Start with the code below and complete the getInt method. The method should prompt the user to enter an integer. Scan the input the user types. If the input is not an int, throw an IOException; otherwise, return the int.
Start with the code below and complete the getInt method. The method should prompt the user to enter an integer. Scan the input the user types. If the input is not an int, throw an IOException; otherwise, return the int.
In the main
import java.util.*;
import java.io.*;
public class ReadInteger{
public static void main() {
// your code goes here
}
public static int getInt() throws IOException {
// your code goes here
}
}

import java.util.*;
import java.io.*;
public class ReadInteger {
public static void main(String[] args) {
// prompt user to enter integer
System.out.print("Enter integer : ");
// invoke the getInt method
try {
// using try catch block in main program
int i = getInt();
} catch (IOException e) {
// print exception message
System.out.println(e.getMessage());
}
}
public static int getInt() throws IOException {
// using scanner object
Scanner scanner = new Scanner(System.in);
// get input
String inputEntered = scanner.nextLine();
try {
return Integer.parseInt( inputEntered );
} catch ( Exception e ) {
// throw exception
throw new IOException( "IOException: The input is not integer");
}
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

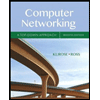
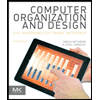
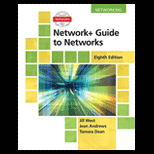
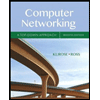
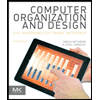
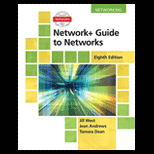
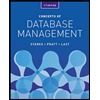
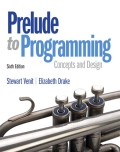
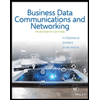