(Subclasses of Account) In Programming Exercise 9.7 - see the textbook, the Account class was defined to model a bank account. An account has the properties account number, balance, annual interest rate, and date created, and methods to deposit and withdraw funds. Create two subclasses for checking and saving accounts. A checking account has an overdraft limit, but a savings account cannot be overdrawn. Draw the UML diagram for the classes and implement them. Write a test program that creates objects of Account, SavingsAccount, and CheckingAccount and invokes their toString() methods. UML Diagram
UML Diagram

/*
* Class for Account
*/
import java.util.Date;
public class Account {
private String accountNumber;
private double balance;
private double intrestRate;
private Date dateCreated;
// constructor
public Account(String accountNumber, double balance, double intrestRate) {
this.accountNumber = accountNumber;
this.balance = balance;
this.intrestRate = intrestRate;
this.dateCreated = new Date();
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
// method to deposit amount
public void deposit(double amount){
balance = balance + amount;
System.out.println("Deposited "+amount);
}
// method to withdraw amount
public void withdraw(double amount){
// withdraw only if there is sufficient balance
if(balance>=amount){
balance = balance - amount;
System.out.println("Withdrawed "+amount);
}
else
System.out.println("No enough balance");
}
public String toString() {
return "AccountNumber: " + accountNumber + "\nBalance:" + balance + "\nIntrestRate=" + intrestRate + "\nDateCreated=" + dateCreated+"\n";
}
}
/*
* Class for checking account
*/
public class CheckingAccount extends Account{
private double overDraftLimit;
public CheckingAccount(String accountNumber, double balance, double intrestRate, double odLimit) {
super(accountNumber, balance, intrestRate);
overDraftLimit = odLimit;
}
public String toString() {
return super.toString() + "OverDraftLimit:" + overDraftLimit + "\n";
}
// method to withdraw amount
public void withdraw(double amount){
// withdraw only if there is sufficient balance
if((getBalance()+overDraftLimit)>=amount){
setBalance(getBalance()- amount);
System.out.println("Withdrawed "+amount);
}
else
System.out.println("No enough balance");
}
}
/*
* Class for savings account
*/
public class SavingsAccount extends Account{
public SavingsAccount(String accountNumber, double balance, double intrestRate) {
super(accountNumber, balance, intrestRate);
}
}
/*
* Class to test
*/
public class TestProgram {
public static void main(String[] args) {
//create objects
Account a = new Account("12345", 100, 5);
CheckingAccount c = new CheckingAccount("34534", 100, 3, 100);
SavingsAccount s = new SavingsAccount("67687", 100, 5);
// do operations and display account details
a.withdraw(100);
a.deposit(100);
System.out.println(a.toString());
c.withdraw(200);
c.deposit(500);
System.out.println(c.toString());
s.withdraw(200);
s.deposit(500);
System.out.println(s.toString());
}
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

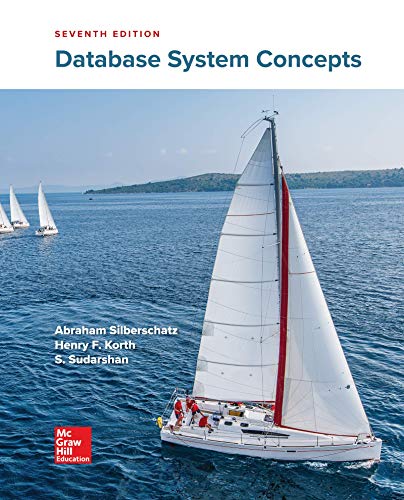
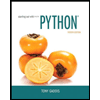
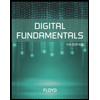
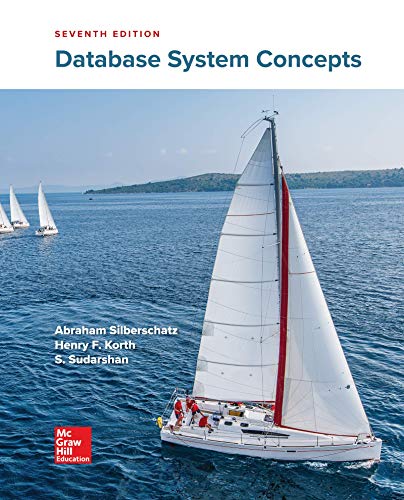
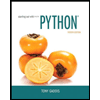
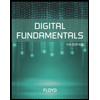
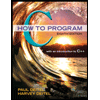
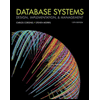
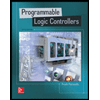