a. Write a function countBits(int) that counts the number of bits that are 1. For example countBits(9) should return 2 because 9 = 1001 while countBits(16) should return 1 (10000 has one 1-bit).
a. Write a function countBits(int) that counts the number of bits that are 1. For example countBits(9) should return 2 because 9 = 1001 while countBits(16) should return 1 (10000 has one 1-bit).
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![Computation
a. Write a function countBits(int) that counts the number of bits that are 1. For example
countBits(9) should return 2 because 9 = 1001 while countBits(16) should return 1
(10000 has one 1-bit).
b. Write a function polynomial that takes an array of double, a length, and x as shown:
double c[] = {3.0, 1.5, 1.0, -2.5);
double y = polynomial(c, 4, 2.0);
should compute the polynomial 3.0x + 1.5x² + 1.0x - 2.5
Note that the efficient way to do this is Horner's form:
((3.0 * x + 1.5) * x+ 1.0) * x - 2.5
You must use a loop, not hardcode it. Your code should work for.
double c[] = {5.0, 1.0, 2.0, 3.5, 5.2};
cout << polynomial(c, 5. 2.2):
c. Given the following 2-dimensional matrix, write loops to print out the sum of every row
then column:
constexpr int rows = 3, cols = 4;
double x[rows][cols)] = {
{1, 2, 3.5},
(2. -1, 1.5}
}:
Your loop should compute 1+2+3.5 = 6.5 and 2-1+1.5 = 2.5 for the two rows, then for the
columns: 1+2 = 3, 2-1 = 1, and 3.5+1.5 = 5.
The output of the program should be:
6.5 2.5
315
d. Write a template function that sums and prints out the arrays in the following main:
int main() {
string a[] = {"yo", "ho"};
int b[] = {1, 2, 3}:
cout << sum(a, 2) << 'n';
cout << sum(b, 3) < 'n';
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbcfb8dea-921b-49cc-9763-7233db60feda%2F3c326b93-0ef9-4bbd-a841-3019a96a15e0%2F7idcqkb_processed.png&w=3840&q=75)
Transcribed Image Text:Computation
a. Write a function countBits(int) that counts the number of bits that are 1. For example
countBits(9) should return 2 because 9 = 1001 while countBits(16) should return 1
(10000 has one 1-bit).
b. Write a function polynomial that takes an array of double, a length, and x as shown:
double c[] = {3.0, 1.5, 1.0, -2.5);
double y = polynomial(c, 4, 2.0);
should compute the polynomial 3.0x + 1.5x² + 1.0x - 2.5
Note that the efficient way to do this is Horner's form:
((3.0 * x + 1.5) * x+ 1.0) * x - 2.5
You must use a loop, not hardcode it. Your code should work for.
double c[] = {5.0, 1.0, 2.0, 3.5, 5.2};
cout << polynomial(c, 5. 2.2):
c. Given the following 2-dimensional matrix, write loops to print out the sum of every row
then column:
constexpr int rows = 3, cols = 4;
double x[rows][cols)] = {
{1, 2, 3.5},
(2. -1, 1.5}
}:
Your loop should compute 1+2+3.5 = 6.5 and 2-1+1.5 = 2.5 for the two rows, then for the
columns: 1+2 = 3, 2-1 = 1, and 3.5+1.5 = 5.
The output of the program should be:
6.5 2.5
315
d. Write a template function that sums and prints out the arrays in the following main:
int main() {
string a[] = {"yo", "ho"};
int b[] = {1, 2, 3}:
cout << sum(a, 2) << 'n';
cout << sum(b, 3) < 'n';
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
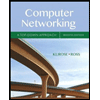
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
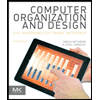
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
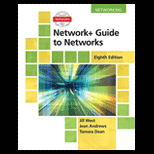
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
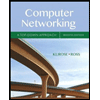
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
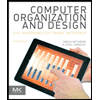
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
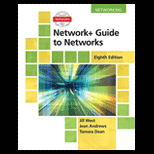
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
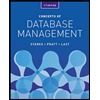
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
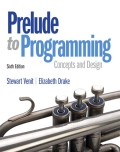
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
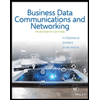
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY