Write an application that computes and displays the day on which you become(or became) 10,000 days old. For example, if you we're born on January 1st, 2000, the output would be I will be 10000 days old on 2027-05-19. It is giving me errors in the picture shown in here. here is my code: import java.time.*; import java.util.Scanner; public class TenThousandDaysOld { public static void main(String[] args) { Scanner in = new Scanner(System.in); int month; int day; int year; int daysOld = 10000; System.out.println("Enter month"); month = in.nextInt(); System.out.println("Enter day"); day = in.nextInt(); System.out.println("Enter year"); year = in.nextInt(); LocalDate birthDate = LocalDate.of(year, month, day); LocalDate futureDate = birthDate.plusDays(daysOld); System.out.println("I will be " + daysOld + " old on " + futureDate); } }
Write an application that computes and displays the day on which you become(or became) 10,000 days old. For example, if you we're born on January 1st, 2000, the output would be I will be 10000 days old on 2027-05-19. It is giving me errors in the picture shown in here. here is my code: import java.time.*; import java.util.Scanner; public class TenThousandDaysOld { public static void main(String[] args) { Scanner in = new Scanner(System.in); int month; int day; int year; int daysOld = 10000; System.out.println("Enter month"); month = in.nextInt(); System.out.println("Enter day"); day = in.nextInt(); System.out.println("Enter year"); year = in.nextInt(); LocalDate birthDate = LocalDate.of(year, month, day); LocalDate futureDate = birthDate.plusDays(daysOld); System.out.println("I will be " + daysOld + " old on " + futureDate); } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
this assignment wants me to:
Write an application that computes and displays the day on which you become(or became) 10,000 days old. For example, if you we're born on January 1st, 2000, the output would be I will be 10000 days old on 2027-05-19.
It is giving me errors in the picture shown in here.
here is my code:
import java.time.*;
import java.util.Scanner;
public class TenThousandDaysOld {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int month;
int day;
int year;
int daysOld = 10000;
System.out.println("Enter month");
month = in.nextInt();
System.out.println("Enter day");
day = in.nextInt();
System.out.println("Enter year");
year = in.nextInt();
LocalDate birthDate = LocalDate.of(year, month, day);
LocalDate futureDate = birthDate.plusDays(daysOld);
System.out.println("I will be " + daysOld + " old on " + futureDate);
}
}

Transcribed Image Text:### Programming Exercise 4-9: Calculate Date of 10,000 Days Old
#### Task Overview
In this exercise, you'll write a program that computes the date on which a person will be 10,000 days old. The implementation uses Java programming language with user input for the month, day, and year of birth.
#### Code Explanation
- **Imports**: The program begins by importing necessary Java packages (`java.time.*` and `java.util.Scanner`).
- **Main Class**: The class `TenThousandDaysOld` has a `main` method where the calculation logic is implemented.
- **Scanner Initialization**: A `Scanner` object is used to gather input from the user.
- **Variables**:
- `int month`, `day`, `year` to store user-provided birth date.
- `int daysOld` set to `10000` to calculate the milestone date.
- **User Input**:
- Prompts the user to enter their birth month, day, and year using `nextInt()`.
#### Terminal Output
- The user is prompted to enter:
- Month: `11`
- Day: `10`
- Year: `2000`
The calculated output displayed:
- `"I will be 10000 days old on 2028-03-28"`
#### Program Results
- Displays the actual and expected outputs.
- **Result Section**:
- Shows a mismatch in output with `Expected Output` indicating date format issue (`\d{4}-\d{2}-\d{2}`).
#### Checks and Feedback
- **Requirements**: The program needs to declare a variable of type `LocalDate` using `LocalDate.of()` function.
- **Passing Criteria**: String literals were not processed correctly, and corrections are needed.
#### Submission and Testing
- Submit the program after writing and click on "Run Checks" for validation.
- The current status indicates "0 out of 1 checks passed."
Make sure to address the output formatting and variable declaration to align with expected standards.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
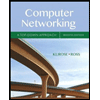
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
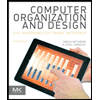
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
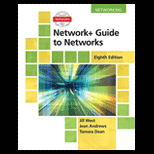
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
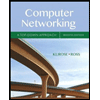
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
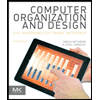
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
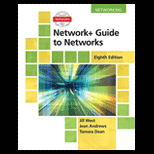
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
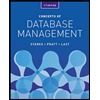
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
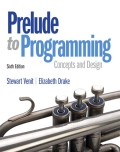
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
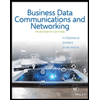
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY