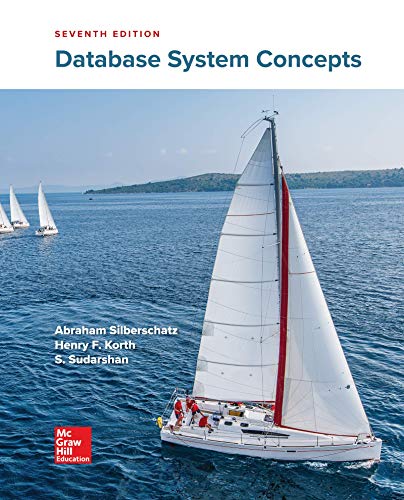
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
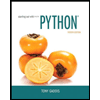
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
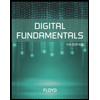
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
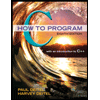
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
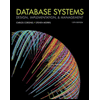
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
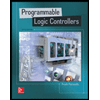
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education