Suppose we have to do m inserts and n deletions, starting from an empty heap. When would it be more beneficial to use a d-heap rather than a binary heap?
Suppose we have to do m inserts and n deletions, starting from an empty heap. When would it be more beneficial to use a d-heap rather than a binary heap?

1) A binary heap is a binary tree that satisfies the heap property, which means that the value of each node is greater than or equal to the values of its children.
2) In a binary heap, the root node is always the smallest element, so the extract-minimum operation involves removing the root node and restoring the heap property by moving the last element in the heap to the root and then down-heapifying.
3) A d-heap is a generalization of a binary heap in which each node has d children instead of 2.
4) In a d-heap, the root node is still the smallest element, and the extract-minimum operation involves removing the root node and restoring the heap property by replacing the root with the smallest child, and then down-heapifying.
5) The advantage of a d-heap over a binary heap is that insertions and deletions can be faster, since they only require traversing a path of length O(log_d n) from the root to the leaf where the new element should be inserted or deleted. This is faster than the O(log_2 n) path length in a binary heap. However, the extract-minimum operation can be slower in a d-heap, since it requires scanning d children to find the smallest one, which takes O(d) time.
Step by step
Solved in 2 steps

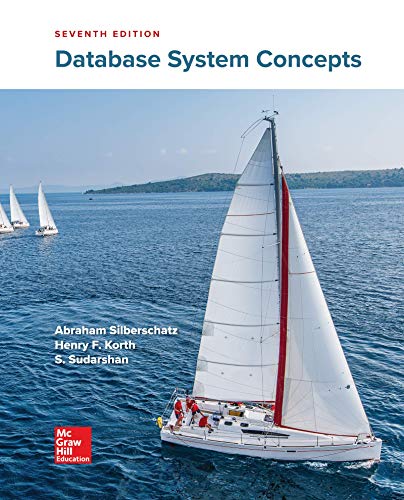
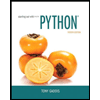
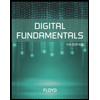
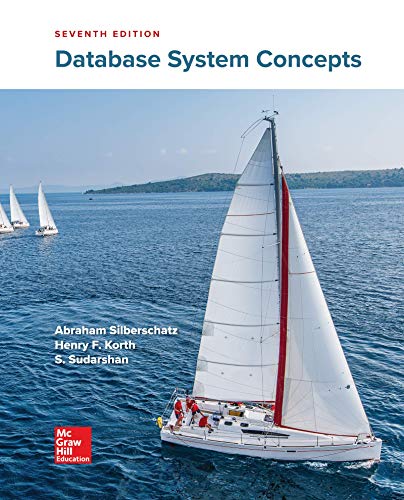
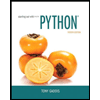
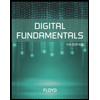
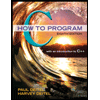
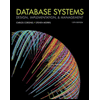
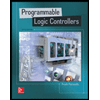