Write a program whose input is two integers and whose output is the two integers swapped. Ex: If the input is: 3 8 the output is: 8 3 Your program must define and call the following function. swap_values() returns the two values in swapped order. def swap_values(user_val1, user_val2)
Write a program whose input is two integers and whose output is the two integers swapped.
Ex: If the input is:
3 8
the output is:
8 3
Your program must define and call the following function. swap_values() returns the two values in swapped order.
def swap_values(user_val1, user_val2)



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Still unsuccessful
# Define function
def swap_values(user_val1,user_val2):
# Perform swap
temp = user_val1
user_val1 = user_val2
user_val2 = temp
# Returned numbers
return user_val1,user_val2
# User input first number
num1 = input("Enter first number: ")
# User input second number
num2 = input("Enter Second Number: ")
# Call the function
user_val1,user_val2 = swap_values(num1,num2)
# Print the returned number
print(user_val1,user_val2)
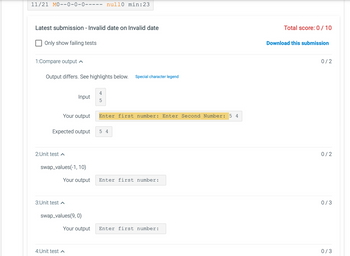
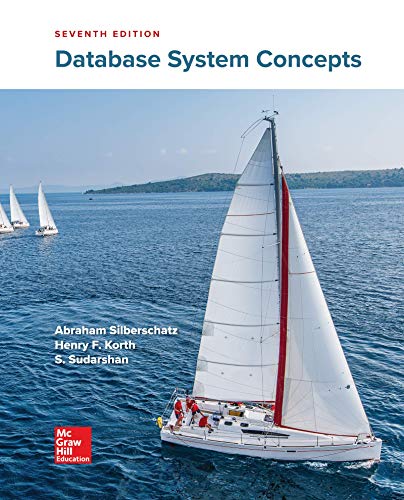
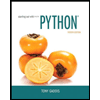
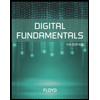
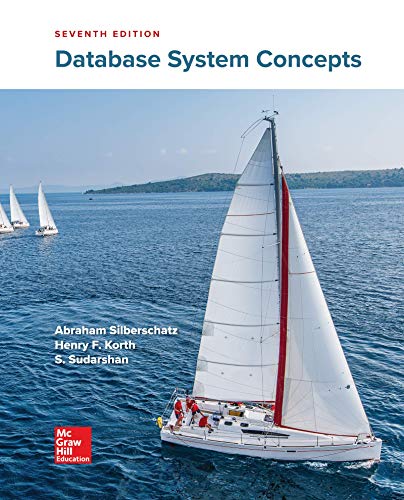
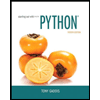
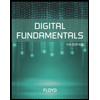
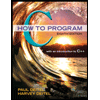
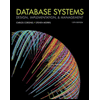
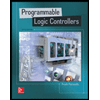